Cómo obtener el tamaño del array en C
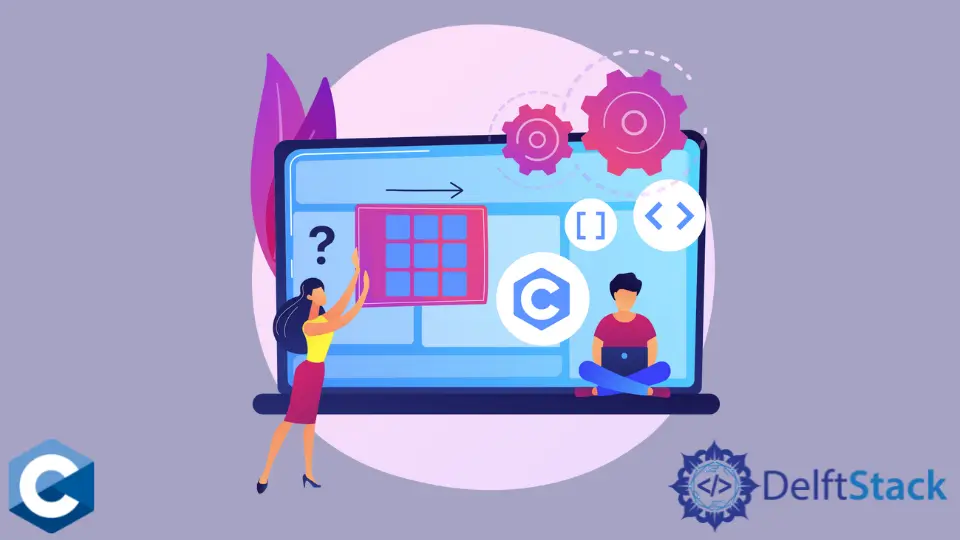
Este tutorial introduce cómo determinar la longitud de un array en C. El operador sizeof()
se utiliza para obtener el tamaño/longitud de un array.
El operador sizeof()
para determinar el tamaño de un array en C
El operador sizeof()
es un operador unario en tiempo de compilación. Se usa para calcular el tamaño de su operando. Devuelve el tamaño de una variable. El operador sizeof()
da el tamaño en la unidad de byte.
El operador sizeof()
se usa para cualquier tipo de datos como primitivos como int
, float
, char
, y también para tipos de datos no primitivos como array
, struct
. Devuelve la memoria asignada a ese tipo de datos.
La salida puede ser diferente en diferentes máquinas, como un sistema de 32 bits puede mostrar una salida diferente y un sistema de 64 bits puede mostrar diferentes de los mismos tipos de datos.
La sintaxis de sizeof()
:
sizeof(operand)
- El
operand
es un tipo de datos o cualquier operando.
sizeof()
Operador para tipos de datos primitivos en C
Este programa usa un int
, float
como un tipo de datos primitivos.
#include <stdio.h>
int main(void) {
printf("Size of char data type: %u\n", sizeof(char));
printf("Size of int data type: %u\n", sizeof(int));
printf("Size of float data type: %u\n", sizeof(float));
printf("Size of double data type: %u\n", sizeof(double));
return 0;
}
Resultado:
Size of char data type : 1 Size of int data type : 4 Size of float data
type : 4 Size of double data type : 8
Obtener la longitud del array en C
Si dividimos el tamaño total del array por el tamaño del elemento del array, obtenemos el número de elementos del array. El programa es el siguiente:
#include <stdio.h>
int main(void) {
int number[16];
size_t n = sizeof(number) / sizeof(number[0]);
printf("Total elements the array can hold is: %d\n", n);
return 0;
}
Resultado:
Total elements the array can hold is: 16
Cuando se pasa un array como parámetro a la función, se trata como un puntero. El operador sizeof()
devuelve el tamaño del puntero en lugar del tamaño del array. Así que dentro de las funciones, este método no funcionará. En su lugar, pasa un parámetro adicional size_t size
para indicar el número de elementos en el array.
#include <stdio.h>
#include <stdlib.h>
void printSizeOfIntArray(int intArray[]);
void printLengthIntArray(int intArray[]);
int main(int argc, char* argv[]) {
int integerArray[] = {0, 1, 2, 3, 4, 5, 6};
printf("sizeof of the array is: %d\n", (int)sizeof(integerArray));
printSizeOfIntArray(integerArray);
printf("Length of the array is: %d\n",
(int)(sizeof(integerArray) / sizeof(integerArray[0])));
printLengthIntArray(integerArray);
}
void printSizeOfIntArray(int intArray[]) {
printf("sizeof of the parameter is: %d\n", (int)sizeof(intArray));
}
void printLengthIntArray(int intArray[]) {
printf("Length of the parameter is: %d\n",
(int)(sizeof(intArray) / sizeof(intArray[0])));
}
Salida (en un sistema operativo Linux de 64 bits):
sizeof of the array is : 28 sizeof of the parameter is : 8 Length of the array
is : 7 Length of the parameter is : 2
Salida (en un sistema operativo Windows de 32 bits):
sizeof of the array is : 28 sizeof of the parameter is : 4 Length of the array
is : 7 Length of the parameter is : 1