How to Convert an Integer to a String in C
-
How to Convert an Integer Into a String in C Using the
sprintf()
Function -
How to Convert an Integer Into a String in C Using the
snprintf()
Function -
How to Convert an Integer Into a String in C Using the
itoa()
Function - Conclusion
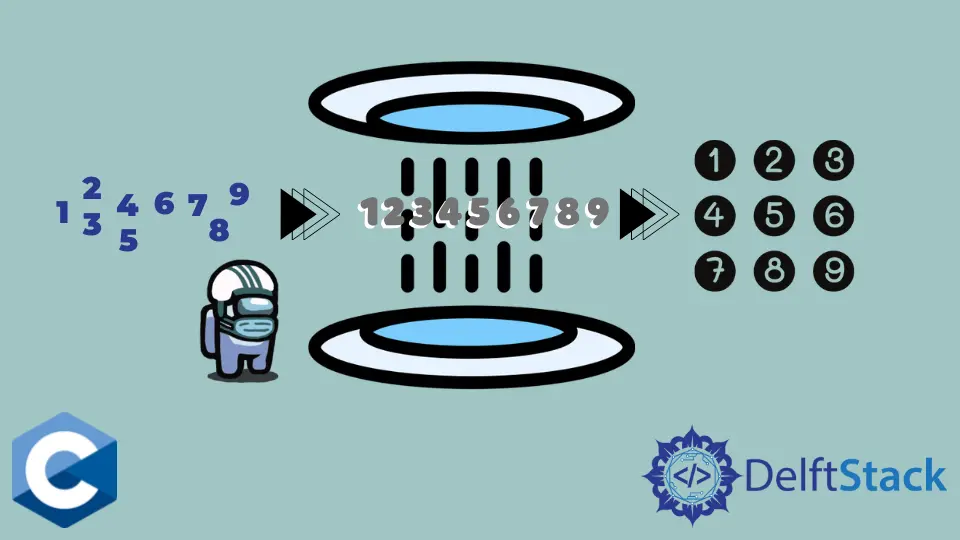
Converting an integer to a string is a fundamental operation, as it allows you to work with numerical data as text. This can be useful when you need to display numbers, log information, or construct strings that include numeric values.
Here, we will look at three common methods for this conversion: sprintf()
, snprintf()
, and itoa()
.
How to Convert an Integer Into a String in C Using the sprintf()
Function
As its name suggests, this function prints any value into a string. It gives a straightforward way to convert an integer value to a string.
This function works the same as the printf()
function, but it does not print a value directly on the console but returns a formatted string. The return value is usually discarded, but if an error occurs during the operation, it will return -1
.
Syntax of the sprintf()
Function
Here’s a general overview of the sprintf()
function:
int sprintf(char *str, const char *format, ...);
Parameters:
char *str
: This is the destination buffer where the formatted string will be stored.const char *format
: This is a format string that determines how the data should be formatted....
: These are the arguments to be formatted according to the format string.
To convert int to a string using sprintf()
, first include the necessary header:
#include <stdio.h>
Then, declare an integer variable and a character array (string) to store the converted value:
int number;
char text[20];
In this example, we’re using number
to store the integer value we want to convert and text
to store the resulting string. Make sure that text
is large enough to hold the converted string.
Use sprintf()
to perform the conversion:
sprintf(text, "%d", number);
In this line of code, we’re using sprintf()
to format and store the integer number
as a string in the text
buffer. The format specifier "%d"
is used to indicate that we are converting an integer.
You can use other format specifiers to control the output format, such as "%x"
for hexadecimal or "%f"
for floating-point numbers.
The resulting string is now stored in the text
buffer and can be used as needed.
Here’s the complete code example:
#include <stdio.h>
int main(void) {
int number;
char text[20];
printf("Enter a number: ");
scanf("%d", &number);
sprintf(text, "%d", number);
printf("\nYou have entered: %s", text);
return 0;
}
Code Output:
When you run this code, you’ll see that the integer value 123
has been successfully converted to the string "123"
.
It’s crucial to ensure that the destination buffer (text
in this case) is large enough to accommodate the converted string. If the buffer is too small, it can lead to a buffer overflow, resulting in unpredictable behavior and potentially crashing your program.
To prevent this, you can use the snprintf()
function, which allows you to specify the buffer size to avoid overflows.
How to Convert an Integer Into a String in C Using the snprintf()
Function
One of the safest and most flexible methods to convert data from one data type to another, such as converting an integer value to a string, is by using the snprintf()
function. It is designed for formatted output, similar to printf()
, but instead of sending the formatted data to the standard output (the console), it stores the result in a character array, effectively converting the data to a string.
Syntax of the snprintf()
Function
Here’s an overview of the snprintf()
function:
int snprintf(char *str, size_t size, const char *format, ...);
Parameters:
char *str
: This is the destination buffer where the formatted string will be stored.size_t size
: The maximum number of characters that can be stored in the buffer, preventing buffer overflows.const char *format
: This is the format string that determines how the data should be formatted....
: These are the arguments to be formatted according to the format string.
To convert int to a string using snprintf()
, again, include the necessary header:
#include <stdio.h>
Declare an integer variable and a character array (string) to store the converted value:
int num;
char str[20];
The int num
will store the integer value we want to convert, and str
will store the resulting string. Ensure that str
has enough space to hold the converted string.
Use snprintf()
to perform the conversion:
snprintf(str, sizeof(str), "%d", num);
In this line of code, we use snprintf()
to format and store the integer num
as a string in the str
buffer. The format specifier "%d"
is used to indicate that we are converting an integer.
You can use other format specifiers to control the output format, such as "%x"
for hexadecimal or "%f"
for floating-point numbers.
The resulting string is now stored in the str
buffer and can be used as needed.
Here’s a complete code example:
#include <stdio.h>
int main() {
int num;
char str[20];
printf("Enter a number: ");
scanf("%d", &num);
snprintf(str, sizeof(str), "%d", num);
printf("\nYou have entered: %s", str);
return 0;
}
Code Output:
When you run this code, you will see that the integer 123
has been successfully converted to the string "123"
.
One of the key advantages of using snprintf()
over sprintf()
is the ability to specify the size of the destination buffer. This method ensures that your code is safe from buffer overflows, which can lead to unpredictable behavior and program crashes.
Always provide the correct buffer size to snprintf()
to prevent such issues.
How to Convert an Integer Into a String in C Using the itoa()
Function
The itoa()
function is not a part of the C standard library, so its availability may vary depending on the C compiler and platform you’re using. Some compilers, like GCC, provide it as an extension.
itoa()
is a function in C that converts an integer to a null-terminated string. It can handle both positive and negative numbers.
The resulting string is null-terminated, meaning it ends with a null character ('\0'
) to mark the string’s termination. This ensures compatibility with C string handling functions.
Syntax of the itoa()
Function
Here’s a general representation of itoa()
:
char *itoa(int num, char *str, int base);
Parameters:
int num
: This is the integer you want to convert to a string.char *str
: This is the character array (string) where the result will be stored.int base
: The numerical base for the conversion (e.g.,10
for decimal,16
for hexadecimal).
To convert an integer to a string using itoa()
, include the necessary header:
#include <stdio.h>
Next, declare an integer variable and a character array (string) to store the converted value:
int num;
char str[20];
The num
variable will hold the integer value you want to convert, and str
will store the resulting string. Ensure that str
is large enough to accommodate the converted string.
Use itoa()
to perform the conversion:
itoa(num, str, 10);
In this line, we use itoa()
to format and store the integer num
as a string in the str
buffer. The 10
specifies the base for conversion (decimal in this case).
If you want to convert to hexadecimal, you would use 16
as the base.
The resulting string is now stored in the str
buffer and can be used as needed.
Here’s a complete code example:
#include <stdio.h>
#include <stdlib.h>
int main() {
int num;
char str[20];
printf("Enter a number: ");
scanf("%d", &num);
itoa(num, str, 10);
printf("\nYou have entered: %s", str);
return 0;
}
Code Output:
When you run this code, you will see that the given integer will be successfully converted to a string.
itoa()
function is not a part of the C standard library, and its availability depends on your compiler and platform. It is not a portable solution, so you may want to consider using standard functions like sprintf()
or snprintf()
for more portable code.Conclusion
Each of the methods discussed in this article has its advantages and considerations. The sprintf()
function is a flexible and widely available option, but you need to manage buffer size carefully.
On the other hand, the snprintf()
function is a safer alternative, allowing you to specify buffer size. Lastly, the itoa()
function is non-standard and not universally available, so it might not be suitable for all projects.
The choice of method depends on your specific needs, the portability requirements of your code, and your preferences for safety and convenience.
Building on the foundation of integer-to-string conversion, a valuable next topic you can explore is string manipulation, which involves understanding how to work with string concatenation in C.
Related Article - C Integer
- Integer Division in C
- Difference Between Unsigned Int and Signed Int in C
- How to Convert Char* to Int in C
- How to Convert a String to Integer in C
- How to Convert Integer to Char in C