How to Convert Int to Binary in Python
-
Use
bin()
Function to Convert Int to Binary in Python -
Use
format
Function to Convert Int to Binary in Python -
Use the
str.format()
Method to Convert Int to Binary in Python - Use the String Formatting Method to Convert Int to Binary in Python
- Use the Bit Manipulation Method to Convert Int to Binary in Python
- Bitwise Operators in Python
- Converting Integers to Binary Using Bit Manipulation
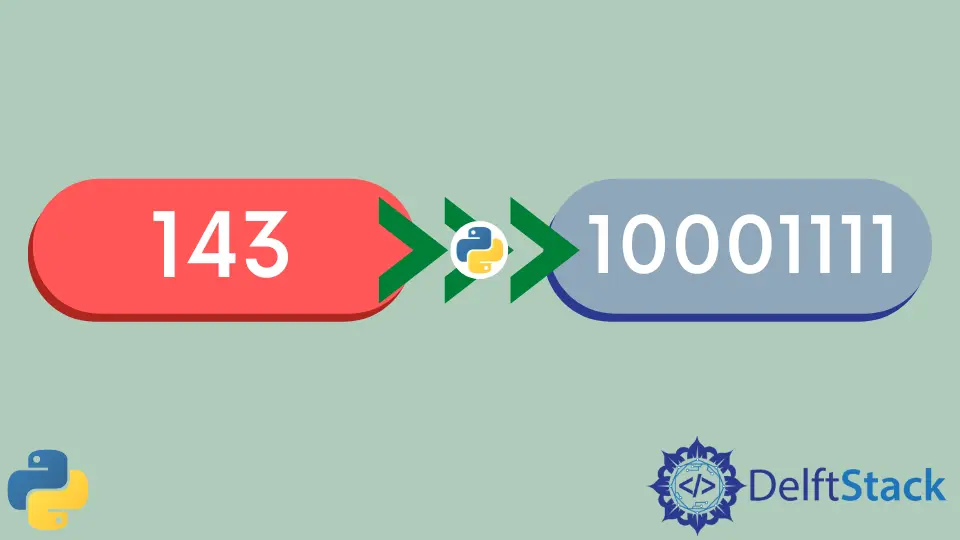
This tutorial introduces how to convert an integer to binary in Python.
This tutorial also lists some example codes to elaborate on different ways of conversion from int to binary in Python.
This article dives deep into the various techniques available in Python to achieve this conversion. We’ll explore the built-in bin()
function, the versatile format()
function, and even conver bit manipulation for those who want a hands-on approach.
Use bin()
Function to Convert Int to Binary in Python
In Python, you can use a built-in function, bin()
to convert an integer to binary. The bin()
function takes an integer as its parameter and returns its equivalent binary string prefixed with 0b
.
An example of this is:
binary = bin(16)
print(binary)
Output:
0b10000
The '0b'
prefix indicates that the string represents a binary number. While this format is suitable for internal calculations and some applications, you might want to remove the prefix when displaying the binary representation.
Use format
Function to Convert Int to Binary in Python
As shown above, the binary of an integer can be simply obtained with bin(x)
method. But if you want to remove the 0b
prefix from its output, you can use the format
function and format the output.
The format(value, format_spec)
function in Python facilitates the creation of formatted output for different types of values.
It requires two parameters: value
(the input value to be formatted) and format_spec
(the specification for formatting). By using appropriate format specifiers, you can control the appearance of the output.
To convert an integer to binary representation using the format()
function, you can make use of the '{0:b}'
format specifier.
The 0
denotes the positional argument index, and b
signifies the binary format. This approach enables you to obtain the binary representation without the 0b
prefix.
Syntax:
binary_representation = format(integer_value, "b")
Example:
decimal_number = 10
binary_representation = format(decimal_number, "b")
print(binary_representation) # Output: '1010'
In this example, the integer 10
is formatted using the '{0:b}'
format specifier, yielding the binary representation '1010'
.
format(value, format_spec)
function has two parameters - value
and format_spec
. It will return the formatted output according to the format_spec
. Below are some example formatting types that can be used inside the placeholders:
Formatting Type | Role |
---|---|
= |
Places the sign to the leftmost position |
b |
Converts the value into equivalent binary |
o |
Converts value to octal format |
x |
Converts value to Hex format |
d |
Converts the given value to decimal |
E |
Scientific format, with an E in Uppercase |
X |
Converts value to Hex format in upper case |
And there are many more formatting types available. As we want to convert int to binary, so b
formatting type will be used.
Use the str.format()
Method to Convert Int to Binary in Python
The str.format()
method is similar to the format()
function above and they share the same format_spec
.
Example code to convert int to binary using the str.format()
method is below.
temp = "{0:b}".format(15)
print(temp)
Output:
1111
Use the String Formatting Method to Convert Int to Binary in Python
Python’s string formatting capabilities offer a versatile way to convert integers to binary representation. The string formatting method allows you to control the format of the output string, making it suitable for various use cases.
The '{0:b}'
Format Specifier
To convert an integer to its binary representation using string formatting, you can use the '{0:b}'
format specifier, where {0}
is the positional argument index. The b
indicates that the argument should be presented as a binary value.
Syntax:
binary_representation = "{0:b}".format(integer_value)
Example:
decimal_number = 16
binary_representation = "{0:b}".format(decimal_number)
print(binary_representation) # Output: '10000'
Output:
'10000'
In this example, the integer 16
is formatted using the '{0:b}'
format specifier, resulting in the binary representation '10000'
.
Formatting Options
Python’s string formatting allows for additional formatting options to customize the output. For instance, you can control the width, precision, and alignment of the output. Here’s an example that demonstrates some formatting options:
decimal_number = 10
binary_representation = "{0:08b}".format(decimal_number)
print(binary_representation)
Output:
'00001010'
In this example, the ':08b'
format specifier ensures that the output is 8 characters wide, with leading zeros.
Using F-Strings (Python 3.6+)
If you’re using Python 3.6 or later, you can take advantage of f-strings for concise and readable code:
decimal_number = 5
binary_representation = f"{decimal_number:b}"
print(binary_representation)
Output:
'101'
F-strings make the code more intuitive by embedding expressions directly within the string.
Use the Bit Manipulation Method to Convert Int to Binary in Python
Bit manipulation involves operating on individual bits within binary representation. In Python, integers are stored in binary format, consisting of multiple bits.
Bit manipulation allows you to perform operations such as setting, clearing, toggling, and checking specific bits within an integer. By using bitwise operators, you can manipulate these bits to achieve desired outcomes.
Bitwise Operators in Python
Python provides several bitwise operators that facilitate bit manipulation:
&
(Bitwise AND): Performs a bitwise AND operation on corresponding bits of two integers.|
(Bitwise OR): Performs a bitwise OR operation on corresponding bits of two integers.^
(Bitwise XOR): Performs a bitwise XOR (exclusive OR) operation on corresponding bits of two integers.~
(Bitwise NOT): Inverts the bits of an integer, changing each0
to1
and vice versa.<<
(Left Shift): Shifts the bits of an integer to the left by a specified number of positions.>>
(Right Shift): Shifts the bits of an integer to the right by a specified number of positions.
Converting Integers to Binary Using Bit Manipulation
To convert an integer to its binary representation using bit manipulation, you can iterate through each bit position, extract the corresponding bit, and append it to a string. Starting from the least significant bit (LSB), you can use the right shift operator >>
to isolate each bit and the bitwise AND operator &
with 1
to extract the value of the bit.
Algorithm:
- Initialize an empty string to store the binary representation.
- Iterate through the bit positions of the integer from LSB to MSB.
- Extract the bit at the current position using the bitwise AND operator
&
with1
. - Append the extracted bit to the binary string.
- Right-shift the integer by one position using the right shift operator
>>
.
Example:
def int_to_binary(n):
binary = ""
while n > 0:
bit = n & 1
binary = str(bit) + binary
n >>= 1
return binary if binary else "0"
decimal_value = 15
binary_representation = int_to_binary(decimal_value)
print(binary_representation)
Output:
'1111'
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedInRelated Article - Python Integer
- How to Convert Roman Numerals to Integers in Python
- How to Convert Integer to Roman Numerals in Python
- Integer Programming in Python
- How to Convert Boolean Values to Integer in Python
- How to Convert String to Integer in Python