Array of Strings in C
- Use 2D Array Notation to Declare Array of Strings in C
-
Use the
char*
Array Notation to Declare Array of Strings in C
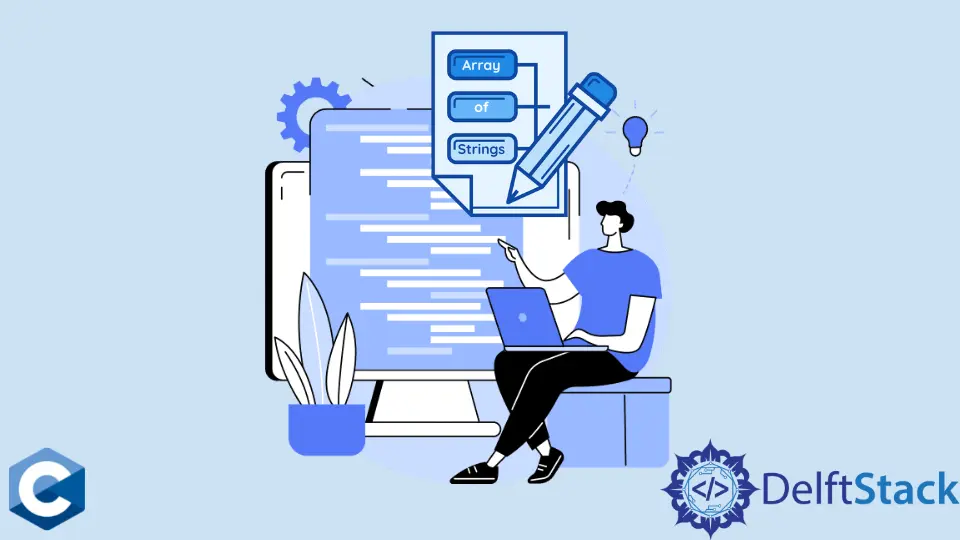
This article will demonstrate multiple methods about how to declare an array of strings in C.
Use 2D Array Notation to Declare Array of Strings in C
Strings in C are simply a sequence of chars
stored in a contiguous memory region. One distinction about character strings is that there is a terminating null byte \0
stored at the end of the sequence, denoting one string’s end. If we declare a fixed array of char
using the []
notation, then we can store a string consisting of the same number of characters in that location.
Note that one extra character space for the terminating null byte should be considered when copying the character string to the array location. Thus, one can declare a two-dimensional char
array with brackets notation and utilize it as the array of strings.
The second dimension of the array will limit the maximum length of the string. In this case, we arbitrarily define a macro constant - MAX_LENGTH
equal to 100 characters.
The whole array can be initialized with {""}
notation, which zeros out each char
element in the array.
When storing the string values in the already initialized array, the assignment operator is not permitted, and special memory copying functions should be employed like strcpy
.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LENGTH 100
#define NUM_STRINGS 10
int main() {
char arr[NUM_STRINGS][MAX_LENGTH] = {""};
arr2[0] = "string literal"; // Not permitted
strcpy(arr[0], "hello world");
printf("%s\n", arr[0]);
printf("%s\n", strcpy(arr[0], "hello world"));
exit(EXIT_SUCCESS);
}
Output:
hello world
hello world
Alternatively, we can initialize the two-dimensional char
array instantly when declared. The modern C11 standard allows to initialize char
arrays with string literals and even store null byte at the end of the string automatically when the array length is larger than the string itself.
Initializer list notation is similar to the C++ syntax. Each curly braced element is supposed to be stored in a consecutive memory region of length MAX_LENGTH
. In case the initializer notation specifies the fewer elements than the array size, the remaining elements are implicitly initialized with \0
bytes.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LENGTH 100
#define NUM_STRINGS 10
int main() {
char arr2[NUM_STRINGS][MAX_LENGTH] = {{"first string"},
{"second string"},
{"third string"},
{"fourth string"},
{"fifth string"}};
for (int i = 0; i < NUM_STRINGS; ++i) {
printf("%s, ", arr2[i]);
}
exit(EXIT_SUCCESS);
}
Output:
first string, second string, third string, fourth string, fifth string, , , , , ,
Use the char*
Array Notation to Declare Array of Strings in C
char*
is the type that is generally used to store character strings. Declaring the array of char*
gives us the fixed number of pointers pointing to the same number of character strings. It can be initialized with string literals as shown in the following example, assigned to or copied using special functions provided in the header string.h
.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LENGTH 100
#define NUM_STRINGS 10
int main() {
char* arr3[NUM_STRINGS] = {"first string", "second string", "third string",
"fourth string", "fifth string"};
char* str1 = "string literal";
arr3[8] = str1;
arr3[9] = "hello there";
for (int i = 0; i < NUM_STRINGS; ++i) {
printf("%s, ", arr3[i]);
}
printf("\n");
exit(EXIT_SUCCESS);
}
Output:
first string, second string, third string, fourth string, fifth string, (null), (null), (null), string literal, hello there,
As an alternative, one can specify the {}
braces only, which will initialize the pointers in the array to null
, and they can be later used to stored other string addresses, or the programmer can even allocate dynamic memory.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define NUM_STRINGS 10
int main() {
char *arr4[NUM_STRINGS] = {};
for (int i = 0; i < NUM_STRINGS; ++i) {
printf("%s, ", arr4[i]);
}
printf("\n");
exit(EXIT_SUCCESS);
}
Output:
(null), (null), (null), (null), (null), (null), (null), (null), (null), (null),
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C Array
- How to Copy Char Array in C
- How to Dynamically Allocate an Array in C
- How to Clear Char Array in C
- How to Initialize Char Array in C
- How to Print Char Array in C