The Modulo Operator in C
- What is the Modulo Operator?
- Basic Syntax of the Modulo Operator in C
- Using the Modulo Operator to Check Even or Odd Numbers
-
Use
%
Modulo Operator to Implement Leap Year Checking Function in C -
Use
%
Modulo Operator to Generate Random Numbers in the Given Integer Range in C - The Modulo Operator in Looping Structures
- Conclusion
- FAQ
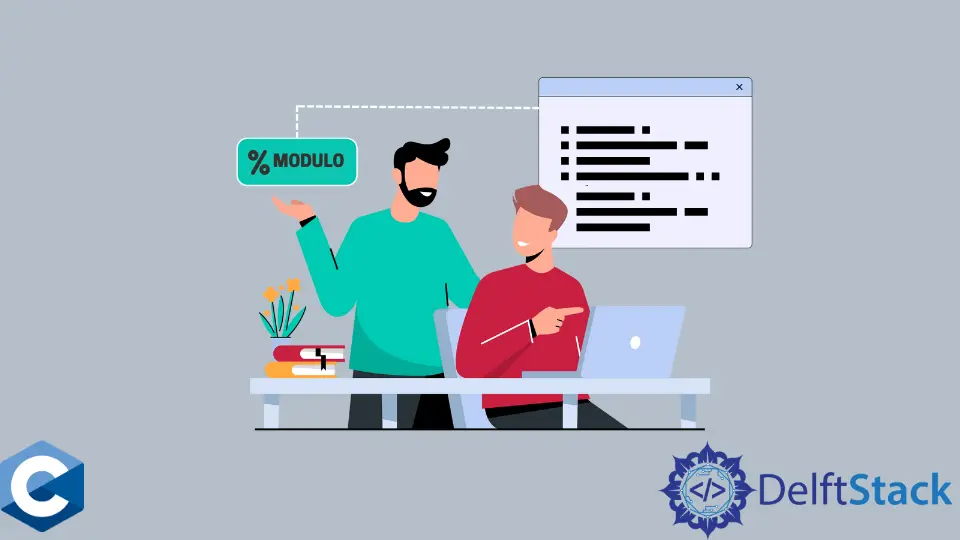
The modulo operator, often represented by the percentage symbol (%), is a fundamental tool in C programming. It allows developers to determine the remainder of a division operation between two integers. Understanding how to effectively use the modulo operator can enhance your programming skills, particularly when dealing with loops, conditional statements, and algorithms that require even or odd number checks.
In this article, we will explore how to use the modulo operator in C, providing clear examples and explanations to help you grasp this essential concept. Whether you’re a beginner or looking to refresh your knowledge, this guide will serve as an invaluable resource.
What is the Modulo Operator?
The modulo operator is a mathematical operator that returns the remainder of a division operation. For example, using the expression a % b
, where a
and b
are integers, the result will be the remainder when a
is divided by b
. If a
is 10 and b
is 3, the expression evaluates to 1 because 10 divided by 3 equals 3 with a remainder of 1.
In C, the modulo operator is particularly useful for various programming tasks. It can help in determining whether a number is even or odd, cycling through arrays, or controlling loops.
Basic Syntax of the Modulo Operator in C
The syntax for using the modulo operator in C is straightforward:
int result = a % b;
Here, result
will store the remainder after dividing a
by b
. It’s important to note that both a
and b
must be integers. Using the modulo operator with floating-point numbers will lead to a compilation error.
Example of Basic Modulo Operation
Here’s a simple example that demonstrates the basic use of the modulo operator in C:
#include <stdio.h>
int main() {
int a = 10;
int b = 3;
int result = a % b;
printf("The result of %d %% %d is: %d\n", a, b, result);
return 0;
}
Output:
The result of 10 % 3 is: 1
In this example, we declare two integers, a
and b
, and calculate the remainder of a
divided by b
. The result is printed to the console.
Using the Modulo Operator to Check Even or Odd Numbers
One of the most common applications of the modulo operator is to check whether a number is even or odd. The logic is simple: if a number modulo 2 equals 0, it is even; otherwise, it is odd.
Example of Even or Odd Check
Here’s how you can implement this logic in C:
#include <stdio.h>
int main() {
int number;
printf("Enter an integer: ");
scanf("%d", &number);
if (number % 2 == 0) {
printf("%d is an even number.\n", number);
} else {
printf("%d is an odd number.\n", number);
}
return 0;
}
Output:
Enter an integer: 5
5 is an odd number.
In this code, we prompt the user to enter an integer. We then use the modulo operator to determine if the number is even or odd and print the appropriate message. This simple program illustrates how the modulo operator can be employed in decision-making processes.
Use %
Modulo Operator to Implement Leap Year Checking Function in C
Alternatively, we can use %
operator to implement more complex functions. The next example code demonstrates isLeapYear
boolean function that checks if the given year is a leap or not. Note that a year is considered a leap year if its value is divisible by 4 but is not divisible by 100. Additionally, if the year value is divisible by 400, it’s a leap year.
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
bool isLeapYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0)
return true;
else
return false;
}
int main(void) {
uint year = 2021;
isLeapYear(year) ? printf("%d is leap\n", year)
: printf("%d is not leap\n", year);
exit(EXIT_SUCCESS);
}
Output:
2021 is not leap
Use %
Modulo Operator to Generate Random Numbers in the Given Integer Range in C
Another useful feature of the modulo operator is to limit the upper floor of numbers during the random number generation process.
Suppose we have a function that generates the random integer. In that case, we can take the remainder of the division between the returned number and the value we need to be the maximum (defined as MAX
macro in the following example).
Note that using srand
and rand
functions for random number generation is not a robust method, and applications requiring quality random numbers should employ other facilities.
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define COUNT 10
#define MAX 100
int main(void) {
srand(time(NULL));
for (int i = 0; i < COUNT; i++) {
printf("%d, ", rand() % MAX);
}
printf("\b\b \n");
exit(EXIT_SUCCESS);
}
Output:
3, 76, 74, 93, 51, 65, 76, 31, 61, 97
The Modulo Operator in Looping Structures
The modulo operator is also useful in looping structures, especially when you want to perform an action at regular intervals. For instance, you might want to print a message every third iteration of a loop.
Example of Using Modulo in a Loop
Here’s an example that demonstrates this concept:
#include <stdio.h>
int main() {
for (int i = 1; i <= 10; i++) {
if (i % 3 == 0) {
printf("This is iteration number %d.\n", i);
}
}
return 0;
}
Output:
This is iteration number 3.
This is iteration number 6.
This is iteration number 9.
In this example, we loop through the numbers 1 to 10. The program checks if the current iteration number is divisible by 3 using the modulo operator. If true, it prints a message. This technique is particularly useful in scenarios where you need to execute specific actions at regular intervals.
Conclusion
The modulo operator is a powerful tool in C programming that allows you to perform various tasks, from checking the parity of numbers to controlling the flow of loops. Understanding how to use the modulo operator effectively can greatly enhance your programming capabilities. Whether you’re developing algorithms, managing data, or creating user interactions, mastering this operator is essential for any C programmer. With the examples provided in this article, you should now have a solid foundation to start using the modulo operator in your own projects.
FAQ
-
What does the modulo operator do in C?
The modulo operator returns the remainder of a division operation between two integers. -
Can the modulo operator be used with floating-point numbers in C?
No, the modulo operator can only be used with integers in C. -
How can I check if a number is even or odd using the modulo operator?
You can check if a number is even by usingnumber % 2 == 0
. If true, the number is even; otherwise, it is odd. -
Can I use the modulo operator in loops?
Yes, the modulo operator is often used in loops to perform actions at regular intervals. -
What happens if I divide by zero using the modulo operator?
Dividing by zero will result in a runtime error in C.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook