How to Convert Integer to Roman Numerals in Python
- Convert Integers to Roman Numerals in Python
- Use Division to Convert Integers to Roman Numerals in Python
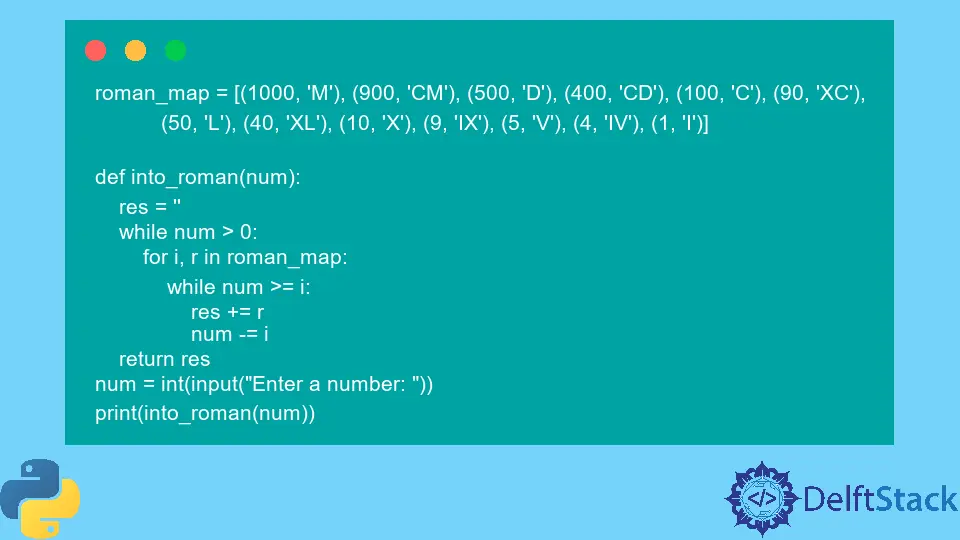
Roman numerals are written using the following seven symbols.
Symbol Value
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
These symbols are used to represent thousands of numbers.
To write 20 in Roman, you can use two X
to make XX
. But XXXX
is not equal to 40.
The Roman numeral for 40 is XL
.
Similarly:
Symbol Value
IV 4
IX 9
XL 40
XC 90
CD 400
CM 900
This tutorial will teach you to convert an integer to a Roman numeral in Python.
Convert Integers to Roman Numerals in Python
The following is an implementation of a Python program that converts a given integer into its Roman numeral equivalent.
roman_map = [
(1000, "M"),
(900, "CM"),
(500, "D"),
(400, "CD"),
(100, "C"),
(90, "XC"),
(50, "L"),
(40, "XL"),
(10, "X"),
(9, "IX"),
(5, "V"),
(4, "IV"),
(1, "I"),
]
def into_roman(num):
res = ""
while num > 0:
for i, r in roman_map:
while num >= i:
res += r
num -= i
return res
num = int(input("Enter a number: "))
print(into_roman(num))
Output:
Enter a number: 42
XLII
In the above example, roman_map
contains a list with corresponding values and symbols. res
is a blank string.
The while num > 0
runs the loop if the value of num
is greater than zero. There is no symbol for zero in Roman numerals.
The for i, r in roman_map
loops over each (integer, roman) pair until it finds the first numeral.
For a detailed visualization of the code, run the code here.
Use Division to Convert Integers to Roman Numerals in Python
The following example uses the division method to convert a user-inputted integer into a Roman numeral.
roman_map = {
1: "I",
4: "IV",
5: "V",
9: "IX",
10: "X",
40: "XL",
50: "L",
90: "XC",
100: "C",
400: "CD",
500: "D",
900: "CM",
1000: "M",
}
num = int(input("Enter a number: "))
# 13 integers in descending order
order = [1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1]
for i in order:
if num != 0:
quotient = num // i
# prints the roman numeral if the quotient is not zero
if quotient != 0:
for j in range(quotient):
print(roman_map[i], end="")
# update input number with a remainder
num = num % i
Output:
Enter a number: 42
XLII
In this approach, we divide the input integer with 13 primary integers in descending order. The roman_map
is a dictionary containing 13 integers with their Roman numerals equivalent in key:value
pairs.
The num
stores the integer entered by the user. The order
list stores 13 integers in descending order.
The input number is divided by all numbers in the order
list until the quotient is not zero.
If the quotient is not zero and there is a remainder, it is updated in a num
. The loop continues to run, and we get the Roman numeral equivalent.
Visualize the above code in this link.
Now you should know how to convert an integer to its Roman numeral equivalent in Python. We hope you find this tutorial helpful.