How to Convert Boolean Values to Integer in Python
-
Convert Boolean Values to Integer Using the
if/else
Block in Python -
Convert Boolean Values to Integer Using the
int()
Function in Python -
Convert Boolean List to Integer Using the
map()
Function in Python -
Convert
True
/False
String to Integer With theint()
Function in Python
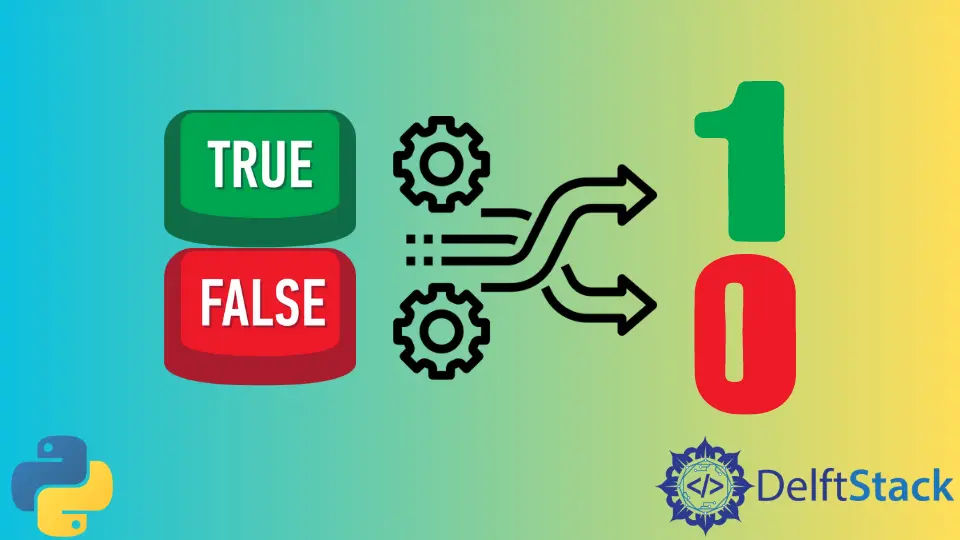
This tutorial will discuss converting boolean values to integers in Python.
Convert Boolean Values to Integer Using the if/else
Block in Python
In Python, the boolean values True
and False
correspond to integer values 1 and 0, respectively.
We can write a user-defined function that returns 1 if the value passed to the function is True
and 0 if the value passed to the function is False
.
We can do this by just the if/else
block. The following example code shows us how to convert Boolean values into 0 or 1 using the if/else
statement.
def booltoint(value):
if value == True:
return 1
else:
return 0
x = booltoint(False)
print(x)
Output:
0
Convert Boolean Values to Integer Using the int()
Function in Python
The int()
function takes the boolean value as an input and returns its equivalent integer value. We can drastically reduce the code size in the previous example with int()
.
The code snippet demonstrates the use of the int()
function to convert a boolean value into 1 or 0 in Python.
x = int(True)
y = int(False)
print(x)
print(y)
Output:
1
0
We converted the values True
and False
into integers 1 and 0, respectively, with the int()
function and stored the integer values inside variables x
and y
. We displayed the values in the output.
Convert Boolean List to Integer Using the map()
Function in Python
The previous sections discussed the methods to convert only a single boolean value to an integer value in Python. Now, we’ll see how to convert a list of boolean values into their equivalent integer values with the map()
function.
The map()
function requires a function and a list of arguments as input parameters. It applies that function on each element of the list and returns the output of that function.
The code below shows us how to convert a list of boolean values into a list of integers with the map()
function in Python.
boo = [True, False, False, True, True]
boo = list(map(int, boo))
print(boo)
Output:
[1, 0, 0, 1, 1]
We converted the list of boolean values boo
into a list of integer values by using the int()
function inside the map()
function. We enclosed the map()
function inside the list()
function to convert the output returned into a list.
In the end, we printed the newly generated list of integers.
Convert True
/False
String to Integer With the int()
Function in Python
In the previous sections, we’ve discussed the methods to convert boolean values into integer values. This section will discuss the scenario where we have to convert a string value into 1 or 0.
We can utilize the int()
function discussed in the previous sections to handle this problem.
Let’s consider an example to convert the string true
to 1 and false
to 0. We know from the previous discussions that the int()
function converts the boolean arguments into equivalent integer values.
We can convert this string into a boolean value with the ==
operator and pass it to the int()
function.
The following code demonstrates a working example of this whole process.
x = "true"
x = int(x == "true")
print(x)
Output:
1
We converted the string variable x
into its boolean equivalent integer value 1 by first converting it into a boolean expression with x == 'true'
and passing that boolean expression to the int()
function.
This method will return a False
boolean value or 0 integer value for every value of x
other than true
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python Integer
- How to Convert Int to Binary in Python
- How to Convert Roman Numerals to Integers in Python
- How to Convert Integer to Roman Numerals in Python
- Integer Programming in Python
- How to Convert String to Integer in Python