How to Convert Roman Numerals to Integers in Python
- Convert Roman Numerals to Integers in Python
- Python Program to Convert Roman Numerals to Integers in Python
- Use Python Dictionaries to Convert Roman Numerals to Integers in Python
- Use the Python Roman Module to Convert Roman Numerals to Integers in Python
- Conclusion
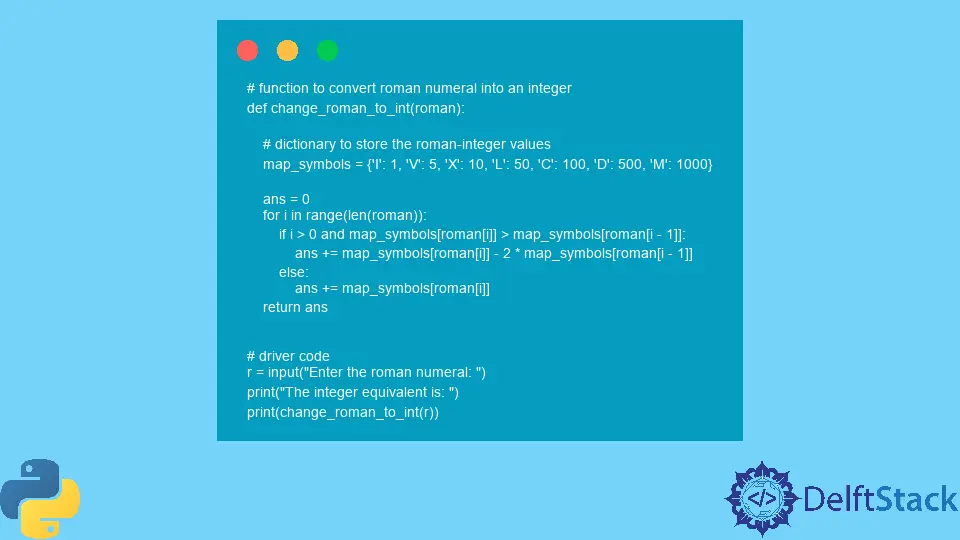
As kids, we all have learned how to convert roman numerals into integers. Now, when we are programmers, let us make a Python program that does this for us.
In this article, we will learn how to convert roman numerals to integers using Python.
Convert Roman Numerals to Integers in Python
Before we jump onto the actual code, let us revise the rules of converting roman numerals to integers. Here are the basic symbols used for certain integers:
Integer Roman Numeral
1 I
5 V
10 X
50 L
100 C
500 D
1000 M
We know that we can find the corresponding value of an integer from a given string of roman numerals. But we have to follow some rules when we do so.
-
For a given string of roman numerals, upon moving from left to right, if the values of the symbols decrease or remain the same, then we add their integer equivalents to get the final answer as an integer.
XVII X - 10 V - 5 I - 1 I - 1 10 + 5 + 1 + 1 = 17
In the above example, the values decrease from left to right. So, we add them all and get 17 as the integer value.
-
For a given string of roman numerals, upon moving from left to right, if the values of the symbols increase, then we subtract the integer equivalents of the smaller value from the bigger one to get the final answer as an integer.
XL X - 10 L - 50 50 - 10 = 40
In the above example, the values increase from left to right. So, we subtract them to get
40
as the integer value.
You need to remember these two rules while writing the code for converting a roman numeral into an integer. Also, note that apart from dictionaries, we can use a few more ways to change a roman numeral into an integer.
Let us look at them one by one.
Python Program to Convert Roman Numerals to Integers in Python
The simplest approach to converting a roman numeral into an integer is using the if
statement to store the basic roman symbols. Here is the code for the same.
# function to store the basic roman symbols
def map_symbols(s):
if s == "I":
return 1
if s == "V":
return 5
if s == "X":
return 10
if s == "L":
return 50
if s == "C":
return 100
if s == "D":
return 500
if s == "M":
return 1000
return -1
# function to perform the conversion
def change_roman_to_int(roman):
ans = 0
i = 0
while i < len(roman):
x1 = map_symbols(roman[i])
if i + 1 < len(roman):
x2 = map_symbols(roman[i + 1])
if x1 >= x2:
ans = ans + x1
i = i + 1
else:
ans = ans + x2 - x1
i = i + 2
else:
ans = ans + x1
i = i + 1
return ans
# driver code
r = input("Enter the roman numeral: ")
print("The integer equivalent is: ")
print(change_roman_to_int(r))
Output:
Enter the roman numeral: MLXVI
The integer equivalent is:
1066
Let us look at the working of this code.
When we enter a roman string as the input, it gets stored in the variable r
, which is then passed to the function change_roman_to_int
. We use a while
loop inside this function to iterate over the entire string.
Further, as the while
loop goes over each value, the if-else
statements inside the while
loop do the following:
- If the value of the current symbol is greater than or equal to the value of the next symbol, then the current value is added to the variable
ans
. - Otherwise, the value of the next symbol is added to the variable
ans
, and then the current value is subtracted from it.
This is essentially how this code works.
Let us now look at another method where we use Python dictionaries to convert roman numerals into integers.
Use Python Dictionaries to Convert Roman Numerals to Integers in Python
Here, the basic idea remains the same. The only thing that changes is that we use a dictionary to store the roman numerals and their corresponding integer values instead of the if
statement.
Here is the code for the same.
# function to convert roman numeral into an integer
def change_roman_to_int(roman):
# dictionary to store the roman-integer values
map_symbols = {"I": 1, "V": 5, "X": 10, "L": 50, "C": 100, "D": 500, "M": 1000}
ans = 0
for i in range(len(roman)):
if i > 0 and map_symbols[roman[i]] > map_symbols[roman[i - 1]]:
ans += map_symbols[roman[i]] - 2 * map_symbols[roman[i - 1]]
else:
ans += map_symbols[roman[i]]
return ans
# driver code
r = input("Enter the roman numeral: ")
print("The integer equivalent is: ")
print(change_roman_to_int(r))
Output:
Enter the roman numeral: MMMCMLXX
The integer equivalent is:
3970
Note that you can use the for
loop or the while
loop to iterate over the input string since both will work similarly.
You can also use the enumerate
function with a dictionary, as in the code below.
# function to convert roman numeral into an integer
def change_roman_to_int(roman):
# dictionary to store the roman-integer values
map_symbols = {"I": 1, "V": 5, "X": 10, "L": 50, "C": 100, "D": 500, "M": 1000}
ans = 0
l = len(roman)
for (i, v) in enumerate(roman):
if i < l - 1 and map_symbols[v] < map_symbols[roman[i + 1]]:
ans -= map_symbols[v]
else:
ans += map_symbols[v]
return ans
# driver code
r = input("Enter the roman numeral: ")
print("The integer equivalent is: ")
print(change_roman_to_int(r))
Output:
Enter the roman numeral: MCMXCIV
The integer equivalent is:
1994
We have been adding only the 7 basic roman symbols in the dictionary. We can simplify the code even more if we add more custom symbols.
Look at the code given below.
# function to change a roman numeral into an integer
def change_roman_to_int(roman):
custom_symbols = {"IV": -2, "IX": -2, "XL": -20, "XC": -20, "CD": -200, "CM": -200}
map_symbols = {"I": 1, "V": 5, "X": 10, "L": 50, "C": 100, "D": 500, "M": 1000}
ans = 0
for i in roman:
ans += map_symbols[i]
for i in range(len(roman) - 1):
merge = str(roman[i] + roman[i + 1])
if merge in custom_symbols:
ans += custom_symbols[merge]
return ans
# driver code
r = input("Enter the roman numeral: ")
print("The integer equivalent is: ")
print(change_roman_to_int(r))
Output:
Enter the roman numeral: MCMXCIV
The integer equivalent is:
1994
Are you not surprised by the cleverness of this code? Let us see how this code works.
Inside the change_roman_to_int
function, we have two dictionaries: the map_symbols
dictionary with the usual 7 values and the custom_symbols
dictionary with negative values corresponding to a pair of roman symbols.
Further, the first for
loop adds the integer values of all the roman symbols and stores the sum in the variable ans
. Here is what this step looks like:
MCMXCIV
M = 1000, C = 100, M = 1000, X = 10, C = 100, I = 1, V = 5
1000 + 100 + 1000 + 10 + 100 + 1 + 5 = 2216
ans = 2216
Then, the second for
loop iterates over the roman string, taking two symbols at a time and storing them as a string in the variable merge
. The if
statement inside this for
loop checks if the merged pair is present in the dictionary custom_symbols
.
The corresponding negative value is added to the variable ans
if found. Here is what this step looks like:
MC - Not found
CM - Found
ans = 2216 + (-200)
ans = 2016
MX - Not found
XC - Found
ans = 2016 + (-20)
ans = 1996
CI - Not found
IV - Found
ans = 1996 + (-2)
ans = 1994
This is essentially how this code works.
We have been writing all this code for so long now. Now, let us sit back and make Python do the work.
Use the Python Roman Module to Convert Roman Numerals to Integers in Python
How can someone use Python and not know that there must be a module that has functions to convert a roman numeral into an integer? Well, that module is called roman
.
To download this module, run the following command on cmd.
pip install roman
Output:
Downloading roman-3.3-py2.py3-none-any.whl (3.9 kB)
Installing collected packages: roman
Successfully installed roman-3.3
You must see the above message once the module is installed.
Now, we can import this module into our code and use the function fromRoman()
to convert any roman numeral to an integer.
import roman
x = roman.fromRoman("MCMXCIV")
print(x)
Output:
1994
Refer to this link to learn more about Python’s roman
module.
Conclusion
In this article, we looked at some very interesting ways to convert a roman numeral into an integer in Python. We used the Python if
statements and Python dictionaries to achieve this.
Also, we looked at how we can use negative values for roman pairs to make the code easier and shorter. At last, we saw the use of the roman
module of Python that uses the fromRoman()
function to convert a roman numeral into an integer.