How to Create Formatted Strings in C
-
Create Formatted Strings Using the
sprintf()
Function in C -
Create Formatted Strings Using the
snprintf()
Function in C
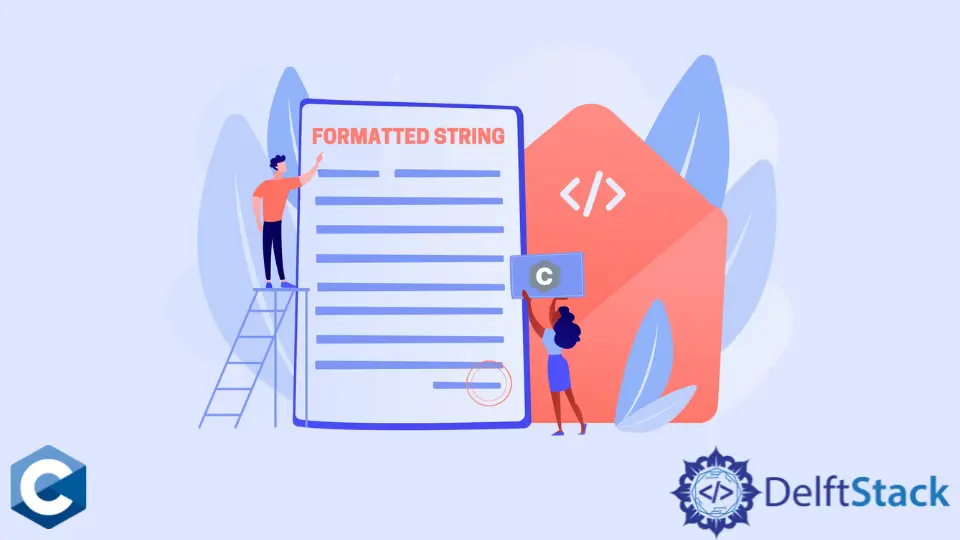
This article discusses how to format a string using C language in such a way as we do in the printf()
function - for example, generating a string by combining string literals and the values of different variables and assigning the result to a string variable for future use.
C language has some built-in library methods that return a formatted string. The two most commonly used functions of them are:
sprintf()
methodsnprintf()
method
We will discuss both of these functions and their examples.
Create Formatted Strings Using the sprintf()
Function in C
The prototype of sprintf()
is as follows:
int sprintf(char* str, const char* format, ...);
The string content will be stored as a C string in the buffer referenced by str
instead of printed if the format was used on printf
. The buffer size should be large enough to hold the complete result string.
Following the content, a null character is automatically inserted.
The function requires at least as many extra arguments as needed for an exact one-to-one correspondence with the format specifiers used in the second argument of this function.
Parameters:
-
char * str
It is a character pointer where the resulting string will be stored. The size of this pointer should be large enough to cater to all the data specified in the format.
-
const char * format
It is also a character pointer containing the string format with all the specifications we specify in the
printf
function. -
...
The function may require a set of additional arguments, each holding a value to replace a format specifier in the format string, depending on the format string (or a pointer to a storage location, for n).
The number of these parameters should, at least, be equal to the number of values indicated in the format specifiers. The function ignores any additional arguments.
Return value:
This function returns an int value specifying the number of characters written in the resultant string. This number of characters does not include the null character automatically appended at the end of the string.
In case of some error or if nothing is written on that string, a negative number is returned.
Example:
#include <stdio.h>
int main() {
char arr[60];
int num, i = 4, j = 2;
num = sprintf(arr, "%d plus %d is %d", i, j, i + j);
printf("[%s] string is %d characters long\n", arr, num);
return 0;
}
The output of the above code is:
[4 plus 2 is 6] string is 13 characters long
In the above code snippet, you can see that we have declared a char array of a fixed size. Then we have used that array in the sprintf
function to save the contents.
For instance, if the format
string in the sprintf
function exceeds the size of the array specified, then a memory error will occur, and the program will be abnormally terminated. A safer version of this function is used to avoid this hassle, the snprintf
function.
Create Formatted Strings Using the snprintf()
Function in C
The prototype of this function is as follows:
int snprintf(char* s, size_t n, const char* format, ...);
The string content is stored as a C string in the buffer pointed by s
instead of being printed if the format was used on printf
(taking n
as the maximum buffer capacity to fill).
If, in this case, the resultant string is of greater size than n-1
, all the extra remaining characters will be discarded for the string but will be counted for the number of characters in the return value.
After the material is written, a null character is automatically appended.
The function requires at least as many extra arguments as are necessary for format after the format parameter.
Parameters:
-
char * s
It is a character pointer for saving the resulting string contents. The size of this array should be at least
n
characters long.
-
size_t n
It is an unsigned integral type telling the maximum number of characters to be written in the buffer. The maximum number of characters that can be written is
n-1
, leaving one character for the null character appended at the end of the string. -
const char * format
It is also a character pointer containing the string format with all the specifications we specify in the
printf
function. -
...
The function may require a set of additional arguments, each holding a value to replace a format specifier in the format string, depending on the format string (or a pointer to a storage location, for n).
The number of these parameters should, at least, be equal to the number of values indicated in the format specifiers. The function ignores any additional arguments.
Return value:
This function returns the number of successfully written characters on the buffer, excluding the terminating null character. In case of any error, it returns a negative number.
Note that if the returned value is greater than 0 and less than the buffer size specified, the string is completely written.
Example:
#include <stdio.h>
int main() {
char arr[100];
int count;
count = snprintf(arr, 100, "The double of %d is %d", 5, 5 * 2);
if (count >= 0 && count < 100) // check returned value
printf("The resultant string is: %s", arr);
else
printf("Not successfully written");
return 0;
}
Output:
The resultant string is: The double of 5 is 10
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn