如何在 C 语言中获取子字符串
Satishkumar Bharadwaj
2023年10月12日
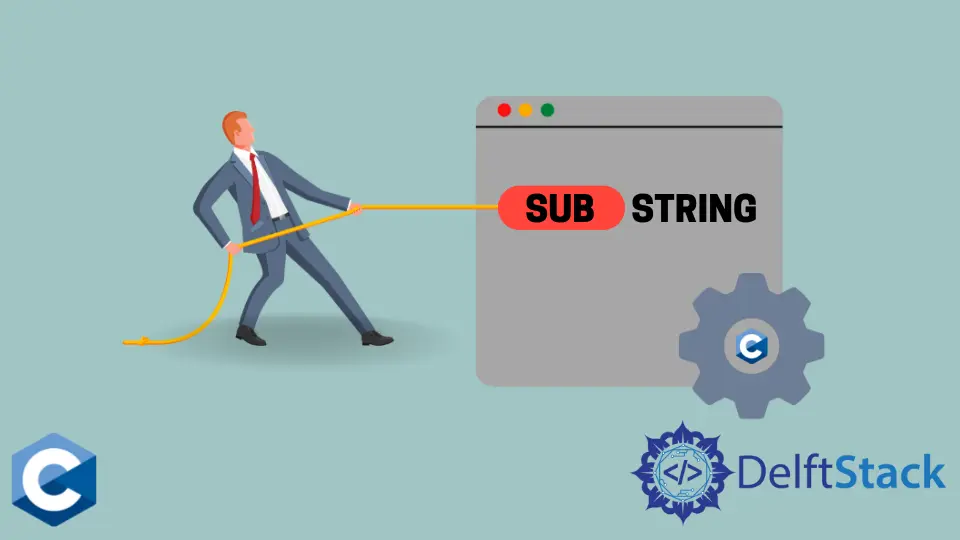
本教程介绍了如何在 C 语言中从字符串中获取子字符串,从字符中获取子字符串的方法有 memcpy()
和 strncpy()
等。
memcpy()
函数在 C 语言中获取子字符串
memcpy()
函数将源文件中的字符数复制到目标文件的内存区。这个函数在 <string.h>
头文件中可用。
当源地址和目标地址重叠时,这个函数会产生问题。这个函数不检查地址是否为空,也不检查是否发生溢出。
memcpy()
函数返回一个指向目标字符串的指针。没有返回值来显示错误。
memcpy()
的语法
void *memcpy(void *destination_string, const void *source_string,
size_t number);
destination_string
是指向目标字符串的指针。source_string
是指向原始字符类型值数组的指针。number
是字符数。
#include <stdio.h>
#include <string.h>
int main(void) {
char *text = "The test string is here";
char subtext[7];
memcpy(subtext, &text[9], 6);
subtext[6] = '\0';
printf("The original string is: %s\n", text);
printf("Substring is: %s", subtext);
return 0;
}
输出:
The original string is: The test string is here
Substring is: string
strncpy()
函数在 C 语言中获取子字符串
strncpy()
函数与 strcpy()
函数相同。唯一不同的是,strncpy()
函数将给定数量的字符从源字符串复制到目标字符串。strncpy()
函数在 <string.h>
头文件中可用。
该函数在复制源字符串后返回一个指向目标字符串的指针。
strncpy()
的语法
void *strncpy(void *destination_string, const void *source_string,
size_t number);
destination_string
是指向目标字符串的指针。source_string
是指向原始字符值数组的指针。number
是字符数。
如果 strncpy()
函数在 source_string
中遇到一个空字符,该函数将在 destination_string
中添加空字符。
#include <stdio.h>
#include <string.h>
int main(void) {
char *text = "The test string is here";
char subtext[7];
strncpy(subtext, &text[9], 6);
subtext[6] = '\0';
printf("The original string is: %s\n", text);
printf("Substring is: %s", subtext);
return 0;
}
输出:
The original string is: The test string is here
Substring is: string