How to Dynamically Allocate an Array in C
- Understanding Dynamic Memory Allocation
- Using malloc to Allocate a Dynamic Array
-
Using
calloc()
for Dynamic Array Initialization - Resizing an Array with realloc
- Conclusion
- FAQ
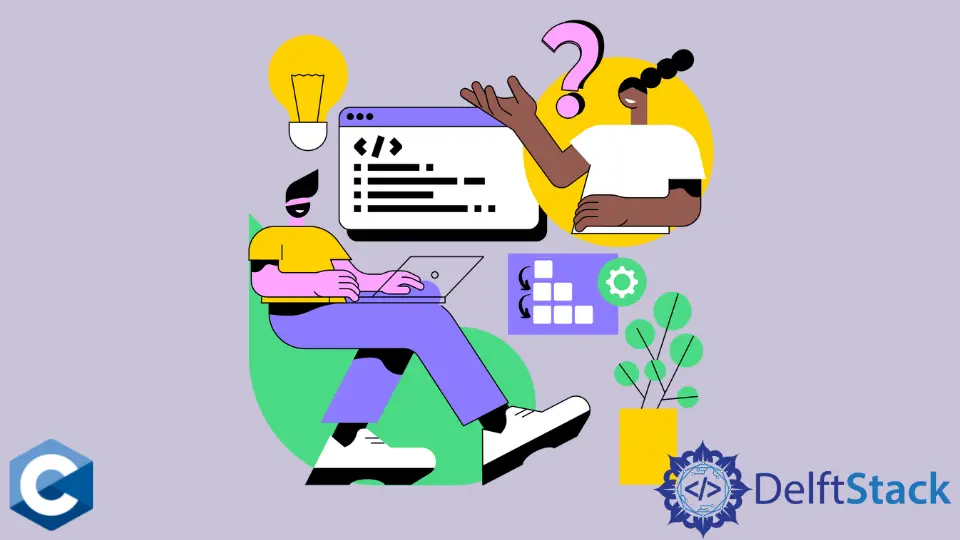
Dynamic memory allocation is a powerful feature in C that allows you to allocate memory at runtime. This is particularly useful for handling arrays when the size is not known at compile time.
In this article, we will explore how to dynamically allocate an array in C, discussing the methods and providing code examples to illustrate the process. By the end of this article, you’ll have a solid understanding of dynamic array allocation in C, which can enhance your programming skills significantly. Let’s dive into the world of dynamic memory management!
Understanding Dynamic Memory Allocation
Dynamic memory allocation in C is achieved through the use of functions such as malloc()
, calloc()
, realloc()
, and free()
. These functions are part of the C standard library and allow you to allocate memory as needed during the execution of your program.
malloc(size_t size)
: Allocates a specified number of bytes and returns a pointer to the allocated memory.calloc(size_t num, size_t size)
: Allocates memory for an array of elements, initializes all bytes to zero, and returns a pointer to the memory.realloc(void *ptr, size_t size)
: Resizes the memory block pointed to byptr
to a new size.free(void *ptr)
: Deallocates the memory previously allocated.
Using these functions, you can create arrays that can grow or shrink based on your program’s needs. Below, we will explore how to use these functions to allocate arrays dynamically.
Using malloc to Allocate a Dynamic Array
The malloc()
function is the most commonly used method for dynamic memory allocation. It allocates a specified number of bytes and returns a pointer to the first byte of the allocated memory. Here’s how you can use it to create a dynamic array:
#include <stdio.h>
#include <stdlib.h>
int main() {
int n;
printf("Enter the number of elements: ");
scanf("%d", &n);
int *array = (int *)malloc(n * sizeof(int));
if (array == NULL) {
printf("Memory allocation failed\n");
return 1;
}
for (int i = 0; i < n; i++) {
array[i] = i + 1;
}
printf("Dynamic array elements are: ");
for (int i = 0; i < n; i++) {
printf("%d ", array[i]);
}
printf("\n");
free(array);
return 0;
}
Output:
Enter the number of elements: 5
Dynamic array elements are: 1 2 3 4 5
In this code, we first prompt the user to enter the number of elements they want in the array. We then use malloc()
to allocate memory for n
integers. It’s crucial to check if the allocation was successful by verifying if the returned pointer is NULL
. After populating the array with values, we print them out. Finally, we free the allocated memory to prevent memory leaks.
Using calloc()
for Dynamic Array Initialization
The calloc()
function is another way to dynamically allocate memory, but it initializes all allocated bytes to zero. This can be particularly useful when you want to ensure that your array elements start with a default value. Here’s how to use calloc()
for dynamic array allocation:
#include <stdio.h>
#include <stdlib.h>
int main() {
int n;
printf("Enter the number of elements: ");
scanf("%d", &n);
int *array = (int *)calloc(n, sizeof(int));
if (array == NULL) {
printf("Memory allocation failed\n");
return 1;
}
for (int i = 0; i < n; i++) {
array[i] = i + 1;
}
printf("Dynamic array elements are: ");
for (int i = 0; i < n; i++) {
printf("%d ", array[i]);
}
printf("\n");
free(array);
return 0;
}
Output:
Enter the number of elements: 5
Dynamic array elements are: 1 2 3 4 5
In this example, we use calloc()
to allocate memory for n
integers. The key difference here is that calloc()
initializes all elements to zero. This can save you from having to explicitly set the values to zero later. After populating and displaying the array, we free the allocated memory just like before.
Resizing an Array with realloc
Sometimes, you may need to resize an already allocated array. The realloc()
function allows you to change the size of a previously allocated memory block. Here’s how you can use realloc()
to expand or shrink a dynamic array:
#include <stdio.h>
#include <stdlib.h>
int main() {
int n;
printf("Enter the initial number of elements: ");
scanf("%d", &n);
int *array = (int *)malloc(n * sizeof(int));
if (array == NULL) {
printf("Memory allocation failed\n");
return 1;
}
for (int i = 0; i < n; i++) {
array[i] = i + 1;
}
printf("Dynamic array elements are: ");
for (int i = 0; i < n; i++) {
printf("%d ", array[i]);
}
printf("\n");
printf("Enter the new size: ");
int newSize;
scanf("%d", &newSize);
array = (int *)realloc(array, newSize * sizeof(int));
if (array == NULL) {
printf("Memory reallocation failed\n");
return 1;
}
for (int i = n; i < newSize; i++) {
array[i] = i + 1;
}
printf("Resized dynamic array elements are: ");
for (int i = 0; i < newSize; i++) {
printf("%d ", array[i]);
}
printf("\n");
free(array);
return 0;
}
Output:
Enter the initial number of elements: 3
Dynamic array elements are: 1 2 3
Enter the new size: 5
Resized dynamic array elements are: 1 2 3 4 5
In this code, we first allocate an initial array size and populate it. After displaying the initial elements, we ask the user for a new size. The realloc()
function is then used to resize the array. If the reallocation is successful, we populate the new elements and display the entire array. Just as in previous examples, we ensure to free the allocated memory at the end.
Conclusion
Dynamically allocating arrays in C is an essential skill for any programmer. It provides flexibility in memory management, allowing you to create arrays of varying sizes during runtime. We explored three primary methods of dynamic memory allocation: using malloc()
, calloc()
, and realloc()
. Each method has its unique advantages, making them suitable for different scenarios. By mastering these techniques, you can write more efficient and adaptable C programs. Happy coding!
FAQ
-
What is dynamic memory allocation in C?
Dynamic memory allocation in C refers to the process of allocating memory storage during the execution of a program, allowing for flexible data structures like arrays. -
How do I check if memory allocation was successful in C?
You can check if memory allocation was successful by verifying if the pointer returned bymalloc()
,calloc()
, orrealloc()
isNULL
. -
What happens if I forget to free dynamically allocated memory?
Forgetting to free dynamically allocated memory can lead to memory leaks, which can exhaust system memory over time and cause your program to crash. -
Can I change the size of a dynamically allocated array?
Yes, you can change the size of a dynamically allocated array using therealloc()
function. -
What is the difference between
malloc()
andcalloc()
?
malloc()
allocates memory without initializing it, whilecalloc()
allocates memory and initializes all bytes to zero.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook