size_t in C
- What is size_t?
- Using size_t in C Programming
- Benefits of Using size_t
- Common Mistakes When Using size_t
- Conclusion
- FAQ
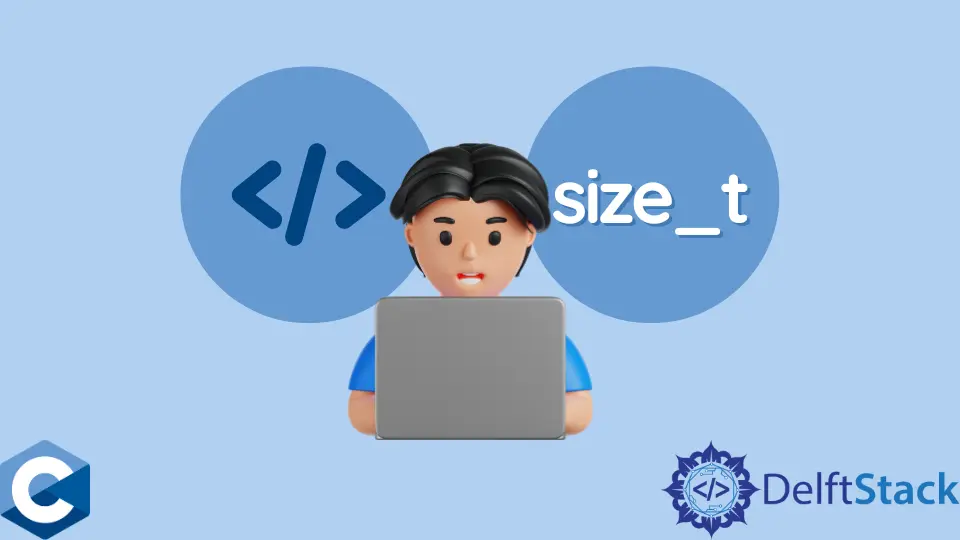
When delving into the world of C programming, one of the most essential yet often overlooked data types is size_t
. This unsigned data type is pivotal for various operations, especially when it comes to handling memory sizes and array indexing. If you’ve ever found yourself wrestling with array sizes or memory allocation, understanding size_t
can significantly ease your programming experience.
In this article, we will explore what size_t
is, why it’s important, and how to effectively use it in your C programs. Whether you’re a beginner or an experienced programmer, grasping this concept will enhance your coding skills and improve your understanding of data types in C.
What is size_t?
size_t
is an unsigned integer type defined in the C standard library, specifically within the <stddef.h>
header file. Its primary purpose is to represent the size of objects in bytes, making it indispensable for memory allocation and manipulation. Since size_t
is unsigned, it can hold larger values than its signed counterparts, which is particularly useful when dealing with large arrays or memory blocks.
Typically, size_t
is used in functions like malloc()
and sizeof()
, which are fundamental for dynamic memory management. By using size_t
, you ensure that your code can handle large data sizes without running into negative values, which can lead to undefined behavior or runtime errors.
In essence, size_t
is a safe and efficient way to handle sizes and counts in C programming, ensuring that your applications run smoothly and efficiently.
Using size_t in C Programming
To effectively use size_t
, you can declare variables of this type to store sizes, counts, or indices. Here’s a simple example illustrating how to use size_t
in a C program:
#include <stdio.h>
#include <stdlib.h>
int main() {
size_t n = 10;
int *arr = malloc(n * sizeof(int));
if (arr == NULL) {
fprintf(stderr, "Memory allocation failed\n");
return 1;
}
for (size_t i = 0; i < n; i++) {
arr[i] = i * 2;
}
for (size_t i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
free(arr);
return 0;
}
Output:
0 2 4 6 8 10 12 14 16 18
In this example, we first declare a variable n
of type size_t
to represent the number of elements we want to allocate memory for. We then use malloc()
to allocate memory for an array of integers. By using size_t
for the loop index and the size of the array, we ensure that our code is safe from negative indices. After populating the array with values, we print them out and finally free the allocated memory. This demonstrates how size_t
can be seamlessly integrated into your code for better memory management.
Benefits of Using size_t
Using size_t
offers several advantages in C programming. Firstly, it enhances portability across different platforms. Since size_t
is defined based on the architecture of the machine, it adjusts accordingly, ensuring that your code behaves consistently on various systems.
Secondly, size_t
prevents errors related to negative values. As an unsigned type, it can only hold non-negative integers, which is crucial when dealing with sizes and counts. This feature helps to avoid common pitfalls that arise from using signed integers, such as underflows or incorrect calculations.
Moreover, size_t
is widely used in standard library functions, making it a familiar type for C programmers. By adhering to this convention, your code will be more readable and maintainable, as other developers will immediately recognize the purpose of your variables.
In conclusion, using size_t
not only makes your code safer and more robust but also aligns with best practices in C programming.
Common Mistakes When Using size_t
Despite its advantages, developers often make mistakes when using size_t
. One common error is mixing size_t
with signed integer types. This can lead to unexpected results, especially when performing arithmetic operations. For instance, subtracting a larger size_t
value from a smaller one can result in a wrap-around, leading to a very large value instead of a negative one.
Another mistake is not considering the size of the data type when using size_t
. While size_t
provides a large range, it is still limited by the architecture (32-bit or 64-bit). Developers should always be aware of this limitation, especially when working with large data sets.
Lastly, failing to check the return value of functions like malloc()
can lead to memory leaks or crashes. Always ensure that your memory allocation is successful before proceeding with your code logic.
By being aware of these common pitfalls, you can use size_t
effectively and avoid potential issues in your C programs.
Conclusion
In summary, size_t
is an invaluable data type in C programming, particularly when handling memory sizes and array indices. Its unsigned nature provides safety against negative values, while its portability ensures consistency across different platforms. By integrating size_t
into your code, you can enhance both the safety and readability of your programs. As you continue your journey in C programming, remember the importance of using the right data types, and let size_t
be your go-to for managing sizes and counts effectively.
FAQ
-
What is size_t in C?
size_t is an unsigned data type in C used to represent the size of objects in bytes. -
Why should I use size_t instead of int?
size_t is unsigned and can hold larger values than int, making it safer for memory sizes and array indexing.
-
Where is size_t defined?
size_t is defined in the C standard library, specifically in the <stddef.h> header file. -
Can size_t be negative?
No, size_t is an unsigned type, so it cannot hold negative values. -
How do I declare a size_t variable?
You can declare a size_t variable like this: size_t myVariable;