C에서 배열의 크기 얻기
Satishkumar Bharadwaj
2023년10월12일
C
C Array
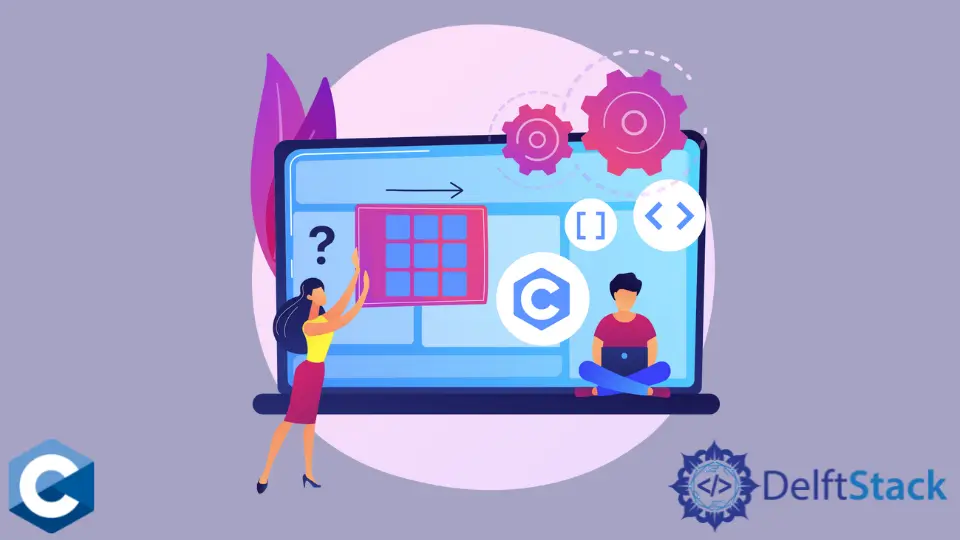
이 튜토리얼은 C에서 배열의 길이를 결정하는 방법을 소개합니다.sizeof()
연산자는 배열의 크기/길이를 얻는 데 사용됩니다.
C에서 배열의 크기를 결정하는sizeof()
연산자
sizeof()
연산자는 컴파일 타임 단항 연산자입니다. 피연산자의 크기를 계산하는 데 사용됩니다. 변수의 크기를 반환합니다. sizeof()
연산자는 바이트 단위로 크기를 제공합니다.
sizeof()
연산자는int
,float
,char
와 같은 기본형과 같은 모든 데이터 유형과array
,struct
와 같은 비 기본형 데이터 유형에 사용됩니다. 해당 데이터 유형에 할당 된 메모리를 반환합니다.
32 비트 시스템은 다른 출력을 표시 할 수 있고 64 비트 시스템은 동일한 데이터 유형을 다르게 표시 할 수있는 것처럼 출력은 다른 시스템에서 다를 수 있습니다.
sizeof()
의 구문:
sizeof(operand)
operand
는 데이터 유형 또는 피연산자입니다.
C의 원시 데이터 유형에 대한sizeof()
연산자
이 프로그램은 기본 데이터 유형으로int
,float
를 사용합니다.
#include <stdio.h>
int main(void) {
printf("Size of char data type: %u\n", sizeof(char));
printf("Size of int data type: %u\n", sizeof(int));
printf("Size of float data type: %u\n", sizeof(float));
printf("Size of double data type: %u\n", sizeof(double));
return 0;
}
출력:
Size of char data type: 1
Size of int data type: 4
Size of float data type: 4
Size of double data type: 8
C에서 배열 길이 얻기
배열의 전체 크기를 배열 요소의 크기로 나누면 배열의 요소 수를 얻습니다. 프로그램은 다음과 같습니다.
#include <stdio.h>
int main(void) {
int number[16];
size_t n = sizeof(number) / sizeof(number[0]);
printf("Total elements the array can hold is: %d\n", n);
return 0;
}
출력:
Total elements the array can hold is: 16
배열이 매개 변수로 함수에 전달되면 포인터로 취급됩니다. sizeof()
연산자는 배열 크기 대신 포인터 크기를 반환합니다. 따라서 함수 내에서이 방법은 작동하지 않습니다. 대신 추가 매개 변수 size_t size
를 전달하여 배열의 요소 수를 나타냅니다.
#include <stdio.h>
#include <stdlib.h>
void printSizeOfIntArray(int intArray[]);
void printLengthIntArray(int intArray[]);
int main(int argc, char* argv[]) {
int integerArray[] = {0, 1, 2, 3, 4, 5, 6};
printf("sizeof of the array is: %d\n", (int)sizeof(integerArray));
printSizeOfIntArray(integerArray);
printf("Length of the array is: %d\n",
(int)(sizeof(integerArray) / sizeof(integerArray[0])));
printLengthIntArray(integerArray);
}
void printSizeOfIntArray(int intArray[]) {
printf("sizeof of the parameter is: %d\n", (int)sizeof(intArray));
}
void printLengthIntArray(int intArray[]) {
printf("Length of the parameter is: %d\n",
(int)(sizeof(intArray) / sizeof(intArray[0])));
}
출력 (64 비트 Linux OS) :
sizeof of the array is : 28 sizeof of the parameter is : 8 Length of the array
is : 7 Length of the parameter is : 2
출력 (32 비트 Windows OS) :
sizeof of the array is : 28 sizeof of the parameter is : 4 Length of the array
is : 7 Length of the parameter is : 1
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다