How to Compare Char in C
- Compare Char in C Using Comparison Operators
-
Compare Char in C Using the
strcmp()
Function - Conclusion
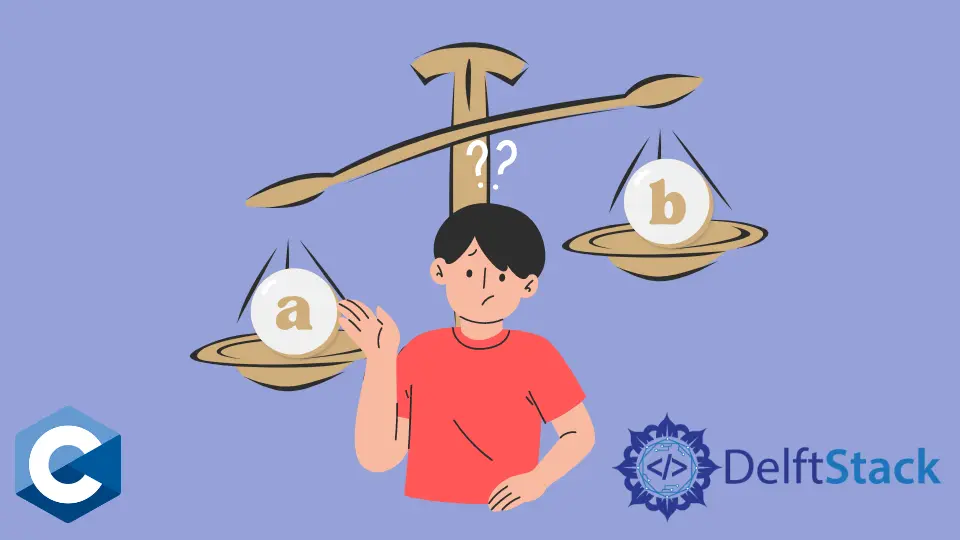
In C, a char
variable is associated with its own ASCII value. This enables us to compare characters by assessing their ASCII values through relational operators.
This article explores two primary methods for comparing characters in C: using comparison operators and the strcmp()
function.
Compare Char in C Using Comparison Operators
In C, a char
variable has its own ASCII value, so we can compare characters by comparing their ASCII values using relational operators. The common relational operators used for character comparison include:
<
(less than): Compares whether one character’s ASCII value is less than another character’s ASCII value.>
(greater than): Compares whether one character’s ASCII value is greater than another character’s ASCII value.==
(equal to): Compares whether one character’s ASCII value is equal to another character’s ASCII value.
Here is an example:
#include <stdio.h>
int main(void) {
char firstCharValue = 'm';
char secondCharValue = 'n';
if (firstCharValue < secondCharValue)
printf("%c is smaller than %c.", firstCharValue, secondCharValue);
if (firstCharValue > secondCharValue)
printf("%c is greater than %c.", firstCharValue, secondCharValue);
if (firstCharValue == secondCharValue)
printf("%c is equal to %c.", firstCharValue, secondCharValue);
return 0;
}
Output:
m is smaller than n.
This program compares the values 'm'
and 'n'
based on their ASCII values:
'm'
has an ASCII value of109
.'n'
has an ASCII value of110
.
Since 'm'
(109
) is smaller than 'n'
(110
), the first if
statement will be true, and it will print m is smaller than n
.
The other two if
statements will be false because 'm'
is not greater than 'n'
, and they are not equal.
Compare Char in C Using the strcmp()
Function
The strcmp()
function, found in the string
header file, is employed to perform a character-by-character comparison of two strings.
When the initial characters of both strings are identical, the comparison proceeds to the next character in each string. This process continues until either differing characters are encountered or a null character '\0'
is encountered in either of the two strings.
Below is the basic syntax of the strcmp()
function:
int strcmp(const char* firstStringValue, const char* secondStringValue);
Here, firstStringValue
and secondStringValue
are the two strings we want to compare.
The function returns an integer value:
- If two strings are equal or identical, it returns
0
. - If the ASCII value of the first unmatched character is greater than the second, it returns a positive integer value.
- If the ASCII value of the first unmatched character is less than the second, it returns a negative integer value.
The complete program to compare two strings is shown below:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(void) {
char firstString[] = "b";
char secondString[] = "b";
char thirdString[] = "B";
int result;
result = strcmp(firstString, secondString);
printf("strcmp(firstString, secondString) = %d\n", result);
result = strcmp(firstString, thirdString);
printf("strcmp(firstString, thirdString) = %d\n", result);
return 0;
}
Output:
strcmp(firstString, secondString) = 0
strcmp(firstString, thirdString) = 32
In the code above, strcmp(firstString, secondString)
compares two identical strings, both containing the character 'b'
. Therefore, it returns 0
, indicating that the two strings are equal.
On the other hand, strcmp(firstString, thirdString)
compares 'b'
with 'B'
. In ASCII character encoding, 'b'
has a smaller value (98
) than 'B'
(66
), so strcmp()
returns a positive integer (32
in this case) to indicate that the first string is greater than the second string in lexicographic order.
Conclusion
In this article, we have discussed the essential techniques for comparing char
variables in C, offering insights into when to use comparison operators and the strcmp()
function. Understanding these methods can help C programmers to efficiently evaluate char
variables based on their ASCII values or compare entire strings, making it possible to implement diverse and powerful algorithms in their applications.