How to Copy Char Array in C
-
Use the
memcpy
Function to Copy a Char Array in C -
Use the
memmove
Function to Copy a Char Array in C
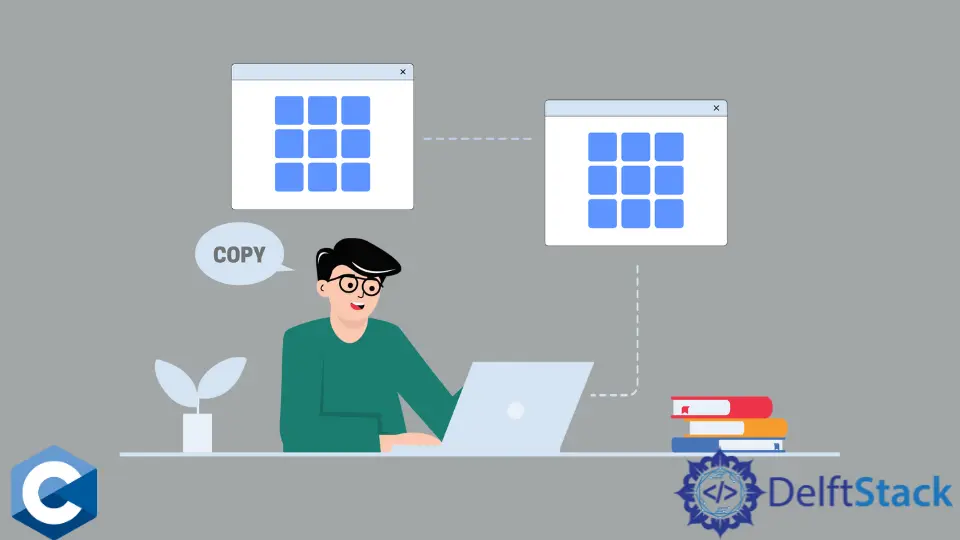
This article will demonstrate multiple methods about how to copy a char array in C.
Use the memcpy
Function to Copy a Char Array in C
char
arrays are probably the most common data structure manipulated in the C code, and copying the array contents is one of the core operations for it. C-style strings are very similar to char
arrays; thus, there are multiple ways to deal with copying the array contents. In the following example, we declared two arrays, arr
and arr2
; the former is initialized with character list notation and the latter with a string literal. Note that this yields different array structures and sizes. The arr
object is 7 characters in the memory, but arr2
takes 17 characters plus terminating null byte resulting in an 18-byte object. Consequently, we pass the value of sizeof arr2 - 1
expression as the second argument to denote the length of the array. On the other hand, one may print the contents of the arr2
array with the printf
function and %s
format specifier.
The same details should be considered when copying the char
arrays to a different location. The memcpy
function is part of the standard library string utilities defined in <string.h>
header file. It takes three parameters, the first of which is the destination pointer, where the contents of the array will be copied. The second parameter is the pointer to the source array, and the last parameter specifies the number of bytes to be copied. Notice that the sizeof
operator returns the char
array object sizes in bytes. So we allocate the dynamic memory with malloc
call passing the sizeof arr
expression value to it. The returned memory region is enough to hold the arr
contents, and we don’t need to worry about buffer overflow bugs. Mind though, that the pointer returned from malloc
should be freed.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void printCharArray(char *arr, size_t len) {
for (size_t i = 0; i < len; ++i) {
printf("%c, ", arr[i]);
}
printf("\n");
}
int main(int argc, char *argv[]) {
char arr[] = {'a', 'b', 'c', 'd', 'e', 'f', 'g'};
char arr2[] = "array initialized";
printf("%lu\n", sizeof arr);
printf("%lu\n", sizeof arr2);
printCharArray(arr, sizeof arr);
printCharArray(arr2, sizeof arr2 - 1);
char *str = malloc(sizeof arr);
memcpy(str, arr, sizeof arr);
printf("str: ");
printCharArray(str, sizeof arr);
free(str);
str = malloc(sizeof arr2);
memcpy(str, arr2, sizeof arr2);
printf("str: %s\n", str);
free(str);
exit(EXIT_SUCCESS);
}
Output:
7
18
a, b, c, d, e, f, g,
a, r, r, a, y, , i, n, i, t, i, a, l, i, z, e, d,
str: a, b, c, d, e, f, g,
str: array initialized
Use the memmove
Function to Copy a Char Array in C
memmove
is another memory area copying function from standard library string utilities. It has been implemented as a more robust function to accommodate the case when destination and source memory regions overlap. memmove
parameters are the same as memcpy
.
When copying the arr2
contents, we passed sizeof arr2
expression as the third parameter. Meaning that even the terminating null byte got copied to the destination pointer, but we consequently utilize this action by calling printf
with %s
to output contents rather than using printCharArray
.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void printCharArray(char *arr, size_t len) {
for (size_t i = 0; i < len; ++i) {
printf("%c, ", arr[i]);
}
printf("\n");
}
int main(int argc, char *argv[]) {
char arr[] = {'a', 'b', 'c', 'd', 'e', 'f', 'g'};
char arr2[] = "array initialized";
char *str = malloc(sizeof arr);
memmove(str, arr, sizeof arr);
printf("str: ");
printCharArray(str, sizeof arr);
free(str);
str = malloc(sizeof arr2);
memmove(str, arr2, sizeof arr2);
printf("str: %s\n", str);
free(str);
exit(EXIT_SUCCESS);
}
Output:
str: a, b, c, d, e, f, g,
str: array initialized
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook