How to Split String Into Array in JavaScript
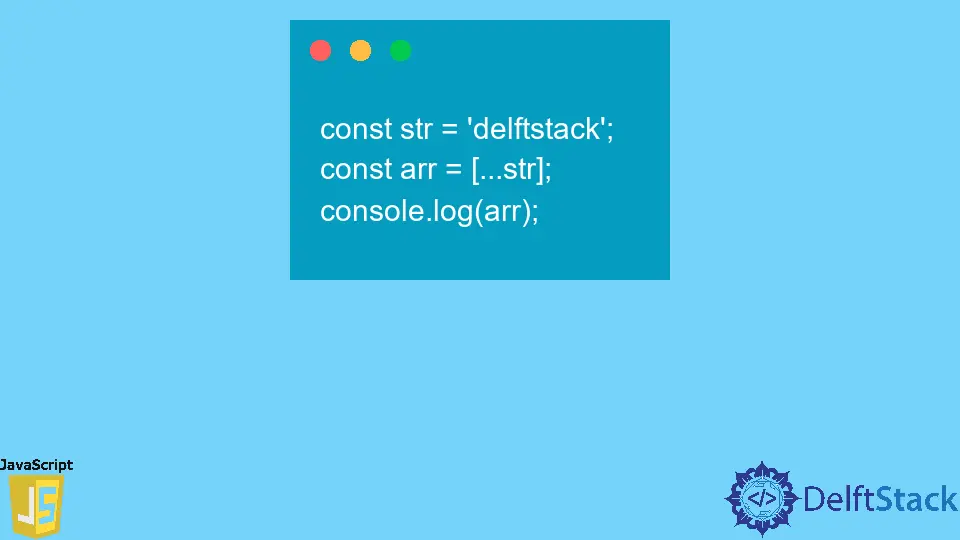
Splitting a string into an array is a fundamental skill for any JavaScript developer. Whether you’re working with user input, processing data from APIs, or manipulating text, knowing how to effectively break down strings can enhance your programming toolkit.
In this tutorial, we will explore various methods to split strings into arrays in JavaScript. You’ll learn about the built-in split()
method, regular expressions, and how to handle different delimiters. By the end of this article, you’ll have a solid understanding of how to manipulate strings in JavaScript, making your coding experience smoother and more efficient.
Using the split()
Method
The simplest and most common way to split a string into an array in JavaScript is by using the split()
method. This method divides a string into an array of substrings based on a specified separator.
Here’s a straightforward example:
const str = "JavaScript is fun";
const arr = str.split(" ");
console.log(arr);
Output:
["JavaScript", "is", "fun"]
In this code snippet, we first define a string str
containing the phrase “JavaScript is fun”. We then call the split()
method on this string, passing a space character as the argument. This tells JavaScript to break the string wherever it finds a space. The result is an array containing three elements: “JavaScript”, “is”, and “fun”.
The split()
method is versatile; you can use it with different delimiters, such as commas, hyphens, or even regular expressions. For instance, if you wanted to split a string by a comma, you would simply change the argument to ","
. This flexibility makes split()
a powerful tool for string manipulation in JavaScript.
Splitting with Regular Expressions
While the split()
method is effective for simple cases, sometimes you need more control over the splitting process. This is where regular expressions come in handy. Regular expressions allow you to specify complex patterns for splitting strings.
Here’s an example of using regular expressions with the split()
method:
const str = "apple, orange; banana|grape";
const arr = str.split(/[,;|]/);
console.log(arr);
Output:
["apple", " orange", " banana", "grape"]
In this case, we have a string containing various fruits separated by commas, semicolons, and pipes. By using the regular expression /[,;|]/
, we instruct JavaScript to split the string at any of these characters. The result is an array of fruits, demonstrating the power of regular expressions in string manipulation.
One important thing to note is that the resulting array may contain leading spaces. You can use the map()
function to trim these spaces if necessary. This method provides a robust way to split strings based on multiple delimiters, making it ideal for more complex string formats.
Handling Edge Cases
When splitting strings, it’s crucial to consider edge cases, such as empty strings or strings without the specified delimiter. Handling these scenarios gracefully can prevent potential errors in your code.
Here’s how you can manage edge cases:
const str1 = "";
const arr1 = str1.split(",");
console.log(arr1);
const str2 = "Hello World";
const arr2 = str2.split(";");
console.log(arr2);
Output:
[""]
["Hello World"]
In the first example, we attempt to split an empty string. The result is an array containing a single empty string element. In the second example, we try to split a string that does not contain the specified delimiter (a semicolon). The output is the original string encapsulated in an array.
To handle these edge cases effectively, you might want to check if the string is empty before attempting to split it. This can help you avoid unexpected results and ensure your application behaves as intended.
Conclusion
Splitting strings into arrays in JavaScript is an essential skill that every developer should master. Whether you use the built-in split()
method, employ regular expressions for more complex scenarios, or handle edge cases, understanding these techniques will enhance your ability to manipulate text effectively. As you continue to explore JavaScript, remember that string manipulation is a powerful tool in your programming arsenal. With practice, you’ll become more adept at handling strings, making your code cleaner and more efficient.
FAQ
- What is the
split()
method in JavaScript?
Thesplit()
method in JavaScript is used to divide a string into an array of substrings based on a specified separator.
-
Can I use multiple delimiters with the
split()
method?
Yes, by using regular expressions, you can specify multiple delimiters for splitting a string. -
What happens if I split an empty string?
If you split an empty string, the result will be an array containing a single empty string element. -
How do I remove leading or trailing spaces after splitting a string?
You can use themap()
function to trim spaces from each element in the resulting array. -
Is it possible to split a string without using any delimiters?
Yes, if you call split() without any arguments, it will return an array containing the original string as its only element.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript