The Round Function in C
- Understanding the Round Function
- Basic Example of the Round Function
- Rounding Negative Numbers
- Rounding with Different Data Types
- Conclusion
- FAQ
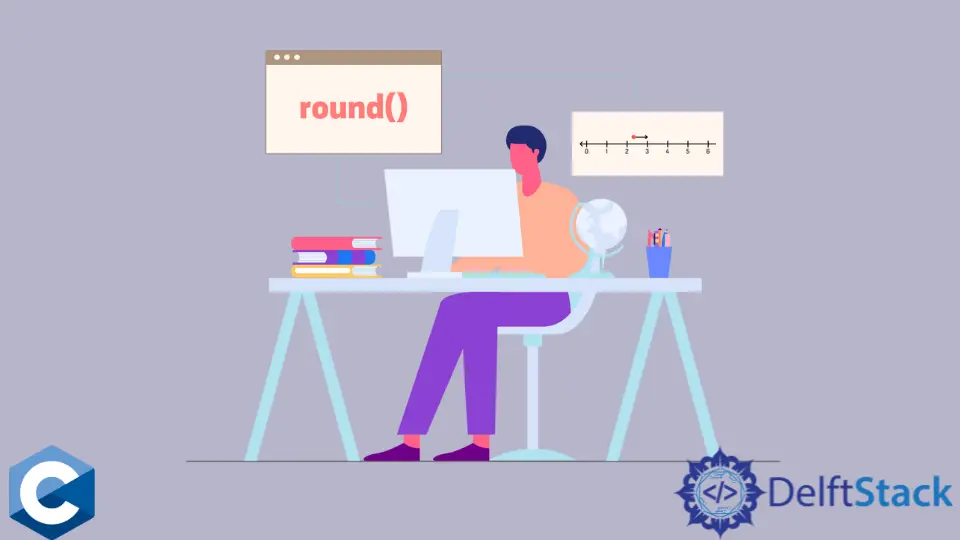
In the world of programming, dealing with numbers is a daily task. One common requirement is rounding numbers to the nearest integer. In C, the round function serves this purpose effectively. Whether you’re working on a simple calculator or a complex scientific application, understanding how to utilize the round function can enhance your code’s precision and performance.
This article will guide you through the round function in C, explaining its syntax, usage, and providing practical examples to illustrate its functionality. By the end, you’ll have a solid grasp of how to implement this function in your own projects.
Understanding the Round Function
The round function in C is part of the math library, which means you need to include the math.h header file to use it. The primary purpose of this function is to round a floating-point number to the nearest integer. If the fractional part of the number is 0.5 or greater, it rounds up to the next integer. If it’s less than 0.5, it rounds down.
The syntax for the round function is straightforward:
double round(double x);
Here, x
is the floating-point number you want to round. The function returns the rounded value as a double. This is particularly useful when you need to ensure that your calculations yield whole numbers, especially in applications like statistical analysis or financial calculations.
Basic Example of the Round Function
To illustrate how the round function works, let’s look at a simple example where we round a few floating-point numbers.
#include <stdio.h>
#include <math.h>
int main() {
double num1 = 2.3;
double num2 = 2.5;
double num3 = 2.7;
printf("Rounded value of %.1f: %.0f\n", num1, round(num1));
printf("Rounded value of %.1f: %.0f\n", num2, round(num2));
printf("Rounded value of %.1f: %.0f\n", num3, round(num3));
return 0;
}
Output:
Rounded value of 2.3: 2
Rounded value of 2.5: 3
Rounded value of 2.7: 3
In this example, we define three double variables with different floating-point values. We then use the round function to round each of these values and print the results. As you can see, 2.3 rounds down to 2, while both 2.5 and 2.7 round up to 3. This behavior of the round function is essential for scenarios where precise rounding is crucial.
Rounding Negative Numbers
The round function also works seamlessly with negative numbers. Understanding how it behaves with negatives is essential for comprehensive number handling. Let’s explore this with a code example.
#include <stdio.h>
#include <math.h>
int main() {
double num1 = -2.3;
double num2 = -2.5;
double num3 = -2.7;
printf("Rounded value of %.1f: %.0f\n", num1, round(num1));
printf("Rounded value of %.1f: %.0f\n", num2, round(num2));
printf("Rounded value of %.1f: %.0f\n", num3, round(num3));
return 0;
}
Output:
Rounded value of -2.3: -2
Rounded value of -2.5: -3
Rounded value of -2.7: -3
Here, we are rounding negative floating-point numbers. The results show that -2.3 rounds up to -2, while both -2.5 and -2.7 round down to -3. This is a crucial aspect to remember, especially in calculations involving both positive and negative values, as it can affect the outcome of your results.
Rounding with Different Data Types
While the round function is primarily used with double data types, you can also use it with float and long double types. However, the return type will always be a double. Let’s see how this works with a float.
#include <stdio.h>
#include <math.h>
int main() {
float num = 3.6f;
printf("Rounded value of %.1f: %.0f\n", num, round(num));
return 0;
}
Output:
Rounded value of 3.6: 4
In this example, we declared a float variable and passed it to the round function. Despite being a float, the function correctly rounds it and returns a double result. This flexibility allows programmers to work with various numeric types without worrying about type conversions.
Conclusion
The round function in C is a powerful tool for managing floating-point numbers and ensuring accurate calculations. By rounding numbers to their nearest integer, you can maintain precision in your programs, whether you’re dealing with positive or negative values. Understanding how to use this function effectively can enhance your coding skills and lead to better software development practices. With the examples provided, you now have a practical understanding of how to implement the round function in your own C projects.
FAQ
-
What is the round function in C?
The round function in C rounds a floating-point number to the nearest integer. -
Do I need to include any special libraries to use the round function?
Yes, you need to include the math.h library to use the round function. -
How does the round function behave with negative numbers?
The round function rounds negative numbers towards zero, meaning -2.5 becomes -3. -
Can I use the round function with float variables?
Yes, you can use the round function with float variables, but it will return a double.
- What happens if I pass a NaN value to the round function?
If you pass a NaN value to the round function, it will return NaN.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook