Difference Between Unsigned Int and Signed Int in C
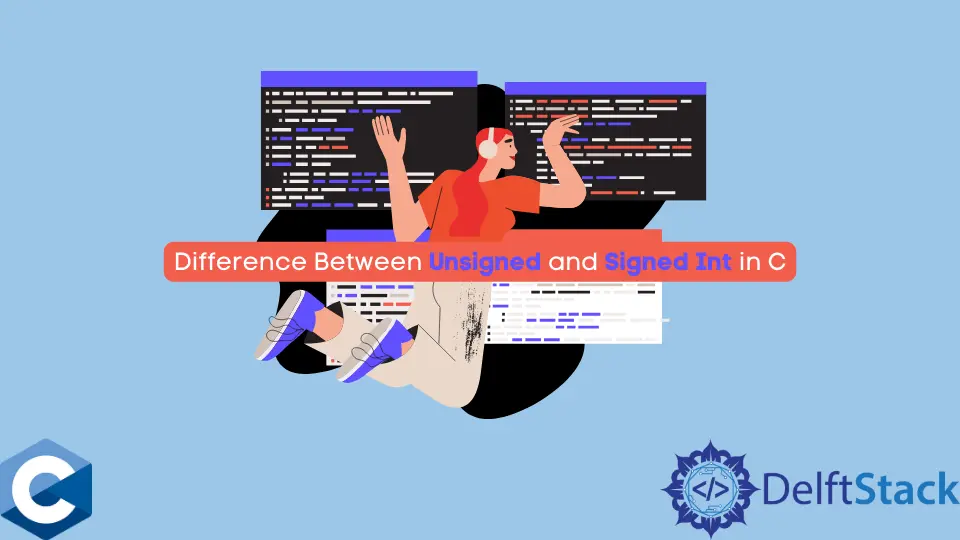
The following article will explore the distinctions between signed and unsigned int
in the C programming language.
Difference Between signed
and unsigned
Int in C
The distinction between a signed int
and an unsigned int
is not nearly as complicated as some think. A signed int
is often represented as int
, but when dealing with unsigned int
, we must write unsigned int
.
The fact that int
is a data type and unsigned
is only a keyword has a specific function connected to the hardware level. This unique purpose creates the more significant difference between the two, which will be covered in further detail in the following parts.
- The
int
data type issigned
and must have a range of at least-32767
to32767
as its minimum range requirement. Thelimits.h
refers to the actual values as their corresponding values forINT MIN
andINT MAX
. - An
unsigned int
has a minimum range that extends from0
up to65535
, inclusive, with the actual maximum value defined by the header file’sUINT_MAX
constant. You don’t need to utilize one of thebits
for asign
if the value isunsigned
.
That implies that you receive a wider variety of positive
encodings at the expense of no negative
ones.
Memory Requirements
First, check how much memory space the signed int
and unsigned int
take up in the computer’s system.
-
Memory requirements for an
int
are4 bytes
, which when multiplied by8
is32 bits
.4x8 = 32 bits
-
Additionally, the
unsigned int
also takes up to4 bytes
of memory space, which adds up to32 bits
.- In the
int
data type, the leftmostbit
in the32-bits
is designated for thesign bit
, which indicates whether the integer is positive or negative.1
indicates a negative value, while0
indicates a positive one. - The range of integers that may currently be stored in an
int
variable is-(2^31)
to2^31-1
, which is equal to-2 147 483 648
to2 147 483 647
).
- In the
When a negative number was input into the computer, it would be represented as the 2's
complement of that number in binary form. Because of this, the beginning bits
of the memory would always be set to one
, and the computer would know that it had encountered a negative number whenever it saw the initial bit
set to 1
.
Whenever we access that number, it reverts to its original form by applying the complement of 2
to it. However, with the unsigned int
data type, there is no such bit
as a sign bit
.
Thus, it now has all 32-bits
available for us to use to store the data. The range of integers that may be stored in an unsigned int
is from 0
to 2^32-1
, which is equivalent to 0
to 4
, 294
, 967
, 295
, inclusive.
The unsigned int
data type’s variable is where the computer typically stores data in binary format.
Let’s have an example. To begin, we will give the variable x
an initial value of the unsigned
type.
Then, when we attempted to save the negative number in the unsigned int
variable x
, the computer used the 2's
complement of the 123
to represent it as a negative number. Then stored that representation in the memory of the x
variable.
unsigned int x;
x = -123;
So, when we saved the negative -123
in the int
data type’s variable y
, the computer took the 2's
complement of the -123
to represent it as a negative integer and then stored that in the memory of the y
variable.
int y;
y = -123;
Unsigned int
variables hold only positive values in standard binary form; no sign bit
was reserved when we attempted to access its memory through the x
variable’s memory. As a result, the computer did not need conversions, and the 2's
compliment that had previously been saved was recovered and shown as a regular binary number.
printf("%d\n", x);
When we attempted to access the memory of the y
variable, the computer determined that it was of the int
data type. It then checked for the bit
to the left of the 32-bit
leftmost position and found that it was 1
.
As a result, it took the 2
’s complement again, added a negative sign(-
), and printed the result.
printf("%d\n", y);
Complete Source Code:
#include <stdio.h>
int main() {
unsigned int x;
x = -123;
int y;
y = -123;
printf("%d\n", x);
printf("%d\n", y);
return 0;
}
Output:
-123
-123
Conclusion
Now we’re familiar with the primary distinctions between signed
and unsigned
integers, you should also be aware that several benefits and drawbacks are associated with both forms of data, the balance of which relies entirely on the needs that you have.
For example, you should use unsigned int
if you wish to store enormous numbers, and you are sure there will never be a negative value. Otherwise, it would help if you used the int
data type.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn