How to Convert a String to Integer in C
-
atoi()
Function to Convert a String to an Integer in C -
strtol()
Function to Convert a String to an Integer in C -
strtoumax()
Function for Convert String to an Integer in C
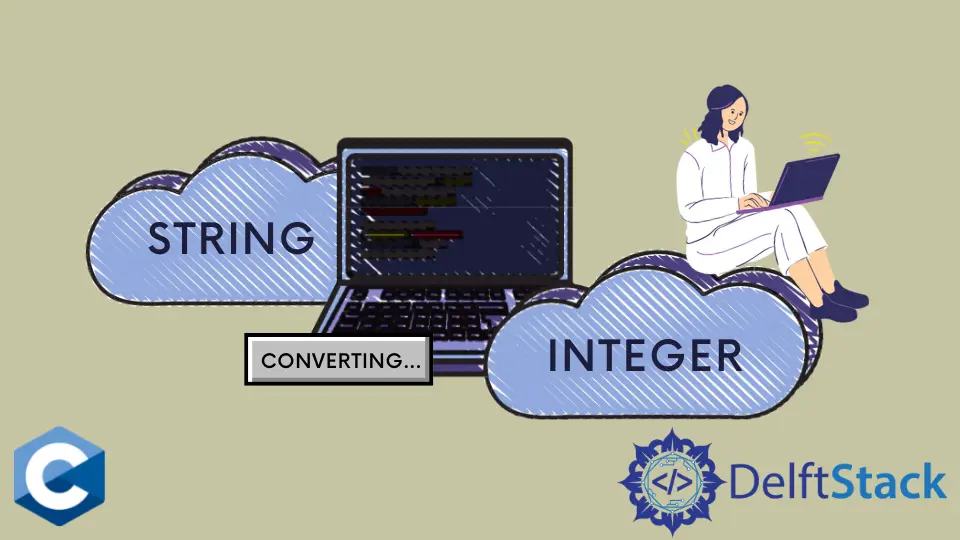
In C programming, converting a string to an integer is a common operation, and there are several methods available to accomplish this task. Three commonly used functions for this purpose are atoi()
, strtol()
, and strtoumax()
.
This comprehensive article will introduce and explain each of these methods, providing examples along the way.
Before diving into the specific functions, let’s briefly discuss the need for converting strings to integers in C programming.
Strings are sequences of characters, while integers are whole numbers. There are numerous situations in programming where you may need to extract numerical information from user input, files, or other sources stored as strings. This conversion process is crucial for processing and manipulating this data effectively.
atoi()
Function to Convert a String to an Integer in C
The atoi()
function converts a string into an integer in the C programming language. atoi()
stands for ASCII to Integer
. The atoi()
function neglects all white spaces at the beginning of the string, converts the characters after the white spaces, and then stops when it reaches the first non-number character.
The atoi()
function returns the integer representation of the string.
We need to include the <stdlib.h>
header to use the atoi()
function.
atoi()
Syntax
int atoi(const char *str);
*str
is a pointer to a string to be converted to an integer.
atoi()
Example Codes
#include <stdio.h>
#include <stdlib.h>
int main(void) {
int value;
char str[20];
strcpy(str, "123");
value = atoi(str);
printf("String value = %s, Int value = %d\n", str, value);
return (0);
}
Output:
String value=123, Int value=123
In this example:
- We include the necessary headers (
<stdio.h>
and<stdlib.h>
). - We define a string
str
containing the numeric characters123
. - We use
atoi()
to convertstr
to an integer and store the result in thevalue
variable. - Finally, we print the integer using
printf()
.
strtol()
Function to Convert a String to an Integer in C
The strtol()
function converts a string into a long integer in the C programming language. The strtol()
function omits all white-spaces characters at the beginning of the string, after it converts the subsequent characters as part of the number, and then stops when it finds the first character that isn’t a number.
The strtol()
function returns the long integer value representation of a string.
We need to include the <stdlib.h>
header to use the atoi()
function.
strtol()
Syntax
long int strtol(const char *string, char **laststr, int basenumber);
*string
is a pointer to a string to be converted to a long integer.**laststr
is a pointer to indicate where the conversion stops. It setslaststr
to the first character that is not part of the converted number, which can be useful for error checking.basenumber
is the base with the range of[2, 36]
.
#include <stdio.h>
#include <stdlib.h>
int main(void) {
char str[10];
char *ptr;
long value;
strcpy(str, " 123");
value = strtol(str, &ptr, 10);
printf("decimal %ld\n", value);
return 0;
}
Output:
decimal 123
In this example:
- We include the necessary headers (
<stdio.h>
and<stdlib.h>
). - We define a string
str
containing the numeric characters" 123"
. - We use
strtol()
to convertstr
to a long integer and store the result in thevalue
variable. - We also provide the base (10 for decimal) as the third argument.
When you run this program, it will produce the output: decimal 123
.
strtoumax()
Function for Convert String to an Integer in C
The strtoumax()
function interprets a string’s content as in the form of an integral number of the specified base. It omits any whitespace character until the first non-whitespace character. It then takes as many characters as possible to form a valid base integer number representation and converts them to an integer value.
strtoumax()
returns the corresponding integer value of a string. This function returns 0 if the conversion is not successfully done.
strtoumax()
Syntax
uintmax_t strtoumax(const char* string, char** last, int basenumber);
*string
is a pointer to a string to be converted to a long integer.**last
is a pointer to indicate where the conversion stops.basenumber
is the base with the range of[2, 36]
.
strtoumax()
Example
#include <stdio.h>
#include <stdlib.h>
int main(void) {
char str[10];
char *ptr;
int value;
strcpy(str, " 123");
printf("The integer value:%d", strtoumax(str, &ptr, 10));
return 0;
}
In this example:
- We include the necessary headers,
<stdio.h>
and<stdlib.h>
, to access the functions we need. - We declare the following variables:
str
: An array of characters (a string) containing the input" 123"
.ptr
: A character pointer that will be used to track the parsing progress bystrtoumax()
.value
: An unsigned integer variable to store the converted value.
- We use the
strtoumax()
function to perform the conversion:str
: The string to convert, which contains the value" 123"
.&ptr
: A pointer toendptr
, allowing us to check where the conversion stopped.10
: The base of the numeric representation, which is 10 (decimal).
When you run this program, it will output:
The long integer value: 123
Converting strings to integers is a common task in C programming, and each of the discussed methods (atoi()
, strtol()
, and strtoumax()
) has its own advantages and use cases. While atoi()
is simple and straightforward, it may lack error handling and base conversion capabilities. On the other hand, strtol()
and strtoumax()
offer better error detection, support for different bases, and are suitable for a wider range of applications.
When choosing a method for string-to-integer conversion in C, consider your specific requirements, such as error handling, base conversion, and the range of integer values you need to handle. By selecting the appropriate method, you can ensure accurate and reliable conversions in your C programs.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C String
- Null Terminated Strings in C
- Scanf String With Spaces in C
- How to Create Formatted Strings in C
- How to Truncate String in C
- How to Concatenate String and Int in C
- How to Trim a String in C