How to Concatenate String and Int in C
-
How to Concatenate String and Int in C Using the
asprintf
,strcat
, andstrcpy
Functions -
How to Concatenate String and Int in C Using the
asprintf
andmemccpy
Functions -
How to Concatenate String and Int in C Using the
snprintf
Function - Conclusion
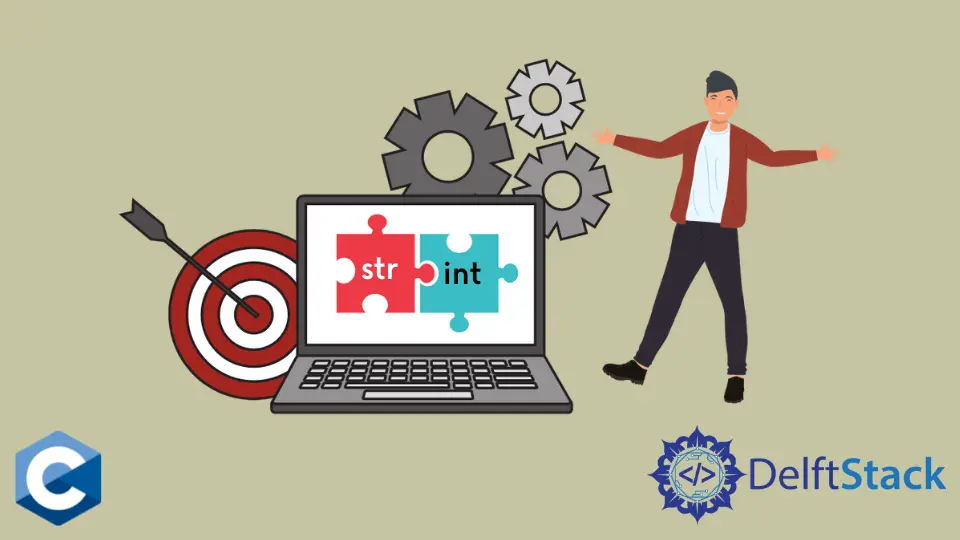
Concatenating strings and integers is a fundamental aspect of C programming, often encountered in various scenarios where textual and numerical data need to seamlessly come together. Achieving this concatenation involves a careful interplay of functions and techniques, ensuring both the accuracy of the result and the efficient use of memory.
In this comprehensive guide, we delve into the intricacies of concatenating strings and integers in the C language, exploring multiple approaches and functions that empower programmers to handle this common task with precision. From the widely-used snprintf
to the dynamic memory allocation of asprintf
and the standard library’s memccpy
, this guide will equip you with the knowledge and skills needed to concatenate strings and integers confidently in your C programming endeavors.
How to Concatenate String and Int in C Using the asprintf
, strcat
, and strcpy
Functions
The initial step in concatenating an integer variable and a character string involves the conversion of the integer to a string. The key tool in achieving this is the asprintf
function.
The asprintf
function is a part of the GNU C library extension, primarily used for dynamically allocating memory and formatting strings. Unlike sprintf
, which requires a pre-allocated destination string buffer, asprintf
dynamically allocates memory using the malloc
function internally.
It returns the length of the formatted string and a pointer to the newly allocated memory. However, it’s important to note that asprintf
may not be available in all C implementations.
Syntax of asprintf
:
int asprintf(char **strp, const char *format, ...);
Where:
strp
: A pointer to a pointer that will be set to the allocated string.format
: A format string similar to those used inprintf
....
: Additional arguments based on the format string.
On the other hand, the strcpy
and strcat
functions are standard string manipulation functions in C. strcpy
is used to copy one string to another, while strcat
concatenates two strings.
It’s essential to ensure that the destination string buffer
has enough space to accommodate the concatenated result.
Syntax of strcpy
:
char *strcpy(char *dest, const char *src);
Where:
dest
: Pointer to the destination array where the content is to be copied.src
: C string to be copied.
Syntax of strcat
:
char *strcat(char *dest, const char *src);
Where:
dest
: Pointer to the destination array, which should contain a C string.src
: C string to be appended.
Let’s delve into a practical example demonstrating the use of these functions for integer and string concatenation.
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#ifndef MAX
#define MAX 100
#endif
int main(int argc, char *argv[]) {
const char *str1 = "hello there";
int n1 = 1234;
char *num;
char buffer[MAX];
// Convert integer to string using asprintf
if (asprintf(&num, "%d", n1) == -1) {
perror("asprintf");
} else {
// String concatenation using strcpy and strcat
strcat(strcpy(buffer, str1), num);
printf("%s\n", buffer);
// Free dynamically allocated memory
free(num);
}
exit(EXIT_SUCCESS);
}
In the above example, we begin by including the necessary header files and define the _GNU_SOURCE
macro, indicating that we are using GNU extensions.
Next, a preprocessor directive sets the value of MAX
to 100
if it has not been defined elsewhere in the code. This macro will later be used to define the size of the character array buffer
.
Moving on to the main
function, we declare a constant character pointer str1
pointing to the string hello there
and an integer n1
initialized with the value 1234
. Two character arrays are then declared: num
, which will be used to store the string representation of n1
, and buffer
, a destination string buffer
for the concatenated string.
The code utilizes the asprintf
function to convert the integer n1
to a string and dynamically allocate memory for it.
The result is stored in the num
pointer. An error check is performed to ensure the success of the allocation, and if unsuccessful, an error message using perror
is printed.
Assuming successful conversion and memory allocation, the code proceeds to concatenate two strings using the strcpy
and strcat
functions.
The strcpy
function copies the string str1
to the beginning of the buffer
, and strcat
then appends the string stored in num
. The concatenated result is stored in the buffer
array.
Finally, the concatenated string in buffer
is printed using printf
, and the dynamically allocated memory for the string representation of n1
is freed using free(num)
to prevent memory leaks.
Output:
Chaining Calls With Additional Strings
The versatility of this approach lies in its extensibility. Chained calls can be followed by further invocations of the strcat
function, allowing the concatenation of two or more strings to the designated character buffer
.
Here’s an extended example:
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#ifndef MAX
#define MAX 100
#endif
int main(int argc, char *argv[]) {
const char *str1 = "hello there";
int n1 = 1234;
char *num;
char buffer[MAX];
if (asprintf(&num, "%d", n1) == -1) {
perror("asprintf");
} else {
// Concatenate strings and append more
strcat(strcpy(buffer, "Hello there, "), num);
strcat(buffer, "! continued");
printf("%s\n", buffer);
// Free dynamically allocated memory
free(num);
}
exit(EXIT_SUCCESS);
}
In this extended example, the initial concatenation process is followed by appending more strings to the given character buffer
. The robustness of the code is maintained by checking the return value of the asprintf
function for successful memory allocation.
Output:
We can also initialize the buffer
as an empty string by assigning an empty string literal (""
) to it. This ensures that it starts with only the NULL
character.
As we can see, the combination of asprintf
, strcat
, and strcpy
functions provides a flexible approach to concatenate strings and integers in C. Careful memory management, dynamic allocation, and extensibility make this method suitable for various concatenation scenarios.
How to Concatenate String and Int in C Using the asprintf
and memccpy
Functions
Another approach to concatenate a character string and an integer in C involves using asprintf
in conjunction with memccpy
.
The asprintf
function dynamically allocates memory for a formatted string. It is part of the GNU C library extension and is particularly useful for converting integers to strings.
Syntax of asprintf
:
int asprintf(char **strp, const char *format, ...);
Where:
strp
: A pointer to a pointer that will be set to the allocated string.format
: A format string similar to those used in printf....
: Additional arguments based on the format string.
On the other hand, the memccpy
function is part of the C standard library’s string utilities and is found in the <string.h>
header file. It copies bytes from one buffer
to another, stopping when a specified character is encountered.
Syntax of memccpy
:
void *memccpy(void *dest, const void *src, int c, size_t n);
Where:
dest
: Pointer to the destination array where the content is to be copied.src
: Pointer to the source of data to be copied.c
: The character to stop copying at.n
: Maximum number of bytes to copy.
Let’s explore the provided code to understand how these functions work together:
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#ifndef MAX
#define MAX 100
#endif
int main(int argc, char *argv[]) {
const char *str1 = "hello there";
int n1 = 1234;
char *num;
char buffer[MAX];
// Convert integer to string using asprintf
if (asprintf(&num, "%d", n1) == -1) {
perror("asprintf");
} else {
// String concatenation using memccpy
memccpy(memccpy(buffer, str1, '\0', MAX) - 1, num, '\0', MAX);
printf("%s\n", buffer);
// Free dynamically allocated memory
free(num);
}
exit(EXIT_SUCCESS);
}
In the above example, we declare the same constant character pointer str1
pointing to the string hello there
and an integer n1
initialized with the value 1234
.
Two character arrays are then declared: num
, which will be used to store the string representation of n1
, and buffer
, a destination buffer
for the concatenated strings.
The code utilizes the asprintf
function to convert the integer n1
to a string and dynamically allocate memory for it. The result is stored in the num
pointer. An error check is performed to ensure the success of the allocation, and if unsuccessful, an error message using perror
is printed.
Assuming successful conversion and memory allocation, the code proceeds to concatenate strings using the memccpy
function. The memccpy
function is used twice: first to copy the content of the character string str1
to the beginning of the buffer
, and then to append the string stored in num
to the buffer
. The -1
adjustment ensures proper placement in the buffer
.
Finally, the concatenated string in buffer
is printed using printf
, and the dynamically allocated memory for the string representation of n1
is freed using free(num)
to prevent memory leaks.
Output:
This method provides an efficient way to concatenate strings and integers in C, utilizing both dynamic memory allocation and standard library functions. It showcases the flexibility of asprintf
for converting integers to strings and memccpy
for safe concatenation.
Always remember to manage memory appropriately to avoid leaks, as demonstrated by freeing the dynamically allocated memory in the example. This approach is particularly useful when dealing with scenarios where the new string representation of an integer needs to be seamlessly integrated with existing character strings.
How to Concatenate String and Int in C Using the snprintf
Function
Let’s move on to an alternative approach that employs the snprintf
function for concatenating strings and integers in C.
The snprintf
function in the C standard library is designed for safely formatting strings and is particularly useful when concatenating different types of data. It takes a destination string buffer
, the maximum number of characters to be written, a format string, and a variable number of arguments based on the format string.
Syntax of snprintf
:
int snprintf(char *str, size_t size, const char *format, ...);
Where:
str
: A pointer to the destination stringbuffer
where the formatted string will be stored.size
: The maximum number of characters to be written to thebuffer
, including the null-terminator.format
: A format string similar to those used inprintf
....
: Additional arguments based on the format string.
Take a look at the code examples below to see how to use snprintf
to concatenate strings and integers in C.
Example 1: Basic String Concatenation
#include <stdio.h>
int main() {
int n = 42;
char buffer[50];
// C concatenate string and int using snprintf
snprintf(buffer, sizeof(buffer), "The answer is: %d", n);
// Print the result
printf("%s\n", buffer);
return 0;
}
In this code example, we start with the declaration of an integer n
with the value 42
and a character array buffer
with a size of 50. The primary objective is to concatenate a string and an integer.
Using the snprintf
function, we achieve this concatenation by formatting the string The answer is: %d
with the value of the integer n
. The formatted result is then stored in the buffer
while ensuring that the size of the buffer
is not exceeded.
Finally, we print the concatenated string using printf
. The %s
format specifier is used to interpret the buffer
as a string.
Code Output:
Example 2: Concatenation With Existing String
#include <stdio.h>
#include <string.h>
int main() {
const char *str = "Hello, ";
int n = 123;
char buffer[50];
// Concatenate string and int using snprintf
snprintf(buffer, sizeof(buffer), "%s%d", str, n);
// Print the result
printf("%s\n", buffer);
return 0;
}
In this next example, we introduce an existing character string str
(Hello,
) and an integer n
with the value 123
. The objective is to concatenate these two elements and store the result in the character array buffer
.
The snprintf
function is again employed, this time with the format string %s%d
, which combines the existing string with the integer. The resulting string is stored in the buffer
, and we print the final concatenated string using printf
.
This example illustrates the versatility of snprintf
in appending integers to existing strings.
Code Output:
Example 3: Handling Larger Buffers
#include <stdio.h>
int main() {
int x = 9876;
char buffer[100];
// Concatenate string and int using snprintf with a larger buffer
snprintf(buffer, sizeof(buffer), "The value of x is: %d", x);
// Print the result
printf("%s\n", buffer);
return 0;
}
The above example demonstrates how to handle larger buffers
when concatenating a string and an integer. We declare an integer x
with the value 9876
and create a character array buffer
with a size of 100.
Using snprintf
with the format string The value of x is: %d
, we concatenate the string and integer, ensuring that the buffer
size is sufficient. The resulting formatted string is stored in the buffer
, and we print the final concatenated string using printf
.
This example emphasizes the importance of choosing an adequately sized buffer
to accommodate the concatenated string without encountering buffer
overflow issues.
Code Output:
Conclusion
Concatenating strings and integers in C is important for effective string manipulation and versatile programming. Throughout this article, we’ve uncovered several techniques and functions that facilitate this process.
Whether leveraging the dynamic memory allocation of asprintf
and memccpy
, or the safe formatting capabilities of snprintf
, C provides a robust toolkit for handling diverse string concatenation scenarios. These methods not only enable seamless integration of strings and integers but also promote efficient memory management and code clarity.
Whether you’re aiming for basic string concatenation, appending to existing strings, or dealing with larger buffers
, the examples and discussions presented illuminate the paths to achieve these objectives concisely and effectively.
As with any programming task, selecting the right approach depends on the specific requirements of your application. Be mindful of buffer
sizes, handle memory allocations judiciously, and choose the method that aligns with the nature and scale of your concatenation needs.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook