How to Truncate String in C
- Understanding String Truncation in C
- Method 1: Using String Length and Manual Assignment
-
Method 2: Using
strncpy
for Safe Copying - Method 3: Using a Loop for Custom Truncation Logic
- Conclusion
- FAQ
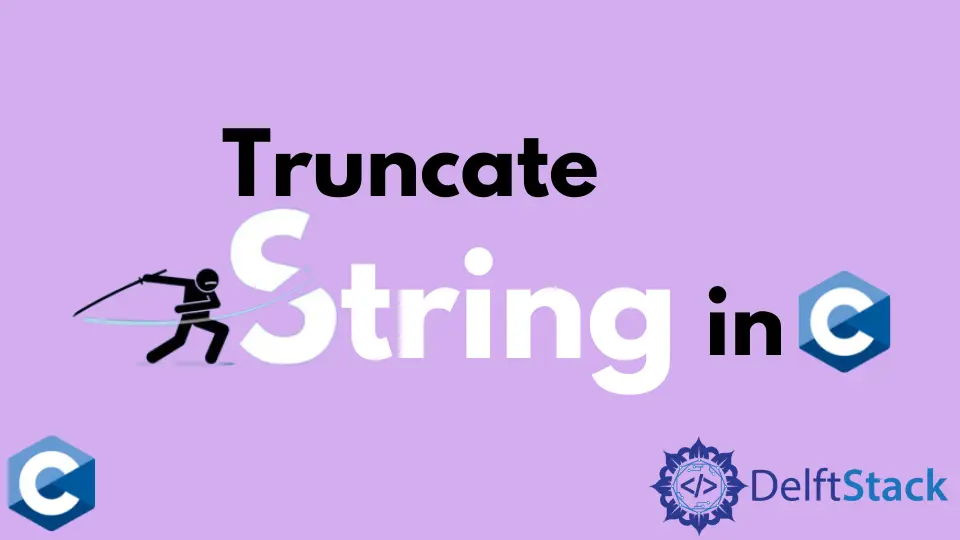
Truncating strings in C can be a common requirement when dealing with text data. Whether you need to limit the size of a string for display purposes or ensure that it fits within a specific buffer, knowing how to effectively truncate strings is essential.
In this article, we will explore various methods to truncate strings in C, providing clear examples and explanations to help you understand the process. By the end, you will have a solid grasp of string manipulation in C, empowering you to handle strings more efficiently in your programming tasks.
Understanding String Truncation in C
Before diving into the methods of truncating strings, it’s important to understand what string truncation means in the context of C programming. A string in C is essentially an array of characters terminated by a null character (\0
). Truncating a string involves shortening this array to a specified length while ensuring that the null terminator remains intact. This is crucial to avoid undefined behavior when working with strings, as C does not manage strings like higher-level languages.
Method 1: Using String Length and Manual Assignment
One of the simplest methods to truncate a string in C is by using the strlen()
function to determine the length of the string, followed by manual assignment to limit the string to a desired length.
#include <stdio.h>
#include <string.h>
void truncateString(char *str, int length) {
if (length < strlen(str)) {
str[length] = '\0';
}
}
int main() {
char str[] = "Hello, World!";
truncateString(str, 5);
printf("%s\n", str);
return 0;
}
Output:
Hello
In this example, we define a function truncateString
that takes a character pointer and an integer length as arguments. It checks if the specified length is less than the actual length of the string. If it is, we assign the null character at the specified index, effectively truncating the string. The main
function demonstrates the truncation of “Hello, World!” to “Hello”. This method is straightforward and effective for simple string truncation tasks.
Method 2: Using strncpy
for Safe Copying
Another efficient way to truncate strings in C is by using the strncpy()
function. This function allows you to copy a specified number of characters from one string to another, making it ideal for truncation.
#include <stdio.h>
#include <string.h>
void truncateString(char *source, char *destination, int length) {
strncpy(destination, source, length);
destination[length] = '\0';
}
int main() {
char source[] = "Hello, World!";
char destination[6]; // +1 for null terminator
truncateString(source, destination, 5);
printf("%s\n", destination);
return 0;
}
Output:
Hello
In this code, we define the truncateString
function, which takes a source string, a destination string, and a length. The strncpy()
function is used to copy the specified number of characters from the source to the destination. After copying, we ensure to add a null terminator at the end of the destination string. This method is particularly useful because it prevents buffer overflow by allowing you to specify the maximum number of characters to copy.
Method 3: Using a Loop for Custom Truncation Logic
For more complex truncation needs, you might want to implement a custom truncation logic using a loop. This method gives you more control over how the truncation is performed.
#include <stdio.h>
void truncateString(char *str, int length) {
int i;
for (i = 0; i < length && str[i] != '\0'; i++) {
// Do nothing, just iterate
}
str[i] = '\0';
}
int main() {
char str[] = "Hello, World!";
truncateString(str, 5);
printf("%s\n", str);
return 0;
}
Output:
Hello
In this example, the truncateString
function uses a loop to iterate through the string until it reaches the desired length or the null terminator. By modifying the string in place, we ensure that the truncation is handled effectively. This method can be particularly useful if you want to implement additional logic during the truncation process.
Conclusion
In conclusion, truncating strings in C is a fundamental skill that can enhance your programming capabilities. Whether you choose to use manual assignment, the strncpy()
function, or a custom loop, understanding these methods will allow you to manipulate strings effectively. By implementing these techniques, you can ensure that your C programs handle string data gracefully and efficiently.
FAQ
-
What is string truncation in C?
String truncation in C refers to the process of shortening a string to a specified length while ensuring that it remains properly terminated with a null character. -
How do I safely copy a string in C?
You can use thestrncpy()
function to safely copy a specified number of characters from one string to another, which helps prevent buffer overflow. -
Can I truncate a string in C without using external libraries?
Yes, you can truncate a string in C using built-in functions likestrlen()
andstrncpy()
, or by implementing your own logic with loops. -
What happens if I forget to add a null terminator after truncating a string?
If you forget to add a null terminator, you may encounter undefined behavior when trying to use the truncated string, as C relies on the null character to determine the end of the string. -
Is there a maximum length for strings in C?
In C, the maximum length of a string is determined by the size of the array that holds the string. However, you should always ensure that your strings are properly terminated to avoid overflow issues.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook