How to Convert String to Boolean in Python
-
Use the
bool()
Function to Convert String to Boolean in Python -
Use the
distutils.util.strtobool()
Function to Convert String to Boolean in Python - Use the List Comprehension to Convert String to Boolean in Python
-
Use the
map()
and Lamda Function to Convert String to Boolean in Python - Use the JSON Parser to Convert String to Boolean in Python
-
Use the
eval()
Function to Convert String to Boolean in Python
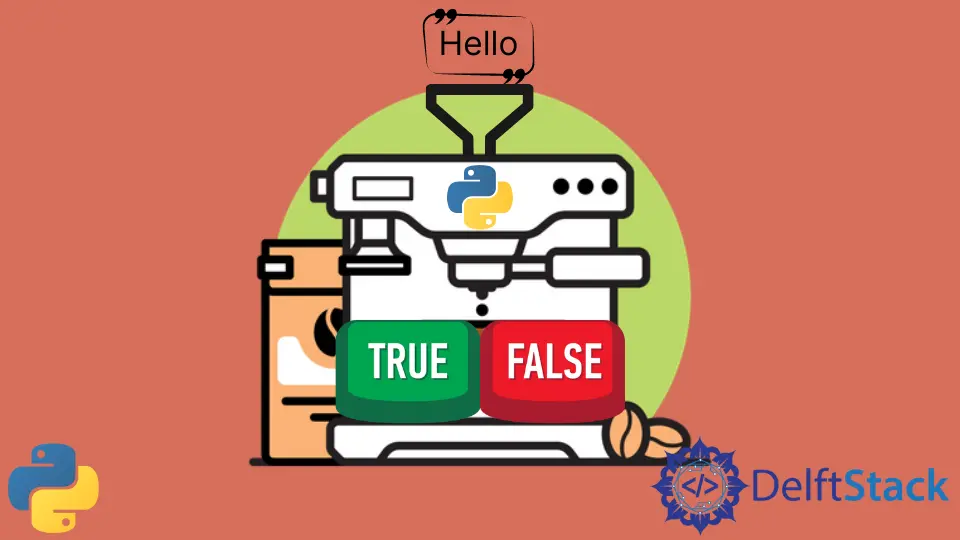
In the world of programming, there are many conversions of data types that programmers have to make that best suit their problem statement. One of those data types is the boolean data type with two possible values, true or false.
This tutorial will introduce different ways to convert a string to a boolean value in Python.
Use the bool()
Function to Convert String to Boolean in Python
We can pass a string as the argument of the function to convert the string to a boolean value. This function returns true for every non-empty argument and false for empty arguments.
Example 1:
string_value = "Hello"
boolean_value = bool(string_value)
print(boolean_value)
Output:
True
Example 2:
string_value = ""
boolean_value = bool(string_value)
print(boolean_value)
Output:
False
Use the distutils.util.strtobool()
Function to Convert String to Boolean in Python
This function converts the string value to 1 or 0. It depends on whether the value is positive or negative. Positive values like True
, Yes
, and On
are converted to 1, and negative values like False
, No
and Off
are converted into 0.
Example:
String_value = distutils.util.strtobool("Yes")
print(String_value)
Output:
1
To convert the output to a boolean, use the bool()
function.
Boolean_value = bool(String_value)
Output:
True
Use the List Comprehension to Convert String to Boolean in Python
In this method, only one value, either true or false is checked; the other value automatically falls under the opposite of what’s been checked.
Example:
String_list = ["False", "True", "False", "False", "True"]
print(str(String_list))
boolean_list = [ele == "True" for ele in String_list]
print(str(boolean_list))
Here, false values are checked, and those non-false values fall under true.
Use the map()
and Lamda Function to Convert String to Boolean in Python
The map()
function is used when a transformation function is applied to each item in an iteration and a new iteration is formed after the transformation.
Lambda function is an anonymous function in Python. Whenever an anonymous function is declared in a program, we use the keyword lambda
.
Example:
String_list = ["False", "True", "False", "False", "True"]
print(str(String_list))
Boolean_list = list(map(lambda ele: ele == "True", String_list))
print(str(Boolean_list))
Output:
[False, True, False, False, True]
We’ve also taken list comprehension as the main approach, but the difference here is that we’ve used the map()
function to make a list and have made an anonymous function to check the String_list.
Use the JSON Parser to Convert String to Boolean in Python
The JSON Parser is also useful for the basic conversion of strings to python data types. Parsing of a JSON string is done with the help of the json.loads()
method.
Example:
import json
json.loads("true".lower())
Output:
True
Also, this can be carried out using only the lower case i.e .lower()
and not the upper case.
Use the eval()
Function to Convert String to Boolean in Python
If the given string is either True
or False
, eval()
function can also be used. eval()
function examines the given string. If the string is legal, it will be executed; otherwise, it won’t be executed.
Example:
eval("False")
Output:
False
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn