Null Terminated Strings in C
- What Are Null Terminated Strings?
- Creating Null Terminated Strings
- String Manipulation Functions
- Common Pitfalls with Null Terminated Strings
- Conclusion
- FAQ
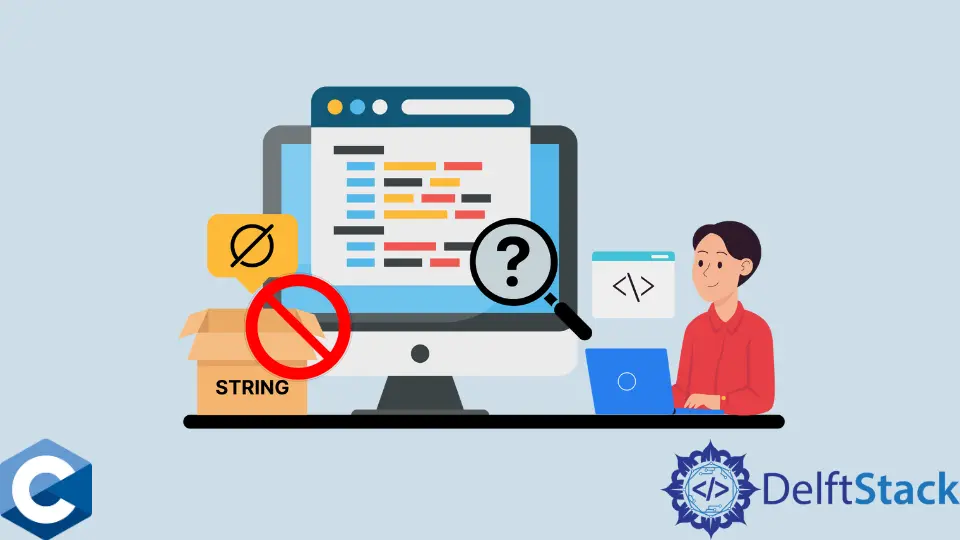
Understanding null-terminated strings is crucial for anyone diving into the C programming language. These strings, which end with a special character known as the null character (\0
), play a vital role in how data is stored and manipulated in C. Unlike many modern programming languages that handle strings as objects, C requires a more manual approach.
This article serves as a small tutorial on null-terminated strings, explaining their structure, how to work with them, and why they are essential in C programming. Whether you are a beginner or brushing up on your C knowledge, this guide will provide valuable insights into managing strings effectively.
What Are Null Terminated Strings?
In C, a string is essentially an array of characters. However, unlike other languages, C does not have a built-in string data type. Instead, it uses arrays of characters, and to define the end of a string, C uses a null character (\0
). This character signals the termination of the string, allowing functions to determine where the string ends.
For example, if you have a string like “Hello”, it is stored in memory as an array of characters followed by a null character, like this: H
, e
, l
, l
, o
, \0
. This method of string representation is efficient in terms of memory usage, but it requires programmers to be cautious, as failing to include the null character can lead to undefined behavior.
Creating Null Terminated Strings
Creating a null-terminated string in C is straightforward. You can declare a character array and initialize it with a string literal. Here’s how you can do it:
#include <stdio.h>
int main() {
char str[] = "Hello, World!";
printf("%s\n", str);
return 0;
}
Output:
Hello, World!
In this example, the string “Hello, World!” is stored in the character array str
. The compiler automatically adds the null character at the end. When we use printf
with the %s
format specifier, it prints the string until it encounters the null character.
The beauty of null-terminated strings lies in their simplicity. They allow you to easily manipulate and traverse strings using standard library functions like strlen
, strcpy
, and strcat
. However, it’s essential to ensure that your strings are properly null-terminated to avoid unexpected behavior.
String Manipulation Functions
C provides a variety of functions for manipulating null-terminated strings. Here are a few common ones:
strlen
: This function calculates the length of a string, excluding the null character.strcpy
: It copies one string to another.strcat
: This function concatenates two strings.
Let’s see how these functions work in practice:
#include <stdio.h>
#include <string.h>
int main() {
char str1[20] = "Hello";
char str2[20] = "World";
printf("Length of str1: %zu\n", strlen(str1));
strcpy(str1, str2);
printf("After copying, str1: %s\n", str1);
strcat(str1, "!");
printf("After concatenation, str1: %s\n", str1);
return 0;
}
Output:
Length of str1: 5
After copying, str1: World
After concatenation, str1: World!
In this code snippet, we first determine the length of str1
using strlen
, which returns 5. Then, we copy str2
into str1
using strcpy
, and finally, we concatenate an exclamation mark to str1
using strcat
. Each function demonstrates the power and flexibility of null-terminated strings in C.
Common Pitfalls with Null Terminated Strings
While working with null-terminated strings can be straightforward, there are several common pitfalls to watch out for:
- Buffer Overflow: If you try to copy a string that is larger than the destination array, you can overwrite memory, leading to undefined behavior.
- Forget to Null-Terminate: If you forget to add the null character, functions that expect a null-terminated string may read past the intended end, causing crashes or incorrect results.
- Pointer Arithmetic: When manipulating strings using pointers, ensure that you do not exceed the bounds of the allocated memory.
To illustrate these pitfalls, consider the following example:
#include <stdio.h>
#include <string.h>
int main() {
char str[5];
strcpy(str, "Hello"); // This will cause a buffer overflow
printf("%s\n", str);
return 0;
}
Output:
Segmentation fault
In this example, the array str
is only large enough to hold 5 characters, including the null terminator. Attempting to copy “Hello” into it causes a buffer overflow, which can lead to a segmentation fault. Always ensure that your arrays are sufficiently sized to hold the strings you intend to store.
Conclusion
Null-terminated strings are a fundamental concept in C programming, providing a simple yet effective way to handle strings. By understanding how they work, you can manipulate text data efficiently and avoid common pitfalls. Whether you’re writing simple programs or complex applications, mastering null-terminated strings will enhance your C programming skills. Remember to always be mindful of memory management and string termination to ensure your programs run smoothly.
FAQ
-
What is a null-terminated string?
A null-terminated string is an array of characters in C that ends with a null character (\0
), indicating where the string terminates. -
How do I create a null-terminated string in C?
You can create a null-terminated string by declaring a character array and initializing it with a string literal. For example,char str[] = "Hello";
. -
What are some common functions for manipulating null-terminated strings?
Common functions includestrlen
for calculating string length,strcpy
for copying strings, andstrcat
for concatenating strings. -
What happens if I forget to null-terminate a string?
If you forget to null-terminate a string, functions that operate on strings may read past the intended end, leading to undefined behavior or crashes.
- How can I avoid buffer overflow when working with strings?
To avoid buffer overflow, always ensure that your destination arrays are large enough to hold the source strings, including the null terminator.