How to Print Char Array in C
- Use a Loop to Print Char Array in C
-
Use
printf
With%s
Specifier to Print Char Array in C -
Use
puts
to Print Char Array in C - Conclusion
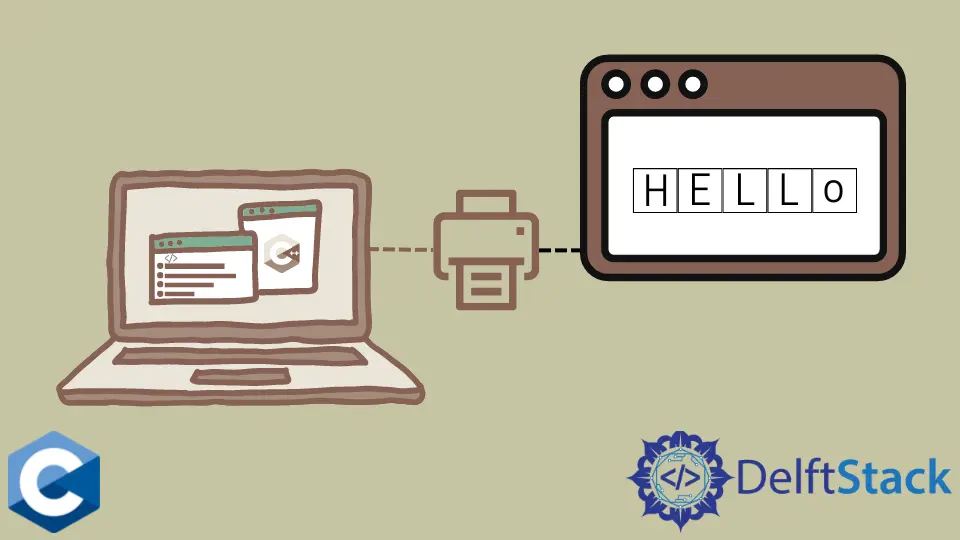
In this article, we will introduce multiple methods on how to print a char array in C.
Use a Loop to Print Char Array in C
Using a loop is a versatile way to print the elements of a character array. It provides control over the printing process and allows for more complex formatting.
However, it’s essential to have a clear understanding of the array’s length to avoid going out of bounds and causing segmentation faults.
Use the for
Loop
The for
loop is an excellent choice when you know the length of the character array in advance. It allows you to iterate precisely the right number of times, ensuring that you access only valid elements.
In the following example, we demonstrate the for
loop method by iterating six times over an array of six characters:
#include <stdio.h>
#include <stdlib.h>
#define STR(num) #num
int main(void) {
char arr1[] = {'a', 'b', 'c', 'd', 'e', 'f'};
printf(STR(arr1) ": ");
for (int i = 0; i < 6; ++i) {
printf("%c, ", arr1[i]);
}
printf("\b\b\n");
exit(EXIT_SUCCESS);
}
Output:
arr1: a, b, c, d, e, f
Here are the steps involved in the code above:
-
We include the necessary header files for input/output operations (
stdio.h
) and the standard library (stdlib.h
) as they are required for functions likeprintf
andexit
. -
We define the character array
arr1
, containing six characters:a
,b
,c
,d
,e
, andf
. -
We use the
#define
preprocessor directive to create a string representation of the array’s name. This step isn’t required for printing the array but can be useful for descriptive output. -
Inside the
main
function, we print the name of the array (in this case,arr1
) using theprintf
function. -
We then employ a
for
loop to iterate fromi = 0
toi = 5
(inclusive), ensuring that we access each element of the array. The loop counteri
is used to access the individual characters in the array. -
Within the loop, we use
printf
to print the character at the current index, followed by a comma and a space. This formatting separates each character with a comma. -
After the loop completes, we use
printf
again to erase the last comma and space by printing backspace characters (\b\b
), which results in a cleaner and well-formatted output. -
Finally, we call
exit(EXIT_SUCCESS)
to indicate that the program has executed successfully. A return value of0
(represented byEXIT_SUCCESS
) is a conventional way to signal a successful program execution.
In this example, the for
loop method allows us to precisely control the number of iterations over the array, ensuring that we print each character without going out of bounds.
Use the while
Loop
The while
loop method is a more flexible approach when you may not know the array’s length in advance, but you need to ensure the loop stops at the correct point to prevent segmentation faults. Here’s an example:
#include <stdio.h>
int main(void) {
char arr2[] = {'a', 'b', 'c', 'd', 'e', 'f'};
int i = 0;
printf("arr2: ");
while (arr2[i] != '\0') {
putchar(arr2[i]);
i++;
}
printf("\n");
return 0;
}
Output:
arr2: abcdef
Here’s the process involved in the code above:
-
Include the necessary header file for input/output operations (
stdio.h
) to use functions likeprintf
andputchar
. -
Define the character array
arr2
with the charactersa
,b
,c
,d
,e
, andf
. -
Initialize an integer variable
i
to0
. This variable serves as an index to traverse the character array. -
Print the name of the array (
arr2
) using theprintf
function. -
Use a
while
loop to iterate through the character arrayarr2
. The loop continues until it encounters the null character'\0'
, which indicates the end of the string. -
Inside the loop, use
putchar
to print the character at the current index (arr2[i]
). This function prints a single character to the standard output. -
After printing the character, increment the loop counter
i
to move to the next character in the array. -
Finally, return
0
to indicate successful execution of the program.
Use printf
With %s
Specifier to Print Char Array in C
The printf
function in C is a versatile tool for formatting and outputting data to the console. It can manipulate input variables using type specifiers and format them accordingly.
When it comes to printing character arrays, the %s
specifier in printf
plays a crucial role.
A character array in C is essentially a sequence of characters stored in memory. While it shares a structure similar to C-style strings, character arrays don’t always end with the null character '\0'
.
In contrast, C-style strings terminate with a '\0'
to signify the string’s end. When using printf
to print character arrays, including the null byte ('\0'
) at the end of the array is crucial for proper output.
If you omit the null termination, printf
may attempt to access memory regions beyond the array’s boundaries, leading to undefined behavior, crashes, or segmentation errors. Therefore, it’s essential to ensure that your character array is null-terminated for safe and predictable behavior when using %s
with printf
.
#include <stdio.h>
#include <stdlib.h>
#define STR(num) #num
int main(void) {
char arr1[] = {'a', 'b', 'c', 'd', 'e', 'f'};
char arr2[] = {'t', 'r', 'n', 'm', 'b', 'v', '\0'};
printf("%s\n", arr1);
printf("%s\n", arr2);
exit(EXIT_SUCCESS);
}
Output:
abcdeftrnmbv
trnmbv
As you can see, when we print the arr1
that doesn’t have the null terminator, we will get more characters until the iteration reaches one null terminator, \0
.
Here’s another example:
#include <stdio.h>
#include <stdlib.h>
int main(void) {
char arr[] = {'H', 'e', 'l', 'l', 'o', '\0'};
printf("%s\n", arr);
exit(EXIT_SUCCESS);
}
Output:
Hello
In this example, we’ve defined a character array arr
that represents the string Hello
. The null character '\0'
is included at the end to ensure proper termination.
As we can see, it printed the entire string Hello
.
Provide Length Information With %s
In some cases, you might want to specify the length of the character array to be printed, even if it contains null termination. The %.*s
specifier allows you to do just that by dynamically specifying the length during printing.
You can either hard-code the length as a number between the %
and s
, or you can use an asterisk *
to take the length from a provided argument. Note that both methods include the .
character before the number or asterisk in the specifier.
#include <stdio.h>
#include <stdlib.h>
int main(void) {
char arr[] = {'H', 'e', 'l', 'l', 'o', '\0'};
printf("%.3s\n", arr); // Prints the first 3 characters: "Hel"
printf("%.*s\n", 4, arr); // Prints the first 4 characters: "Hell"
exit(EXIT_SUCCESS);
}
Output:
Hel
Hello
In the first printf
statement, we use %.3s
to print only the first three characters of the array arr
. In the second printf
, we use %.*s
to specify the length as 4, printing the first four characters.
This flexibility can be useful when you need to extract substrings or print a portion of the character array.
Use puts
to Print Char Array in C
While the printf
function is a versatile tool for formatted output, the puts
function offers a straightforward way to print strings and character arrays in C. What sets puts
apart from other output functions like printf
is its automatic appending of a newline character ('\n'
) to the end of the printed string.
This behavior is particularly useful when you want to print strings as separate lines, as it ensures proper formatting.
The puts
function takes a character array, often representing a string, as its argument. This character array must be null-terminated, meaning it ends with a null character '\0'
to denote the string’s conclusion.
puts(string);
When puts
is called, it begins reading characters from the start of the character array and continues until it encounters the null character '\0'
. During this process, each character is printed to the standard output.
After successfully printing all the characters from the character array, puts
automatically appends a newline character '\n'
to the output. This newline character ensures that the next output appears on a new line, making it suitable for printing strings as separate lines.
The puts
function returns a non-negative integer if it succeeds, and it returns EOF
(End of File) in case of an error.
Here’s an example of how to use puts
to print a character array in C:
#include <stdio.h>
int main() {
char str[] = "Hello, World!";
char arr[] = {'H', 'e', 'l', 'l', 'o', '\0'};
puts(str);
puts(arr);
return 0;
}
In this example, the character array str
contains the string Hello, World!
, and the character array arr
contains {'H', 'e', 'l', 'l', 'o', '\0'}
. The puts
function is used to print str
and arr
.
When you compile and run this program, you will see the following output:
Hello, World!
Hello
The newline character is automatically added by puts
, making the output well-formatted and easy to read.
Conclusion
In this article, we explored several methods for printing character arrays in C. We discussed using loops, the %s
specifier with printf
, and the puts
function.
These methods provide diverse approaches to print character arrays effectively. When choosing a method, consider factors like null termination and formatting requirements to ensure successful character array printing in C.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook