How to Initialize Char Array in C
-
Use
{}
Curly Braced List Notation to Initialize achar
Array in C -
Use String Assignment to Initialize a
char
Array in C -
Use
{{ }}
Double Curly Braces to Initialize 2Dchar
Array in C
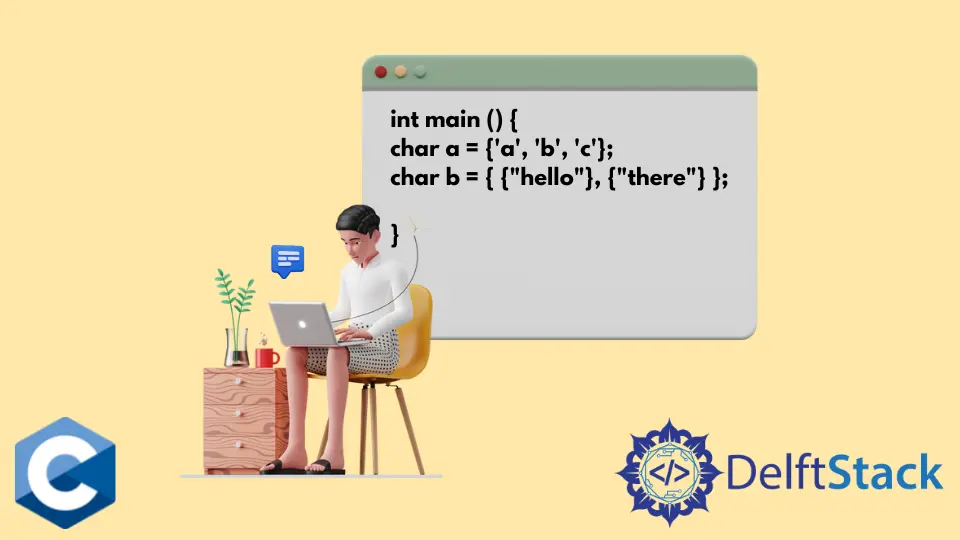
This article will demonstrate multiple methods of how to initialize a char
array in C.
Use {}
Curly Braced List Notation to Initialize a char
Array in C
A char
array is mostly declared as a fixed-sized structure and often initialized immediately. Curly braced list notation is one of the available methods to initialize the char
array with constant values.
It’s possible to specify only the portion of the elements in the curly braces as the remainder of chars is implicitly initialized with a null byte value.
It can be useful if the char
array needs to be printed as a character string. Since there’s a null byte character guaranteed to be stored at the end of valid characters, then the printf
function can be efficiently utilized with the %s
format string specifier to output the array’s content.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void printCharArray(char *arr, size_t len) {
printf("arr: ");
for (int i = 0; i < len; ++i) {
printf("%c, ", arr[i]);
}
printf("\n");
}
enum { LENGTH = 21, HEIGHT = 5 };
int main() {
char c_arr[LENGTH] = {'a', 'b', 'c', 'd', 'e', 'f', 'g'};
printCharArray(c_arr, LENGTH);
exit(EXIT_SUCCESS);
}
Output:
arr: a, b, c, d, e, f, g, , , , , , , , , , , , , , ,
Use String Assignment to Initialize a char
Array in C
Another useful method to initialize a char
array is to assign a string value in the declaration statement. The string literal should have fewer characters than the length of the array; otherwise, there will be only part of the string stored and no terminating null character at the end of the buffer.
Thus, if the user will try to print the array’s content with the %s
specifier, it might access the memory region after the last character and probably will throw a fault.
Note that c_arr
has a length of 21 characters and is initialized with a 20 char
long string. As a result, the 21st character in the array is guaranteed to be \0
byte, making the contents a valid character string.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void printCharArray(char *arr, size_t len) {
printf("arr: ");
for (size_t i = 0; i < len; ++i) {
printf("%c, ", arr[i]);
}
printf("\n");
}
enum { LENGTH = 21, HEIGHT = 5 };
int main() {
char c_arr[LENGTH] = "array initialization";
char c_arr2[LENGTH] = "array initialization.f";
printCharArray(c_arr, LENGTH);
printCharArray(c_arr2, LENGTH);
printf("%s\n", c_arr);
printf("%s\n", c_arr2);
exit(EXIT_SUCCESS);
}
Output:
arr: a, r, r, a, y, , i, n, i, t, i, a, l, i, z, a, t, i, o, n, ,
arr: a, r, r, a, y, , i, n, i, t, i, a, l, i, z, a, t, i, o, n, .,
array initialization
array initialization.//garbage values//
Use {{ }}
Double Curly Braces to Initialize 2D char
Array in C
The curly braced list can also be utilized to initialize two-dimensional char
arrays. In this case, we declare a 5x5 char
array and include five braced strings inside the outer curly braces.
Note that each string literal in this example initializes the five-element rows of the matrix. The content of this two-dimensional array can’t be printed with %s
specifier as the length of each row matches the length of the string literals; thus, there’s no terminating null byte stored implicitly during the initialization. Usually, the compiler will warn if the string literals are larger than the array rows.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
enum { LENGTH = 5, HEIGHT = 5 };
int main() {
char c_arr[HEIGHT][HEIGHT] = {
{"hello"}, {"there"}, {"multi"}, {"dimen"}, {"sion."}};
for (size_t i = 0; i < HEIGHT; ++i) {
for (size_t j = 0; j < HEIGHT; ++j) {
printf("%c, ", c_arr[i][j]);
}
printf("\n");
}
printf("\n");
exit(EXIT_SUCCESS);
}
Output:
h, e, l, l, o,
t, h, e, r, e,
m, u, l, t, i,
d, i, m, e, n,
s, i, o, n, .,
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook