How to Convert Integer to Char in C
-
Add
'0'
to Convertint
tochar
in C -
Assign an
int
Value to achar
Value to Convertint
tochar
in C -
Use the
sprintf()
Function to Convertint
tochar
in C - Conclusion
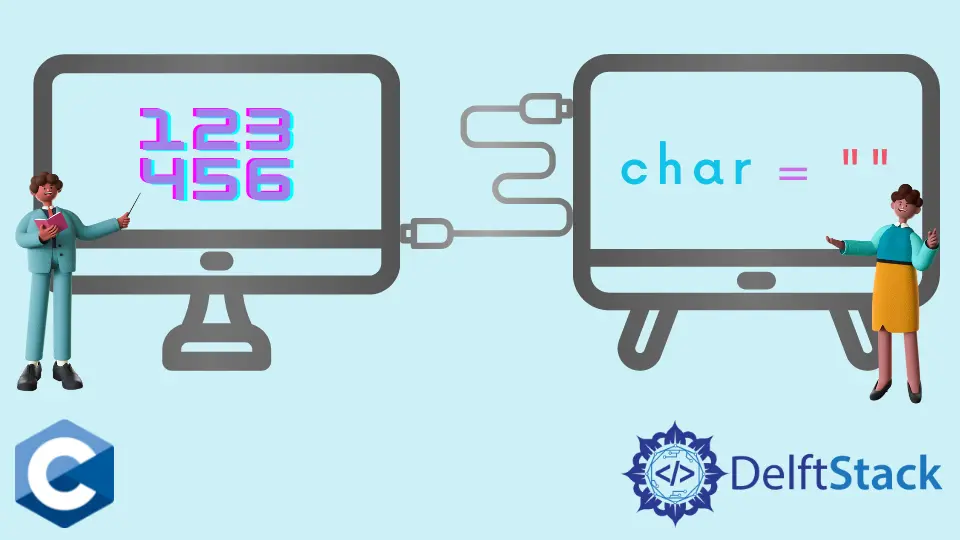
Converting an integer to a character in the C programming language can be essential in various scenarios. Characters in C are represented as ASCII values, making it relatively straightforward to perform this conversion.
This tutorial explores several methods to convert an integer to a character in C, ranging from simple addition to explicit type casting and utilizing the sprintf()
function.
Add '0'
to Convert int
to char
in C
The ASCII value of '0'
is 48. Therefore, adding its value to an integer will convert it into the corresponding character.
Here’s a complete C program demonstrating this method:
#include <stdio.h>
int main(void) {
int number = 71;
char charValue = number + '0';
printf("The character value is: %c", charValue);
return 0;
}
Output:
The character value is: w
We know that '0'
has an ASCII value of 48, and adding 71 to 48 results in 119. In the ASCII character set, the value 119 corresponds to the character w
.
Therefore, the program’s output displays the character value w
.
Moreover, to convert integers within the range 0 to 9 to characters, we can use the same approach:
#include <stdio.h>
int main(void) {
int number = 7;
char charValue = number + '0';
printf("The value is: %c", charValue);
return 0;
}
Output:
The value is: 7
Here, the code declares a character variable named charValue
and also computes its value by adding the integer stored in number
to the ASCII value of '0'
.
In C, characters are represented as integers based on the ASCII encoding, so this operation effectively converts the integer 7 to the character '7'
.
Another program to convert an integer value to a character is shown below:
#include <stdio.h>
int main(void) {
char charValue[] = "stringValueX";
int anyNumber;
for (anyNumber = 0; anyNumber < 10; ++anyNumber) {
charValue[11] = anyNumber + '0';
puts(charValue);
}
return 0;
}
Output:
stringValue0
stringValue1
stringValue2
stringValue3
stringValue4
stringValue5
stringValue6
stringValue7
stringValue8
stringValue9
The provided code above begins by initializing a character array called charValue
with the string "stringValueX"
and declaring an integer variable named anyNumber
.
It then enters a for
loop that iterates from 0 to 9. Within the loop, the code replaces the character at index 11 of charValue
by adding the value of anyNumber
to the ASCII value of '0'
, effectively substituting the X
with numbers from 0 to 9.
The puts(charValue)
function prints the modified charValue
in each iteration on separate lines. As a result, the program generates an output where "stringValueX"
is displayed with X
replaced by numbers 0 to 9, each appearing on its line.
Assign an int
Value to a char
Value to Convert int
to char
in C
Another way to convert an integer value to a character value is by assigning the integer directly to a character variable. The character value corresponding to the integer value will be printed.
Here’s an example:
#include <stdio.h>
int main(void) {
int number = 65;
char charvalue = number;
printf("The character value: %c", charvalue);
return 0;
}
Output:
The character value: A
We can also use explicit type casting to convert an integer to a character. See the example code below.
#include <stdio.h>
int main(void) {
int number = 67;
char charValue = (char)number;
printf("The character value = %c", charValue);
return 0;
}
Output:
The character value = C
Here, the program explicitly casts the integer value of number
to a character using (char)
before the variable name. This type casting tells the compiler to treat number
as a character.
Use the sprintf()
Function to Convert int
to char
in C
The sprintf()
function works the same as the printf()
function, but instead of sending output to the console, it returns the formatted string. It allows us to convert an integer to a character and store it in a string.
Syntax of sprintf()
int sprintf(char *strValue, const char *format, [ arg1, arg2, ... ]);
strValue
is a pointer to thechar
data type.format
is used to display the type of output along with the placeholder.[arg1,arg2...]
are the integer(s) to be converted.
The function writes the data in the string pointed to by strValue
and returns the number of characters written to strValue
, excluding the null character. The return value is generally discarded.
If any error occurs during the operation, it returns -1
.
Here’s an example:
#include <stdio.h>
int main(void) {
int number = 72;
char charValue[1];
sprintf(charValue, "%c", number);
printf("The character value = %s", charValue);
return 0;
}
Output:
The character value = H
In this example, we used sprintf()
to convert the integer 72 to the character H
and stored it in the charValue
array. It is crucial to ensure that the character array (charValue
) is large enough to hold the converted char.
Conclusion
In this tutorial, we’ve delved into multiple approaches to convert integers to characters in the C programming language. Each method offers its advantages and use cases, enabling you to choose the most suitable approach based on your specific programming needs.
Related Article - C Integer
- Integer Division in C
- Difference Between Unsigned Int and Signed Int in C
- How to Convert Char* to Int in C
- How to Convert a String to Integer in C
- How to Convert an Integer to a String in C