Integer Division in C
- Integer Division in C
- Rules for Integer and Float Division in C
- Integer Division and the Modulus Operator in C
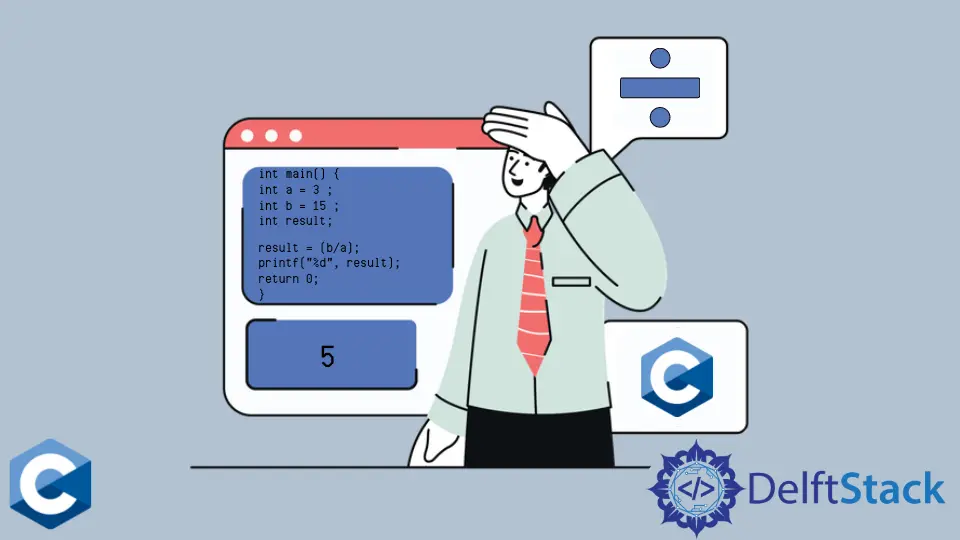
This article demonstrates how the integer division operation works in C.
Integer Division in C
In C, we may divide an integer by performing the division operation on it with another integer or with any other kind of variable. The variable that will be split into parts is the dividend, whereas the variable that will be divided is the divisor.
When we divide an integer by itself, we receive two numbers: the quotient and the remainder. The rules for dividing numbers using integers in C are very similar to those for using arithmetic.
When we think about division, we often see the process leading to an answer that might have a fractional component (a floating-point data type). The floating-point division is the name given to this particular kind of division.
However, when both operands are of the integer data type, a special sort of division is performed that may behave differently depending on the programming language. This type of division is referred to as Integer Division.
Let us take an example of 7/5
, where both operands are of the integer data type. The expression evaluates to 1, and there would be no fractional portion.
Again, this kind of division is known as Integer Division.
The outcome of dividing integers is itself an integer. Take, for instance, the equation 7/4
, which evaluates to the value 1, and 17/5
, which evaluates to the value 3, respectively.
The remainder operator, denoted by %,
is available in C and returns the value obtained after integer division. The residual operator is a kind of integer operator that can only be used for integer operands.
After dividing x
by y
, the result returned by the equation x % y
is the residual. Therefore, 7 % 4
results in 3, whereas 17 % 5
results in 2.
Rules for Integer and Float Division in C
integer / integer = integer
float / integer = float
integer / float = float
Divide Integer by an Integer Value in C
The following example of a program divides an integer value by another integer value, which produces an integer value as the final output.
#include <math.h>
#include <stdio.h>
int main() {
int a = 3;
int b = 15;
int result;
result = (b / a);
printf("%d", result);
return 0;
}
Output:
5
Divide Float by an Integer Value in C
The following example code divides a float value by an integer value, which produces a float value as the final output.
#include <math.h>
#include <stdio.h>
int main() {
int a = 3;
float b = 15.50;
double result;
result = (b / a);
printf("%f", result);
return 0;
}
Output:
5.166667
Divide Integer by a Float Value in C
The following example code divides an integer value by a float value, which produces a float value as the final output.
#include <math.h>
#include <stdio.h>
int main() {
int a = 15;
float b = 3.2;
double result;
result = (a / b);
printf("%f", result);
return 0;
}
Output:
4.687500
Integer Division and the Modulus Operator in C
It’s the second half of the solution to the question about dividing integers.
The %
symbol denotes this operator; its proper name is the percentile
operator. The modulus operator is a new addition to the arithmetic operators in C, and it may function with two different operands at the same time.
To determine a result, it divides the numerator by the provided denominator. To put it another way, it results in the existence of a remainder after the integer division.
Because of this, the remainder is always and only ever an integer number. If no remainder is left, the remainder will equal zero (0).
Calculate the Quotient and Remainder by Integer Division
To begin, we will need to create four variables inside the main()
function of the datatype int and give them the names dividend
, divisor
, quotient
, and remainder.
int main() {
int dividend;
int divisor;
int quotient;
int remainder;
}
Now we will display a message to the user, asking them to enter two values, the dividend
and the divisor
, and then save those values in the variables that correspond to them.
printf("Enter dividend: ");
scanf("%d", ÷nd);
printf("Enter divisor: ");
scanf("%d", &divisor);
Calculate the quotient by dividing the dividend
by divisor
. Also, calculate the remainder by dividend
modulus divisor
.
quotient = dividend / divisor;
remainder = dividend % divisor;
In the last step, we will need to output the quotient and the remainder we just computed on the console.
printf("Quotient = %d\n", quotient);
printf("Remainder = %d", remainder);
Complete Source Code:
#include <stdio.h>
int main() {
int dividend;
int divisor;
int quotient;
int remainder;
printf("Enter dividend: ");
scanf("%d", ÷nd);
printf("Enter divisor: ");
scanf("%d", &divisor);
quotient = dividend / divisor;
remainder = dividend % divisor;
printf("Quotient = %d\n", quotient);
printf("Remainder = %d", remainder);
return 0;
}
Output:
Enter dividend: 500
Enter divisor: 24
Quotient = 20
Remainder = 20
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedInRelated Article - C Integer
- Difference Between Unsigned Int and Signed Int in C
- How to Convert Char* to Int in C
- How to Convert a String to Integer in C
- How to Convert Integer to Char in C
- How to Convert an Integer to a String in C