How to Get the Size of Array in C
- Using the sizeof() Operator
- Limitations of sizeof() with Pointers
- Using a Macro to Get Array Size
- Using a Function to Get Array Size
- Conclusion
- FAQ
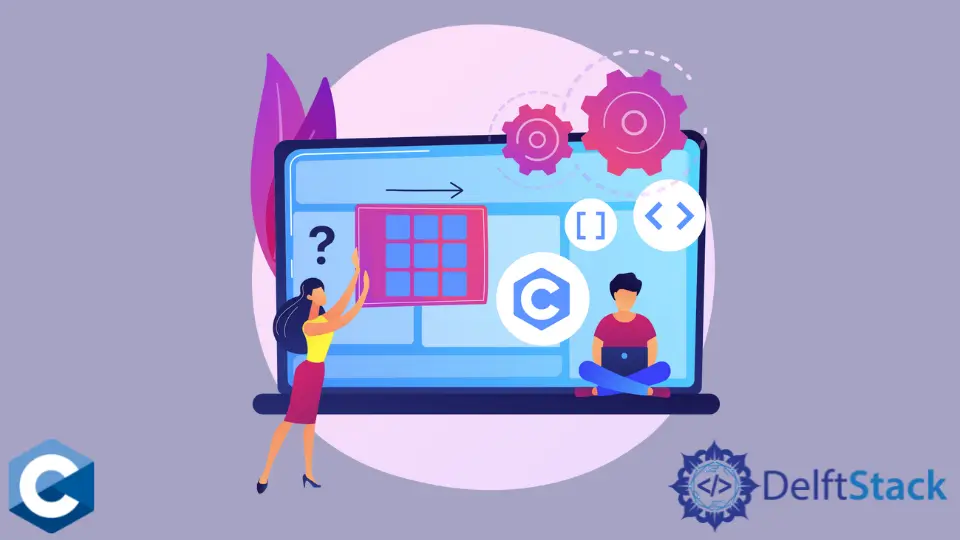
Understanding how to determine the size of an array in C is fundamental for any programmer. Arrays are a core data structure in C, allowing you to store multiple values in a single variable. Knowing the size of an array is crucial for memory management, iteration, and ensuring your program runs smoothly without errors.
In this tutorial, we will explore various methods to find the size of an array, focusing on the powerful sizeof()
operator. By the end, you’ll have a solid grasp of how to effectively manage arrays in your C programs.
Using the sizeof() Operator
One of the most straightforward methods to determine the size of an array in C is by using the sizeof()
operator. This operator returns the total size, in bytes, of a variable or data type. To find the number of elements in an array, you can divide the total size of the array by the size of a single element.
The sizeof()
operator is a compile-time unary operator. It is used to calculate the size of its operand. It returns the size of a variable. The sizeof()
operator gives the size in the unit of byte.
The sizeof()
operator is used for any data type such as primitives like int
, float
, char
, and also non-primitives data type as an array
, struct
. It returns the memory allocated to that data type.
The output may be different on different machines like a 32-bit system can show different output and a 64-bit system can show different of the same data types.
Here’s a simple example to illustrate this:
#include <stdio.h>
int main() {
int arr[10];
size_t size = sizeof(arr) / sizeof(arr[0]);
printf("The size of the array is: %zu\n", size);
return 0;
}
Output:
The size of the array is: 10
In this example, the sizeof(arr)
gives the total size of the array in bytes, while sizeof(arr[0])
gives the size of one element. By dividing these two values, you quickly get the number of elements in the array. This method is reliable and works seamlessly for static arrays defined within the same scope.
Limitations of sizeof() with Pointers
While the sizeof()
operator is a powerful tool, it’s essential to understand its limitations, especially when dealing with pointers. If you pass an array to a function, it decays into a pointer. Consequently, using sizeof()
on the pointer will not yield the expected result.
Let’s look at an example:
#include <stdio.h>
void printArraySize(int arr[]) {
printf("Size of array inside function: %zu\n", sizeof(arr));
}
int main() {
int arr[10];
printArraySize(arr);
return 0;
}
Output:
Size of array inside function: 8
In this case, sizeof(arr)
inside the printArraySize
function returns the size of the pointer, not the array itself. This limitation is crucial to remember when designing functions that work with arrays. To overcome this, you can either pass the size of the array as an additional parameter or use a different data structure.
Using a Macro to Get Array Size
Another efficient method to get the size of an array is to define a macro. This approach encapsulates the logic of calculating the size and makes it reusable throughout your code. Here’s how you can define and use a macro for this purpose:
#include <stdio.h>
#define ARRAY_SIZE(arr) (sizeof(arr) / sizeof((arr)[0]))
int main() {
int arr[10];
printf("The size of the array is: %zu\n", ARRAY_SIZE(arr));
return 0;
}
Output:
The size of the array is: 10
In this example, the ARRAY_SIZE
macro calculates the size of the array whenever it is called. This approach is clean and avoids the pitfalls of pointer decay, as the macro can only be used with arrays defined in the same scope. However, be cautious when using this macro with multi-dimensional arrays, as it may require additional handling.
Using a Function to Get Array Size
If you prefer a function-based approach, you can write a function that accepts an array and its size as parameters. This method is particularly useful when working with dynamic arrays or when you want to maintain clarity in your code.
Here’s an example:
#include <stdio.h>
void printArraySize(int *arr, size_t size) {
printf("Size of array: %zu\n", size);
}
int main() {
int arr[10];
printArraySize(arr, sizeof(arr) / sizeof(arr[0]));
return 0;
}
Output:
Size of array: 10
In this example, the function printArraySize
takes two arguments: a pointer to the array and its size. This method is flexible and allows you to work with arrays of any size, avoiding the pitfalls associated with the sizeof()
operator when dealing with pointers. It’s good practice to pass the size of the array along with the array itself to maintain clarity and prevent errors.
Conclusion
Determining the size of an array in C is a fundamental skill for any programmer. The sizeof()
operator is your go-to tool for static arrays, while understanding the limitations of pointers is crucial for effective programming. Using macros or functions can enhance code readability and reusability. By mastering these techniques, you can manage arrays efficiently and write robust C programs.
FAQ
-
What does the sizeof() operator do in C?
Thesizeof()
operator returns the size, in bytes, of a variable or data type. -
Can I use sizeof() to determine the size of an array passed to a function?
No, when an array is passed to a function, it decays into a pointer, andsizeof()
will return the size of the pointer, not the array. -
What is a common method to avoid pointer decay when working with arrays?
You can pass the size of the array as an additional parameter to the function. -
How can I define a macro to calculate the size of an array?
You can use the macro#define ARRAY_SIZE(arr) (sizeof(arr) / sizeof((arr)[0]))
to calculate the size of an array. -
Is it safe to use sizeof() with multi-dimensional arrays?
Yes, but you need to ensure that the macro or function correctly handles the dimensions of the array.