How to Convert Hex String to Int in Python
-
Use
int()
to Convert Hex to Int in Python - Convert Non-Prefixed Hex String to Int in Python
- Convert Prefixed Hex String to Int in Python
- Convert Little and Big Endian Hex String to Int in Python
- Convert Hex to Signed Integer in Python
- Conclusion
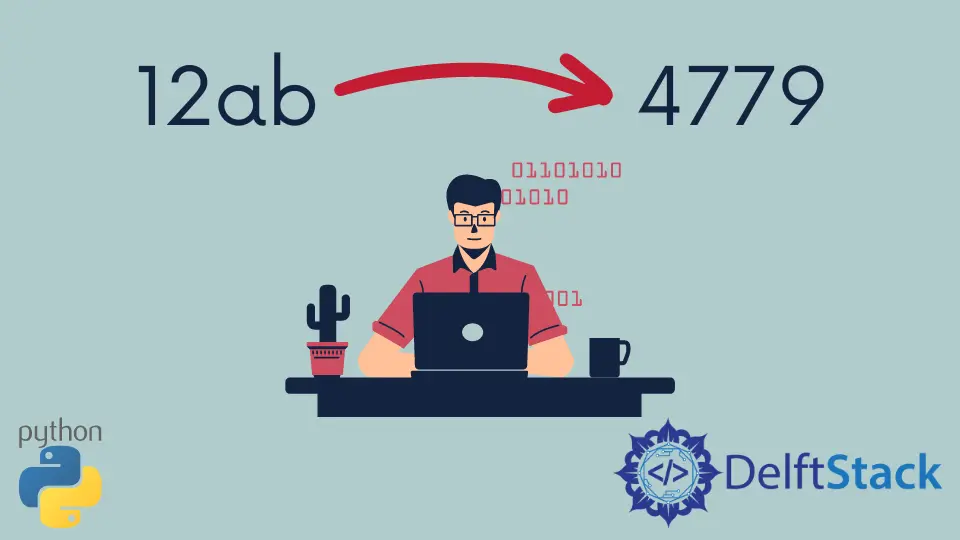
Hexadecimal representation is a fundamental format for expressing binary data in a human-readable form, often encountered in various programming scenarios. In Python, the need to convert hex strings into integers is common, playing a crucial role in low-level data processing, interfacing with hardware, and handling diverse byte order formats.
This tutorial will demonstrate how to convert the hex string to int
in Python. It will cover different hex formats like signed, little, and big-endian, 0x
annotated hexadecimal, and the default hex string.
Use int()
to Convert Hex to Int in Python
The most common and effective way to convert a hex into an integer in Python is to use the type-casting function int()
.
This function accepts two arguments: one mandatory argument, which is the value to be converted, and a second optional argument, which is the base of the number format with the default as 10
.
Other number formats are 2
for binary, 8
for octal, and 16
for hexadecimal.
If you put 0
as the argument for the base value, it will derive the number format from the value’s prefix. If there isn’t any prefix, it will automatically recognize it as a decimal, 0b
for binary, 0o
for octal, and 0x
for hexadecimal.
Convert Non-Prefixed Hex String to Int in Python
The term “non-prefixed” refers to hex strings without the 0x
or 0X
prefix commonly seen in hexadecimal literals. The process is essential for scenarios where hex values are received or stored without any prefix.
Let’s dive into the code to understand how to perform this conversion.
# Replace this hex string with your own
hex_string = "1a"
# Convert the non-prefixed hex string to an integer
integer_value = int(hex_string, 16)
# Display the result
print(f"The integer value of the hex string '{hex_string}' is: {integer_value}")
The variable hex_string
is defined with the hex value that you want to convert to an integer. In this example, it’s set to 1a
.
The int()
function is then used to convert the hex string to an integer. The function takes two arguments - the first is the hex string, and the second is the base, which is set to 16
for hexadecimal.
Finally, the result is displayed using the print()
function. It prints a formatted string that includes both the original hex string and the corresponding integer value.
Output:
The integer value of the hex string '1a' is: 26
Convert Prefixed Hex String to Int in Python
When working with hex values in Python, you may encounter prefixed hex strings, denoted by the 0x
or 0X
at the beginning. Understanding how to convert such prefixed hex strings to integers is essential for various programming tasks.
Let’s delve into the code to grasp the process of converting a prefixed hex string to an integer.
# Replace this hex string with your own (prefixed with '0x' or '0X')
hex_string = "0x1a"
# Convert the prefixed hex string to an integer
integer_value = int(hex_string, 0)
# Display the result
print(f"The integer value of the hex string '{hex_string}' is: {integer_value}")
Firstly, the variable hex_string
is defined with the prefixed hex value. In this example, it’s set to 0x1a
.
The 0x
prefix indicates that the following characters represent a hexadecimal number.
Next, the int()
function is employed for the conversion. The function takes two arguments: the hex string and the base.
In this case, the base is set to 0, which allows the function to auto-detect the base from the prefix. If 0x
or 0X
is present, it will interpret the string as hexadecimal.
Finally, the result is displayed using the print()
function. The output includes both the original hex string and the corresponding integer value.
Output:
The integer value of the hex string '0x1a' is: 26
Convert Little and Big Endian Hex String to Int in Python
Little-endian and big-endian byte orders are two types of ordering systems for hexadecimal. The default order is little-endian, which puts the most significant number in the right-most part of the sequence, while the big-endian does the opposite.
With that in mind, all we have to consider is to convert the big-endian hexadecimal value into a little-endian. Afterward, the usual conversion can now be performed on it.
Let’s explore the code example to illustrate the conversion process.
# Replace this hex string with your own big-endian hex string
big_endian = "efbe"
# Function to convert big-endian hex string to little-endian hex
def to_little(val):
# Convert the big-endian hex string to a byte array
little_hex = bytearray.fromhex(val)
# Reverse the order of the byte array
little_hex.reverse()
print("Byte array format:", little_hex)
# Convert the reversed byte array back to a hex string
str_little = "".join(format(x, "02x") for x in little_hex)
return str_little
# Convert big-endian hex string to little-endian hex
little_endian = to_little(big_endian)
# Display the results
print("Little endian hex:", little_endian)
print("Hex to int:", int(little_endian, 16))
The variable big_endian
is initialized with the big-endian hex value efbe
, which we aim to convert to a little-endian hex.
The to_little
function is defined to perform the conversion. It starts by converting the big-endian hex string into a byte array using bytearray.fromhex(val)
.
The byte order is reversed using the reverse()
method on the byte array. This step is crucial for the conversion to a little-endian format.
The reversed byte array is then converted back to a little-endian hex string using list comprehension and join()
.
The final little-endian hex string and its corresponding integer value are printed using the print()
function.
Output:
Byte array format: bytearray(b'\xbe\xef')
Little endian hex: beef
Hex to int: 48879
Convert Hex to Signed Integer in Python
Hexadecimal notation is frequently used to represent binary data in a more human-readable form. However, interpreting hexadecimal values as signed integers requires consideration of the two’s complement representation, a concept fundamental to handling negative numbers in binary systems.
Let’s explore the code example to illustrate the conversion process.
# Hexadecimal Input
hex_val = "ff"
# Two's Complement Function
def twosComplement_hex(hexval):
bits = 16
val = int(hexval, bits)
if val & (1 << (bits - 1)):
val -= 1 << bits
return val
# Display Result
print(twosComplement_hex(hex_val))
The variable hex_val
is initialized with the hexadecimal value ff,
which we aim to convert to a signed integer.
The function twosComplement_hex
is defined to perform the conversion. It takes a hex value as input and returns the corresponding signed integer.
The variable bits
is set to 16, indicating the number of bits in the representation.
Inside the function, the int()
function is used to convert the hex string to an integer, specifying the base as 16.
The code checks if the most significant bit (MSB) is set, indicating a negative number in two’s complement. This is done using the bitwise AND operation with (1 << (bits - 1))
.
If the MSB is set, indicating a negative value, the code adjusts it to the correct negative value using the two’s complement method: subtracting 1 << bits
.
The final signed integer value is printed using print(twosComplement_hex(hex_val))
.
Output:
255
Conclusion
Handling hex strings in Python, whether prefixed or non-prefixed, little-endian or big-endian, and converting them to signed integers is an essential aspect of programming. This tutorial has provided a comprehensive understanding of various conversion methods, ensuring that whether you are a beginner or an experienced programmer, you can confidently work with hexadecimal data.
Mastering these techniques is key to building robust and efficient Python applications, especially in scenarios involving low-level data processing, interfacing with hardware, and diverse byte order formats.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python Hex
- How to Convert Hex to Base64 in Python
- Bitwise XOR of Hex Numbers in Python
- How to Convert Binary to Hex in Python
- How to Convert HEX to RGB in Python
- How to Convert Hex to ASCII in Python
- How to Convert Hexadecimal to Decimal in Python