How to Get Length of Char Array in C
-
How to Get the Length of a Char Array in C Using the
sizeof
Operator -
How to Get the Length of a Char Array in C Using the
strlen
Function - How to Get the Length of a Char Array in C by Manual Counting
- How to Get the Length of a Char Array in C Using Pointers
- Conclusion
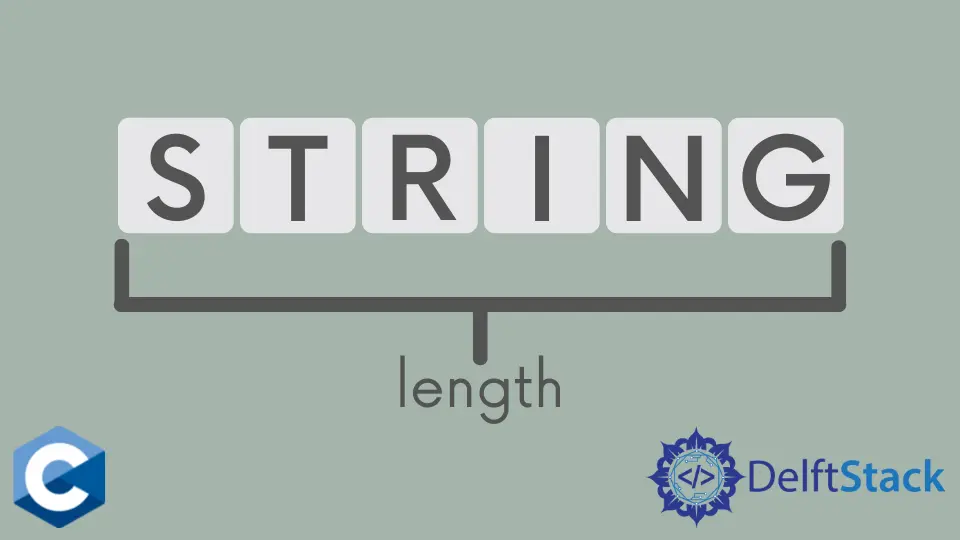
In C programming, character arrays, often representing strings, are prevalent in various applications ranging from text processing to file I/O. Knowing the length of a character array is crucial for proper manipulation and traversal.
In this article, we’ll explore different methods and techniques to accurately determine the length of a character array in C.
How to Get the Length of a Char Array in C Using the sizeof
Operator
One straightforward approach to determine the length of a character array in C is by utilizing the sizeof
operator.
The sizeof
operator in C is a compile-time unary operator that returns the size of its operand in bytes. It is commonly used to determine the size of variables, arrays, and data types.
When applied to an array, sizeof
provides the total size occupied by the array in memory, including all its elements.
The syntax of the sizeof
operator in C is straightforward:
sizeof(array)
Here, array
is the name of the array whose size we want to determine. The sizeof
operator evaluates the size of the array at compile-time and returns the result as a size_t
value, representing the number of bytes occupied by the array.
When applied to a character array (char[]
), the sizeof
operator calculates the size of the array in bytes. Since each element of a character array occupies one byte, the size of the array returned by sizeof
corresponds to the number of characters in the array.
Dividing the total size of the array by the size of an individual element yields the number of elements present in the array, thus providing its length.
Now, let’s consider an example that demonstrates how to use the sizeof
operator to get the length of a character array:
#include <stdio.h>
int main() {
char arr[] = {'a', 'b', 'c', 'd', 'e', 'f', 'g', '\0'};
char arr2[] = "array initialized";
int length1 = sizeof(arr) / sizeof(arr[0]) -
1; // subtract 1 to exclude the null terminator
int length2 = sizeof(arr2) / sizeof(arr2[0]) - 1;
printf("Length of arr: %d\n", length1);
printf("Length of arr2: %d\n", length2);
return 0;
}
Firstly, we initialize two character arrays named arr
and arr2
. These arrays are populated with elements using different initialization methods.
arr
is initialized with individual characters enclosed in curly braces, while arr2
is initialized with a string literal.
Moving forward, we calculate the length of arr
and arr2
using the sizeof
operator. This operator provides us with the total size occupied by the arrays in memory.
int length1 = sizeof(arr) / sizeof(arr[0]) - 1;
int length2 = sizeof(arr2) / sizeof(arr2[0]) - 1;
By dividing this total size by the size of an individual element and subtracting 1 to exclude the null terminator, we obtain the number of elements present in the array, thereby determining its length. This process is performed for both arr
and arr2
, resulting in variables length1
and length2
holding their respective lengths.
After computing the lengths, we proceed to display them using printf
statements. We print the lengths of arr
and arr2
to the console, enabling us to verify the correctness of our calculations.
Output:
Length of arr: 7
Length of arr2: 17
In the output, arr
contains 7 elements, while arr2
holds 17 elements, including the null terminator automatically added when initialized with a string literal. This illustrates the effectiveness of using the sizeof
operator to accurately determine the length of a char
array in C.
If dealing with null-terminated strings, remember that the length obtained using sizeof
includes the terminating null character ('\0'
). Adjustments may be necessary depending on the context.
Also, when working with multidimensional character arrays, sizeof
will return the total size of the array in bytes, not the number of elements. Therefore, division by sizeof(array[0])
might not yield the expected result.
How to Get the Length of a Char Array in C Using the strlen
Function
Determining the length of a character array (char
array), particularly when dealing with null-terminated strings, can be efficiently achieved using the strlen
function.
The strlen
function is part of the C standard library and is specifically designed to determine the length of null-terminated strings. It iterates through the characters of a string until it encounters the null terminator ('\0'
), signifying the end of the string.
By counting the number of characters before the null terminator, strlen
effectively computes the length of the string.
The syntax of the strlen
function is straightforward:
#include <string.h>
size_t strlen(const char *str);
Here, const char *str
is a pointer to the null-terminated character array (string) whose length we want to determine. The strlen
function returns the number of characters in the string, excluding the null terminator.
Here’s how we can use the strlen
function to determine the length of a character array:
#include <stdio.h>
#include <string.h>
int main() {
char arr[] = {'a', 'b', 'c', 'd', 'e', 'f', 'g', '\0'};
char arr2[] = "array initialized";
size_t length1 = strlen(arr);
size_t length2 = strlen(arr2);
printf("Length of arr: %zu\n", length1);
printf("Length of arr2: %zu\n", length2);
return 0;
}
Here, we first initialize two character arrays named arr
and arr2
using different initialization methods. arr
is initialized with individual characters enclosed in curly braces, while arr2
is initialized with a string literal.
Next, we utilize the strlen
function to compute the lengths of arr
and arr2
. By passing each array to strlen
, the function iterates through the characters until it encounters the null terminator ('\0'
), marking the end of the string.
size_t length1 = strlen(arr);
size_t length2 = strlen(arr2);
The strlen
function counts the characters preceding the null terminator, effectively determining the length of the string.
After computing the lengths using strlen
, we store the results in variables named length1
and length2
.
Finally, we display the lengths of both arrays using printf
statements. This allows us to verify the correctness of our calculations and observe the lengths of arr
and arr2
as reported by the strlen
function.
Output:
Length of arr: 7
Length of arr2: 17
The output confirms that the strlen
function accurately determined the lengths of arr
and arr2
.
The length of arr
is reported as 7, corresponding to the number of elements in the array. Similarly, the length of arr2
is depicted as 17, indicating the total number of characters in the string literal "array initialized"
, including the null terminator.
It’s important to note that strlen
expects the input string to be null-terminated. Failure to adhere to this requirement may lead to unpredictable behavior or even runtime errors.
While strlen
is efficient for determining the length of strings, it involves traversing the entire string, which could impact performance for very large strings.
How to Get the Length of a Char Array in C by Manual Counting
In C programming, understanding the underlying mechanisms of fundamental operations is crucial for mastering the language. While functions like sizeof
and strlen
offer convenient ways to determine the length of character arrays, manually counting the elements provides a deeper insight into the workings of arrays and pointers.
Manually counting the elements of an array involves iterating through each element until a termination condition is met. For character arrays, the termination condition typically encounters the null character ('\0'
), which marks the end of the string.
By counting the number of characters before the null character, we effectively determine the length of the array.
To do this, we can use a loop to iterate through the elements of the array until the null terminator is encountered. Here’s the syntax:
size_t length = 0;
while (array[length] != '\0') {
length++;
}
Where:
array
: The character array whose length is to be determined.length
: Counter variable to store the length of the array.
Here’s an example code that demonstrates how to get the length of a character array by manual counting:
#include <stdio.h>
int main() {
char arr[] = {'a', 'b', 'c', 'd', 'e', 'f', 'g'};
int length = 0;
while (arr[length] != '\0') {
length++;
}
printf("Length of arr: %d\n", length);
return 0;
}
In the code example above, we begin by initializing the arr
array with the characters 'a'
to 'g'
. We also declare a variable length
of type int
to store the length of the array.
Subsequently, we employ a while
loop to iterate through each element of the array until we encounter the null terminator ('\0'
). Inside the loop, for each iteration, we increment the length
variable, effectively counting the number of characters in the array.
Once the loop terminates, we have successfully determined the length of the array. Finally, we use the printf
function to display the length of arr
to the console, formatted using the %d
specifier for the size_t
type.
Output:
Length of arr: 7
The output confirms the successful determination of array length using the manual counting method. The length of arr
is depicted as 7, representing the total number of characters, including the null terminator.
Note that manual counting requires careful attention to detail to avoid off-by-one errors or infinite loops. While educational, manual counting may not be as efficient as using built-in functions like strlen
for practical applications.
How to Get the Length of a Char Array in C Using Pointers
Another method to determine the length of a character array (char
array) involves leveraging pointers. This approach offers a dynamic and efficient way to calculate the length of an array.
Pointers are variables that store memory addresses. They enable dynamic memory allocation, efficient array traversal, and intricate data manipulation.
In the context of character arrays, pointers offer a versatile approach to accessing and navigating through array elements.
To obtain the length of a character array using pointers, we iterate through the array elements until we encounter the null terminator ('\0'
). The null terminator marks the end of a string in C, allowing us to determine the array’s length dynamically.
Here’s how it’s done:
#include <stdio.h>
int main() {
char array[] = "array initialized";
char *ptr = array;
int length = 0;
while (*ptr != '\0') {
length++;
ptr++;
}
printf("Length of the array: %d\n", length);
return 0;
}
After initializing the array
with the string literal array initialized
, we then declare a pointer variable ptr
and set it to point to the first element of the array
. Additionally, an integer variable length
is declared and initialized to 0
, which will be used to store the length of the array.
Next, we enter a while
loop where we iterate through the elements of the array using pointers. The loop continues until we encounter the null terminator ('\0'
), which marks the end of the string.
Within each iteration, we increment the length
variable to count the number of characters in the array and advance the ptr
pointer to the next element.
Once the loop terminates, we have successfully determined the length of the array. Finally, we use the printf
function to display the length of the array
to the console.
Output:
Length of the array: 17
The output confirms that using pointers effectively determined the length of the array, which contains 17 characters, including the null terminator.
Conclusion
In conclusion, obtaining the length of a character array in C is a fundamental task with various methods and approaches available. Whether you choose to utilize built-in functions like sizeof
and strlen
, opt for manual counting, or leverage pointers for dynamic traversal, each technique offers its own set of advantages and considerations.
Using the sizeof
operator provides a straightforward and efficient way to determine the length of an array at compile-time, suitable for static arrays. On the other hand, the strlen
function offers a convenient solution for null-terminated strings, automatically handling the termination condition internally.
Manual counting and pointer manipulation provide a deeper understanding of array traversal and memory management in C. These techniques offer flexibility and efficiency, particularly for dynamic arrays or scenarios where built-in functions may not be applicable.
Regardless of the method chosen, it’s essential to consider factors such as null termination, memory safety, and efficiency. By mastering various techniques for calculating the length of character arrays, you’ll be better equipped to handle string manipulation, memory management, and array traversal in your C programs effectively.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook