How to Use the getchar Function in C
-
Use the
getchar
Function to Read a Single Character From Standard Input Stream in C -
Use the
getchar
Function to Read String Input in C
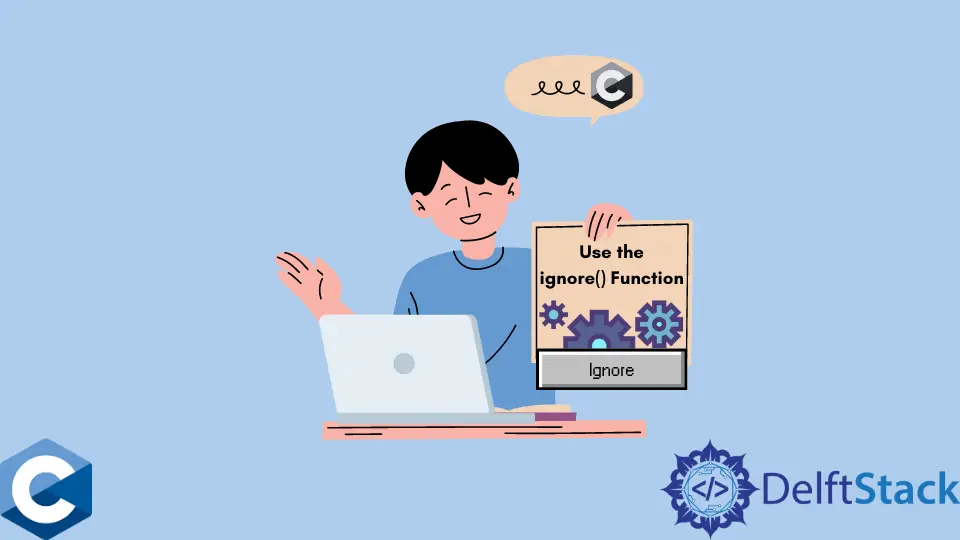
This article will demonstrate multiple methods about how to use the getchar
function in C.
Use the getchar
Function to Read a Single Character From Standard Input Stream in C
The getchar
function is part of standard input/output utilities included in the C library. There are multiple functions for character input/output operations like fgetc
, getc
, fputc
or putchar
. fgetc
and getc
basically have equivalent features; they take file stream pointer to read a character and return it as an unsigned char
cast to an int
type.
Note that getchar
is the specific case of getc
that implicitly passes the stdin
file stream as an argument to read characters from. Thus, getchar
takes no arguments and returns the read character cast to the int
type. In the following example, we demonstrate the basic scenario of inputting a single character and printing it with the putchar
function.
#include <stdio.h>
#include <stdlib.h>
int main(void) {
int ch;
printf("Please, input a single character: ");
ch = getchar();
putchar(ch);
exit(EXIT_SUCCESS);
}
Use the getchar
Function to Read String Input in C
Alternatively, we can implement a loop to read the string input until the new line or EOF
is encountered, and store it in a preallocated char
buffer. Mind though, that this method adds performance overhead compared to calls to gets
or getline
, which are library functions implementing the same feature. The main part of the solution is a while
loop that executes until the returned value from the getchar
function is not equal to the new line character or EOF
.
In this case, we arbitrarily allocated a char
array of size - 20 characters. Each iteration, the pointer to the first element of the array is implemented, and the return value from getchar
is assigned to it. Finally, we output the buffer with the printf
function call.
#include <stdio.h>
#include <stdlib.h>
enum { SIZE = 20 };
int main(void) {
char buf[SIZE];
char *p;
int ch;
p = buf;
printf("Please, provide input: ");
while ((ch = getchar()) != '\n' && ch != EOF) {
*p++ = (char)ch;
}
*p++ = 0;
if (ch == EOF) {
printf("EOF encountered\n");
}
printf("%s\n", buf);
exit(EXIT_SUCCESS);
}
Output:
Please, provide input: string longer than 20 characters
string longer than 20 characters
Even though the previous example code might usually work just fine, it has several errors that can result in buffer overflow bugs or abnormal program termination. Since we process the user input until the new line or EOF
is encountered, there are no guarantees it will fit in the fixed-size char
buffer. If we have to use a fixed buffer, we are responsible for keeping a count of the input size and stopping storing it in the buffer after the capacity is reached.
After we solved this issue in the previous code, we have to deal with the printf
statement that uses %s
specifier to print the buffer contents. Note that there is no guarantee that the last character of the input string is a null byte, so the printf
call would not know where to stop if the user herself does not insert the null byte at the end of the buffer. The following sample code corrects the previous errors and adds some lines for better demonstration.
#include <stdio.h>
#include <stdlib.h>
enum { SIZE = 20 };
int main(void) {
char buf[SIZE];
int ch;
size_t index = 0;
size_t chars_read = 0;
printf("Please, provide input: ");
while ((ch = getchar()) != '\n' && ch != EOF) {
if (index < sizeof(buf) - 1) {
buf[index++] = (char)ch;
}
chars_read++;
}
buf[index] = '\0';
if (ch == EOF) {
printf("EOF encountered\n");
}
if (chars_read > index) {
printf("Truncation occured!\n");
}
printf("%s\n", buf);
exit(EXIT_SUCCESS);
}
Output:
Please, provide input: string longer than 20 characters
Truncation occured!
string longer than
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook