How to Concatenate Strings in C
-
Use the
strcat
andstrcpy
Functions to Concatenate Strings in C -
Use the
memccpy
Function to Concatenate Strings in C - Use the Custom Defined Function to Concatenate String Literals in C
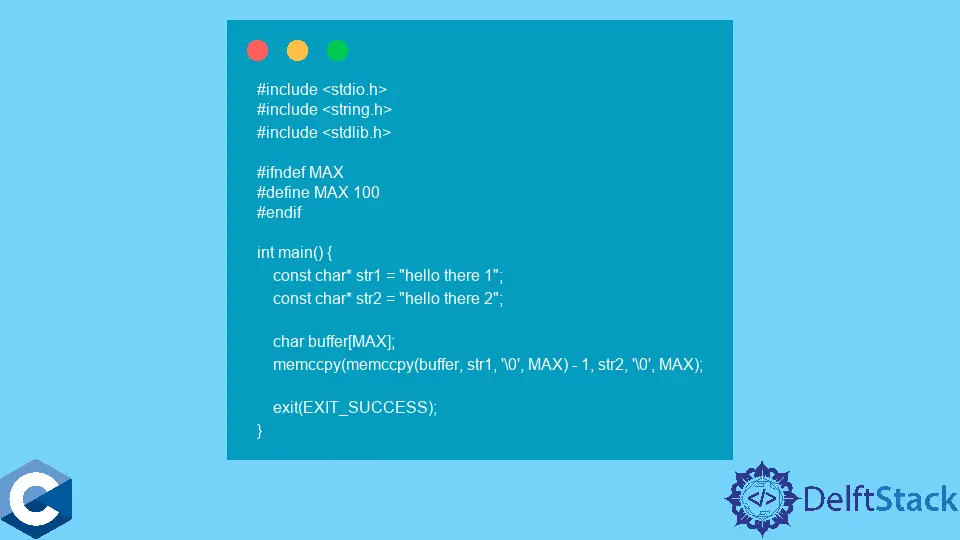
This article will explain several methods of how to concatenate strings in C.
Use the strcat
and strcpy
Functions to Concatenate Strings in C
strcat
is part of the C standard library string facilities defined in the <string.h>
header. The function takes two char*
arguments and appends the string stored at the second pointer to the one at the first pointer.
Since the C-style strings end with the \0
character, strcat
starts appending to the destination string beginning from the null char. Finally, the end of a newly constructed string is ended with the \0
character. Notice that the programmer is responsible for allocating the large enough memory at the destination pointer to store both strings.
The second function to be utilized in this solution is strcpy
, which similarly takes two char*
parameters and copies the string at the second pointer to the first pointer. Note that strcpy
is used to copy the first string to the designated char
buffer and then pass the destination pointer to the strcat
function.
Both functions return the pointer to the destination string, and that makes the chaining calls possible.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#ifndef MAX
#define MAX 100
#endif
int main() {
const char* str1 = "hello there 1";
const char* str2 = "hello there 2";
char buffer[MAX];
strcat(strcpy(buffer, str1), str2);
printf("%s\n", buffer);
char buffer2[MAX];
strcat(strcpy(buffer2, "Hello there, "), "Josh");
strcat(buffer2, "!\nTemporary...");
printf("%s\n", buffer2);
exit(EXIT_SUCCESS);
}
Output:
hello there 1hello there 2
Hello there, Josh!
Temporary...
Use the memccpy
Function to Concatenate Strings in C
The previous method’s biggest downside is its inefficient implementation that does redundant iterations over the first string stored by the strcpy
function.
memccpy
, on the other hand, corrects this issue and processes both strings efficiently. memccpy
copies no more than the user-specified number of bytes from the source char*
to destination pointer only stopping when the given character is found in the source string. memccpy
returns the pointer to the last stored character in the destination buffer.
Thus, two memccpy
calls can be chained similarly to the previous method. The first string is copied to the preallocated buffer by the user, and the second string is appended to the pointer returned from the first call to memccpy
.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#ifndef MAX
#define MAX 100
#endif
int main() {
const char* str1 = "hello there 1";
const char* str2 = "hello there 2";
char buffer[MAX];
memccpy(memccpy(buffer, str1, '\0', MAX) - 1, str2, '\0', MAX);
exit(EXIT_SUCCESS);
}
Output:
hello there 1hello there 2
Use the Custom Defined Function to Concatenate String Literals in C
Alternatively, if the memccpy
is not available on your platform, you can define a custom function implementing the same routine. concatStrings
is the example implementation that copies the one characters from one pointer to another one until the specified character is found. Note that we specify null byte \0
as the character to stop at in both examples.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#ifndef MAX
#define MAX 100
#endif
void* concatStrings(void* restrict dst, const void* restrict src, int c,
size_t n) {
const char* s = src;
for (char* ret = dst; n; ++ret, ++s, --n) {
*ret = *s;
if ((unsigned char)*ret == (unsigned char)c) return ret + 1;
}
return 0;
}
int main() {
const char* str1 = "hello there 1";
const char* str2 = "hello there 2";
char buffer[MAX];
concatStrings(concatStrings(buffer, str1, '\0', MAX) - 1, str2, '\0', MAX);
printf("%s\n", buffer);
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook