如何在 C 语言中获取数组的大小
Satishkumar Bharadwaj
2023年10月12日
C
C Array
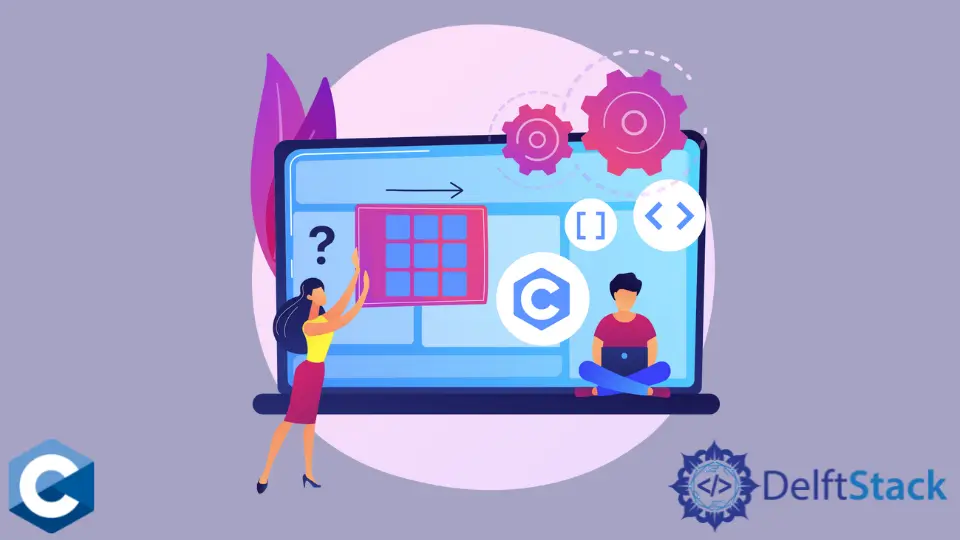
本教程介绍了如何在 C 语言中确定一个数组的长度,sizeof()
运算符用于获取一个数组的大小/长度。
sizeof()
运算符在 C 语言中确定一个数组的大小
sizeof()
运算符是一个编译时的一元运算符。它用于计算操作数的大小。它返回变量的大小。sizeof()
运算符以字节为单位给出大小。
sizeof()
运算符用于任何数据类型,如 int
、float
、char
等基元,也用于 array
、struct
等非基元数据类型。它返回分配给该数据类型的内存。
The output may be different on different machines like a 32-bit system can show different output and a 64-bit system can show different of the same data types.
sizeof()
的语法
sizeof(operand)
operand
是一个数据类型或任何操作数。
sizeof()
在 C 语言中用于原始数据类型的操作数
该程序使用 int
、float
作为原始数据类型。
#include <stdio.h>
int main(void) {
printf("Size of char data type: %u\n", sizeof(char));
printf("Size of int data type: %u\n", sizeof(int));
printf("Size of float data type: %u\n", sizeof(float));
printf("Size of double data type: %u\n", sizeof(double));
return 0;
}
输出:
Size of char data type: 1
Size of int data type: 4
Size of float data type: 4
Size of double data type: 8
用 C 语言获取数组的长度
如果我们用数组的总大小除以数组元素的大小,就可以得到数组中的元素数。程序如下。
#include <stdio.h>
int main(void) {
int number[16];
size_t n = sizeof(number) / sizeof(number[0]);
printf("Total elements the array can hold is: %d\n", n);
return 0;
}
输出:
Total elements the array can hold is: 16
当一个数组作为参数被传送到函数中时,它将被视为一个指针。sizeof()
运算符返回的是指针大小而不是数组大小。所以在函数内部,这个方法不能用。相反,传递一个额外的参数 size_t size
来表示数组中的元素数量。
#include <stdio.h>
#include <stdlib.h>
void printSizeOfIntArray(int intArray[]);
void printLengthIntArray(int intArray[]);
int main(int argc, char* argv[]) {
int integerArray[] = {0, 1, 2, 3, 4, 5, 6};
printf("sizeof of the array is: %d\n", (int)sizeof(integerArray));
printSizeOfIntArray(integerArray);
printf("Length of the array is: %d\n",
(int)(sizeof(integerArray) / sizeof(integerArray[0])));
printLengthIntArray(integerArray);
}
void printSizeOfIntArray(int intArray[]) {
printf("sizeof of the parameter is: %d\n", (int)sizeof(intArray));
}
void printLengthIntArray(int intArray[]) {
printf("Length of the parameter is: %d\n",
(int)(sizeof(intArray) / sizeof(intArray[0])));
}
输出(在 64 位的 Linux 操作系统中):
sizeof of the array is : 28 sizeof of the parameter is : 8 Length of the array
is : 7 Length of the parameter is : 2
输出(在 32 位 Windows 操作系统中):
sizeof of the array is : 28 sizeof of the parameter is : 4 Length of the array
is : 7 Length of the parameter is : 1
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe