Scanf String With Spaces in C
-
Use
RegEx
inscanf
to Get User Input With Spaces in C -
Use
%[^\n]s
inscanf
to Get User Input With Spaces in C -
Use
%[^\n]%*c
inscanf
to Get User Input With Spaces in C -
Use
gets()
inscanf
to Get User Input With Spaces in C - Conclusion
- FAQ
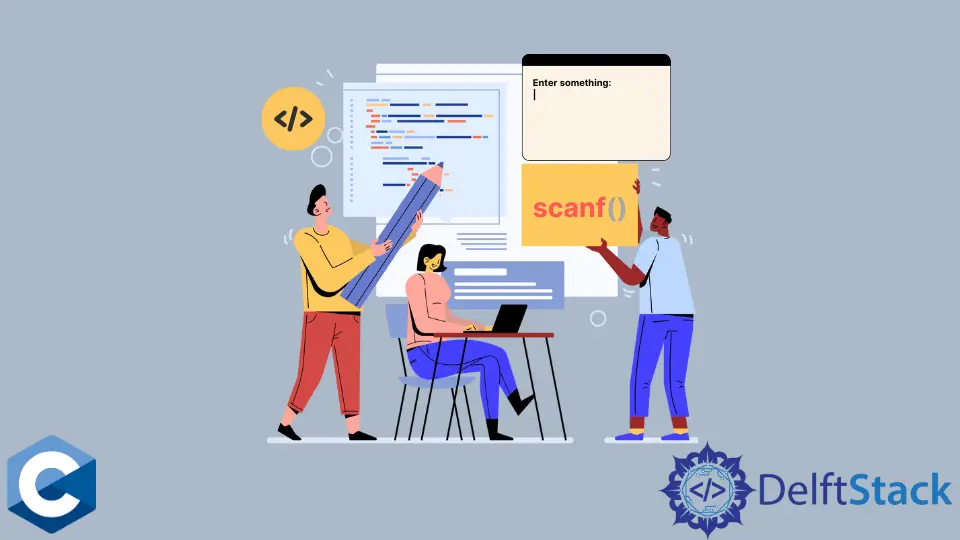
This article will discuss the file descriptor in the C programming language.
When collecting input from the user, scanf()
will, in most cases, ignore things like spaces, backslashes, tabs, and so on; however, we can avoid this limitation by using scanset specifiers. The scanset specifiers are denoted by the notation %[]
.
By expressing it in these square brackets, we may write a character or a string, depending on what we want to accomplish.
The C programming language provides several different ways that we may use to get input from the user while keeping the spaces included in it. Let us have a look at some examples to implement it.
Use RegEx
in scanf
to Get User Input With Spaces in C
This %[]
is a scanset specifier, and using 0-9, a-z, a space
and A-Z
signifies that only those characters may be used as input. Nothing else can be used.
The s
indicates the computer accepts a string as its input.
Source Code:
#include <stdio.h>
int main(int argc, char const *argv[]) {
char name[20];
printf("Please enter your full name: ");
scanf("%[0-9a-zA-Z ]s", name);
printf("\nYour name is: %s", name);
return 0;
}
Output:
Please enter your full name: Saad Aslam
Your name is: Saad Aslam
Use %[^\n]s
in scanf
to Get User Input With Spaces in C
The scanset character for this instance is [].
The ^\n
character instructs the operating system to continue reading user input until a new line is found.
In this case, we took advantage of the XOR operator, which returns true unless two letters are identical. After the character has been determined to be a new line \n
, the XOR operator ^
will return false when the string is read.
Therefore, we write it as %[n]s
rather than %s
. Therefore, to get an input line that contains spaces, we may use the following:
scanf("%[n]s", str);
Source Code:
#include <stdio.h>
int main() {
char str[100];
printf("Please enter your full name: ");
scanf("%[^\n]s", str);
printf("\nYour name is: %s", str);
return 0;
}
Output:
Please enter your full name: Saad Aslam
Your name is: Saad Aslam
Use %[^\n]%*c
in scanf
to Get User Input With Spaces in C
In this case, the scanset character is [].
The ^\n
character instructs the operating system to continue taking input until a new line is found.
Then, using the %*c
, it reads the newline character, and the here-used *
indicates that this newline character is excluded from the output.
Source Code:
#include <stdio.h>
int main() {
char str[20];
printf("Please enter something: ");
scanf("%[^\n]%*c", str);
printf("\nYou entered: %s", str);
return 0;
}
Output:
Please enter something: Writer at Delft Stack
You entered: Writer at Delft Stack
Use gets()
in scanf
to Get User Input With Spaces in C
The char *gets(char *str)
function included in the C library will read a line from the standard input (stdin
) and save it in the string referred to by str
. It halts either when the newline character is read or when the end of the file is reached, whichever occurs first, depending on circumstances.
Source Code:
#include <stdio.h>
int main() {
char str[50];
printf("Please enter your name: ");
gets(str);
printf("\nYour name is: %s", str);
return (0);
}
Output:
Please enter your name: Saad Aslam
Your name is: Saad Aslam
Conclusion
Capturing user input in C, especially when dealing with strings that contain spaces, can be straightforward when you know the right methods. While scanf
has its limitations, functions like fgets
and custom input functions provide flexible alternatives. By employing these techniques, you can ensure that your programs handle user input effectively and efficiently. Whether you’re working on a small project or a larger application, mastering string input will enhance your programming skills and improve user experience.
FAQ
-
What is the difference between scanf and fgets?
scanf stops reading input at the first space, while fgets reads an entire line until a newline character. -
Can I use scanf to read strings with spaces?
Yes, you can use scanf with the format specifier %[^n] to read strings with spaces. -
What happens if the input exceeds the buffer size in fgets?
fgets will read only up to the specified buffer size, and the remaining input will be left in the input buffer, which could lead to unexpected behavior in subsequent input operations. -
Is there a risk of buffer overflow with scanf?
Yes, if the input exceeds the allocated size for the string, it can cause a buffer overflow, leading to undefined behavior. -
Can I customize the input method for strings in C?
Yes, you can create your own functions to handle string input as needed, providing greater control and flexibility.
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn