C에서 문자열과 Int 연결
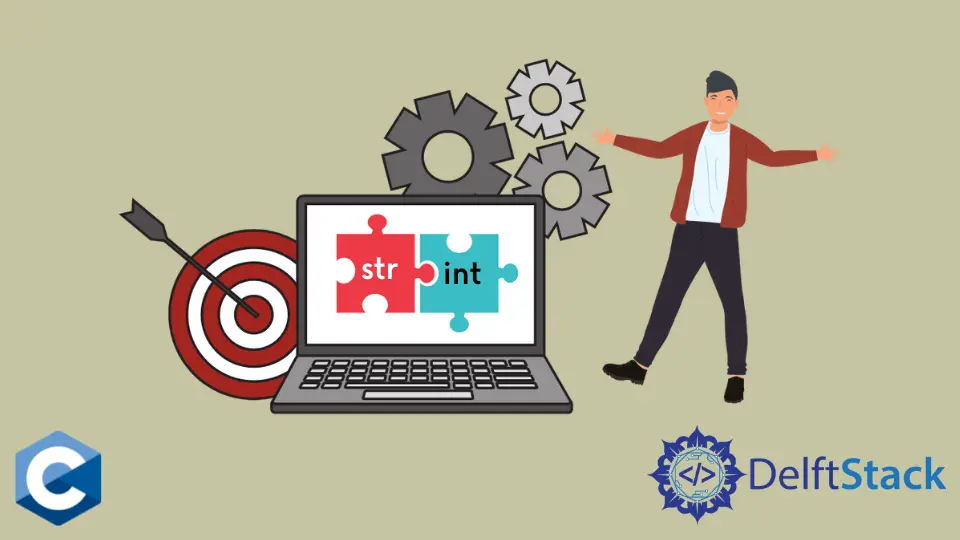
이 기사에서는 C에서string
및int
를 연결하는 여러 방법을 보여줍니다.
asprintf
, strcat
및strcpy
함수를 사용하여 C에서 문자열과 Int 연결
int
변수와 문자열을 연결하는 첫 번째 단계는 정수를 문자열로 변환하는 것입니다. asprintf
함수를 사용하여 전달 된 정수를 문자열로 저장합니다. asprintf
는 GNU C 라이브러리 확장의 일부이며 다른 구현에서는 사용할 수 없습니다. 대상 문자열 버퍼가 내부적으로malloc
함수 호출을 사용하여 동적으로 할당된다는 점을 제외하면sprintf
와 유사하게 작동하며 리턴 된 포인터는 프로그램 종료 전에 해제되어야합니다. 정수가 변환되면strcpy
및strcat
호출을 연결하여 사용자가 할당 한 버퍼에서 두 개의 주어진 문자열을 연결합니다. 이 경우 대상 버퍼의 크기를 나타 내기 위해MAX
매크로를 임의로 정의했지만 실제 시나리오에서는malloc
을 사용하는 것이 더 유연합니다.
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#ifndef MAX
#define MAX 100
#endif
int main(int argc, char *argv[]) {
const char *str1 = "hello there";
int n1 = 1234;
char *num;
char buffer[MAX];
if (asprintf(&num, "%d", n1) == -1) {
perror("asprintf");
} else {
strcat(strcpy(buffer, str1), num);
printf("%s\n", buffer);
free(num);
}
exit(EXIT_SUCCESS);
}
출력:
hello there1234
또는 연결 호출 다음에strcat
함수를 다시 호출하고 지정된char
버퍼에 다른 문자열을 추가 할 수 있습니다. asprintf
함수에서 성공적인 반환 값을 확인하고 실패하면 연결 프로세스를 계속합니다.
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#ifndef MAX
#define MAX 100
#endif
int main(int argc, char *argv[]) {
const char *str1 = "hello there";
int n1 = 1234;
char *num;
char buffer[MAX];
if (asprintf(&num, "%d", n1) == -1) {
perror("asprintf");
} else {
strcat(strcpy(buffer, "Hello there, "), num);
strcat(buffer, "! continued");
printf("%s\n", buffer);
free(num);
}
exit(EXIT_SUCCESS);
}
출력:
Hello there, 1234! continued
asprintf
및memccpy
함수를 사용하여 C에서 문자열과 Int 연결
또는asprintf
를memccpy
와 함께 사용하여 문자열 및int
를 연결할 수 있습니다. memccpy
는<string.h>
헤더 파일에 정의 된 C 표준 라이브러리 문자열 유틸리티의 일부입니다. 소스 및 대상 버퍼를 나타내는 두 개의 포인터가 필요합니다. 이러한 버퍼 메모리 영역은 겹치지 않아야합니다. 그렇지 않으면 결과가 정의되지 않습니다. 마지막 두 인수는 복사를 중지 할 문자와 소스 위치에서 가져올 최대 바이트 수를 나타냅니다. else
범위에서free
함수를 호출합니다. 그렇지 않으면num
포인터가 유효한 포인터인지 확인할 수 없습니다.
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#ifndef MAX
#define MAX 100
#endif
int main(int argc, char *argv[]) {
const char *str1 = "hello there";
int n1 = 1234;
char *num;
char buffer[MAX];
if (asprintf(&num, "%d", n1) == -1) {
perror("asprintf");
} else {
memccpy(memccpy(buffer, str1, '\0', MAX) - 1, num, '\0', MAX);
printf("%s\n", buffer);
free(num);
}
exit(EXIT_SUCCESS);
}
출력:
hello there1234
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook