How to Clear Char Array in C
-
Use the
memset()
Function to Clear achar
Array in C -
Use
bzero
orexplicit_bzero
Functions to Clear achar
Array in C -
Use a Loop to Clear a
char
Array in C -
Use the
strcpy()
Function to Clear achar
Array in C -
Use
free()
to Clear a Dynamicchar
Array in C - Conclusion
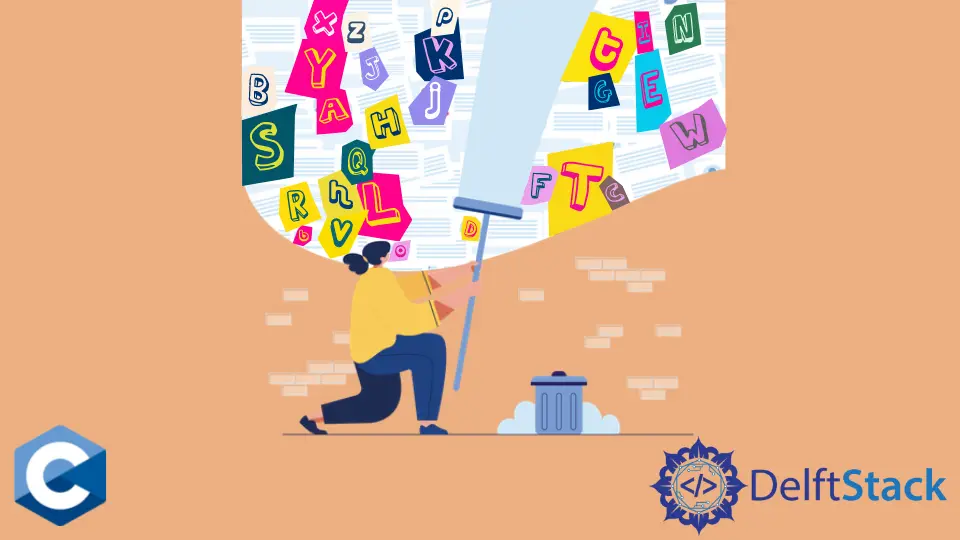
Clearing a character array in C is a critical task in programming, especially when working with strings.
It involves erasing any existing data from an array of characters to make it ready for new information. This process is vital to prevent unwanted residual values that may interfere with the correct functioning of your program.
In this guide, we will explore several methods to clear a char
array in C effectively. These methods range from using specialized functions like memset()
, bzero
, or explicit_bzero
to more manual approaches involving loops and string manipulation functions like strcpy()
.
We will also learn how to clear a dynamic char
array using the free()
function, so let’s get started.
Use the memset()
Function to Clear a char
Array in C
The memset()
function is part of the <string.h>
header file in C. It is primarily used to fill a block of memory with a specified value.
This function is widely employed for tasks like initializing arrays, clearing memory buffers, and more.
Syntax:
void* memset(void* ptr, int value, size_t num);
ptr
: A pointer to the memory area that you want to fill.value
: The value to be set (usually an integer).num
: The number of bytes to be set to the value.
To clear a char
array using memset()
, you pass the array’s address, the value you want to set (usually 0
or '\0'
for a character array), and the size of the array.
Example 1:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void printCharArray(char *arr, size_t len) {
printf("arr: ");
for (size_t i = 0; i < len; ++i) {
printf("%c, ", arr[i]);
}
printf("\n");
}
#define LENGTH 20
int main() {
char c_arr[LENGTH] = {'a', 'b', 'c', 'd', 'e', 'f', 'g'};
printCharArray(c_arr, LENGTH);
memset(c_arr, 0, LENGTH);
printCharArray(c_arr, LENGTH);
exit(EXIT_SUCCESS);
}
This code includes three standard C libraries: stdlib.h
, string.h
, and stdio.h
. These are necessary for functions like printf()
, memset()
, and memory allocation.
There’s a function printCharArray
defined, which takes a pointer to a character array (char *arr
) and its length (size_t len
) as arguments. Inside the function, it prints each character in the array using a loop.
In the main()
function, a constant LENGTH
is defined with a value of 20
.
A character array, c_arr
, of length 20
is then declared and initialized with the values 'a'
, 'b'
, 'c'
, 'd'
, 'e'
, 'f'
, 'g'
. This array is then passed to the printCharArray
function along with its length.
After printing the initial content of c_arr
, the memset()
function is used to set all the elements of c_arr
to 0
, which effectively clears the array. The function printCharArray
is called again to display the modified content of c_arr
.
Output:
arr: a, b, c, d, e, f, g, , , , , , , , , , , , , ,
arr: , , , , , , , , , , , , , , , , , , , ,
Alternatively, memset()
can be called with the specific character as the constant byte argument, which can be useful for initializing every given array element with the same values. In this case, we arbitrarily choose the character '0'
to fill the array, resulting in a cleared memory region.
Example 2:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void printCharArray(char *arr, size_t len) {
printf("arr: ");
for (size_t i = 0; i < len; ++i) {
printf("%c, ", arr[i]);
}
printf("\n");
}
#define LENGTH 20
int main() {
char c_arr2[LENGTH] = "temp array";
printCharArray(c_arr2, LENGTH);
memset(c_arr2, '0', LENGTH);
printCharArray(c_arr2, LENGTH);
exit(EXIT_SUCCESS);
}
In this code, the printCharArray
function is defined, which takes a pointer to a character array (char *arr
) and its length (size_t len
) as parameters. This function prints each character in the array, separated by commas.
In the main
function, a char
array c_arr2
of length 20
is declared and initialized with the string "temp array"
. The printCharArray
function is called to display the contents of the array.
Subsequently, the memset()
function is used to set all the elements of c_arr2
to the character '0'
.
Afterward, the printCharArray
function is called again to display the modified array. This time, it will show that all elements are now '0'
characters.
Output:
arr: t, e, m, p, , a, r, r, a, y, , , , , , , , , , ,
arr: 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
Use bzero
or explicit_bzero
Functions to Clear a char
Array in C
The bzero
and explicit_bzero
functions are part of the <string.h>
header file in C. They are designed for clearing memory areas, such as char
arrays, by setting all bytes in the area to zero.
These functions are particularly useful when dealing with sensitive data that needs to be securely erased.
Syntax:
void bzero(void *s, size_t n);
void explicit_bzero(void *s, size_t n);
s
: A pointer to the memory area that you want to clear.n
: The number of bytes to clear starting from the pointers
.
explicit_bzero
is an alternative to bzero
that guarantees to conduct the write
operation regardless of compiler optimizations. If instructed by the user, the compiler analyzes code for redundant instructions and removes them, and the explicit_bzero
function is designed for this specific scenario.
Example:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void printCharArray(char *arr, size_t len) {
printf("arr: ");
for (size_t i = 0; i < len; ++i) {
printf("%c, ", arr[i]);
}
printf("\n");
}
#define LENGTH 20
int main() {
char c_arr[LENGTH] = {'a', 'b', 'c', 'd', 'e', 'f', 'g'};
printCharArray(c_arr, LENGTH);
bzero(c_arr, LENGTH);
printCharArray(c_arr, LENGTH);
explicit_bzero(c_arr, LENGTH);
printCharArray(c_arr, LENGTH);
exit(EXIT_SUCCESS);
}
The code defines a function printCharArray
that takes a pointer to a character array (char *arr
) and its length (size_t len
). This function iterates through the array and prints each character along with a comma, effectively displaying the contents of the array.
In the main()
function, a character array (c_arr
) of length LENGTH
is declared and initialized with the first seven elements ('a'
to 'g'
). This array is then passed to the printCharArray
function, displaying its contents.
The bzero
function is then used to clear the contents of c_arr
. This function sets all bytes in the specified memory block to zero.
After this operation, the array is essentially empty, and it is again passed to printCharArray
for display.
Next, explicit_bzero
is called. This function is similar to bzero
, but it’s specifically designed for zeroing sensitive data to prevent it from being optimized away by the compiler.
In this case, it’s used to clear the array.
Output:
arr: a, b, c, d, e, f, g, , , , , , , , , , , , , ,
arr: , , , , , , , , , , , , , , , , , , , ,
arr: , , , , , , , , , , , , , , , , , , , ,
Use a Loop to Clear a char
Array in C
A loop is a control structure that allows for repetitive execution of a block of code. Using a loop to clear a char
array involves iterating through each element of the array and assigning a known value (usually null or zero).
In this example, we use a for
loop to iterate over each element of the array and set it to the null character \0
. This achieves the same result as using memset()
.
#include <stdio.h>
#define ARRAY_LENGTH 10
void clearArray(char arr[], size_t len) {
for (size_t i = 0; i < len; i++) {
arr[i] = '\0'; // Set each element to null
}
}
int main() {
char myArray[ARRAY_LENGTH] = "Hello";
printf("Before clearing: %s\n", myArray);
clearArray(myArray, ARRAY_LENGTH);
printf("After clearing: %s\n", myArray);
return 0;
}
The clearArray
function takes a character array (arr
) and its length (len
) as parameters. It uses a for
loop to set each element to null ('\0'
).
In main()
, the myArray
is declared and initialized with the string "Hello"
. The contents of myArray
are printed before clearing.
The clearArray
function is called to clear the array. The contents of the array are printed again to observe the effect of the clearing operation.
Output:
Before clearing: Hello
After clearing:
Use the strcpy()
Function to Clear a char
Array in C
The strcpy()
function is primarily used for copying strings in C. It takes two arguments: the destination
string and the source
string.
When used for clearing a character array, an empty string is typically used as the source.
Syntax:
char* strcpy(char* destination, const char* source);
char*
: This specifies that the function returns a pointer to a character, which is thedestination
string.destination
: This is a pointer to thedestination
string where thesource
string will be copied.const char* source
: This is a pointer to thesource
string that will be copied to the destination.
The function strcpy()
copies characters from the source
string to the destination
string until it reaches the null terminator ('\0'
) of the source
string. It then appends a null terminator to the destination
string to mark its end.
To clear a character array using strcpy()
, you pass the array itself as the destination and an empty string (""
) as the source.
Example:
#include <stdio.h>
#include <string.h>
#define ARRAY_LENGTH 10
int main() {
char myArray[ARRAY_LENGTH] = "Hello";
printf("Before clearing: %s\n", myArray);
strcpy(myArray, "");
printf("After clearing: %s\n", myArray);
return 0;
}
This code includes the necessary header files for standard input/output and string manipulation.
In main()
, myArray
is declared and initialized with the string "Hello"
. The contents of myArray
are printed before clearing.
The strcpy()
function is then used to copy an empty string (""
) into myArray
, effectively clearing it. Finally, the contents of the array are printed again to observe the effect of the clearing operation.
Output:
Before clearing: Hello
After clearing:
Use free()
to Clear a Dynamic char
Array in C
Dynamic memory allocation in C allows you to allocate memory at runtime using functions like malloc()
, calloc()
, and realloc()
. This memory needs to be explicitly deallocated to prevent memory leaks.
Failing to deallocate dynamically allocated memory can lead to memory leaks, which can cause your program to consume excessive resources and potentially crash. The free()
function ensures that the memory is properly released when it is no longer needed.
Syntax:
void free(void* ptr);
ptr
: A pointer to the memory block that was previously allocated using functions likemalloc()
,calloc()
, orrealloc()
.
free()
a pointer that wasn’t dynamically allocated can lead to undefined behavior.To clear a dynamic character array, you need to use free()
to release the allocated memory. This should be done when you’re finished using the array and want to avoid memory leaks.
Example:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void clearAndFreeCharArray(char* array) {
if (array != NULL) {
free(array);
array = NULL;
}
}
int main() {
// Dynamically allocate memory for a character array
char* dynamicArray = (char*)malloc(10 * sizeof(char));
if (dynamicArray == NULL) {
printf("Memory allocation failed. Exiting...\n");
return 1;
}
// Initialize the array
strcpy(dynamicArray, "Hello");
printf("Before clearing: %s\n", dynamicArray);
// Clear and free the dynamic array
clearAndFreeCharArray(dynamicArray);
// Attempting to print the cleared array will result in undefined behavior
printf("After clearing: %s\n",
dynamicArray); // This might cause a segmentation fault or produce
// unexpected results
return 0;
}
In this example, the clearAndFreeCharArray
function is defined to clear and free a dynamically allocated character array.
Inside the function, it first checks if the provided pointer array
is not NULL
to avoid potential errors. If it’s not NULL
, the free
function is called to release the allocated memory, and the pointer array
is set to NULL
to prevent any further access to the released memory.
In the main
function, memory for a character array (dynamicArray
) is dynamically allocated using malloc
. This allocates space for ten characters.
The program checks if the allocation is successful. If not, it prints an error message and exits with a return value of 1
.
Next, the strcpy
function is used to initialize dynamicArray
with the string "Hello"
. The program then prints the contents of the array.
The clearAndFreeCharArray
function is called to clear and free the dynamically allocated memory.
Finally, an attempt to print the cleared array is made. This operation is an undefined behavior and may lead to a segmentation fault or produce unexpected results since the memory has already been deallocated.
Output:
Before clearing: Hello
After clearing:
It’s crucial not to use the array after freeing it to avoid undefined behavior, which, in this case, might lead to a segmentation fault or unexpected results. Always free dynamically allocated memory to prevent memory leaks and ensure efficient memory management.
Conclusion
Clearing character arrays in C is a fundamental operation for managing strings and preventing unwanted residual data. This process is crucial to maintain program integrity.
Throughout this guide, we’ve explored several effective methods for achieving this goal, and we also learned how to clear dynamic char
array. From specialized functions like memset()
, bzero
, and explicit_bzero
to manual approaches using loops and string manipulation functions like strcpy()
, choosing the appropriate method depends on the specific needs of the program.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook