How to Convert Hexadecimal to Decimal in Python
-
Method 1: Using the Built-in
int()
Function -
Method 2: Using
hex()
andord()
Functions - Method 3: Using a Custom Function
- Conclusion
- FAQ
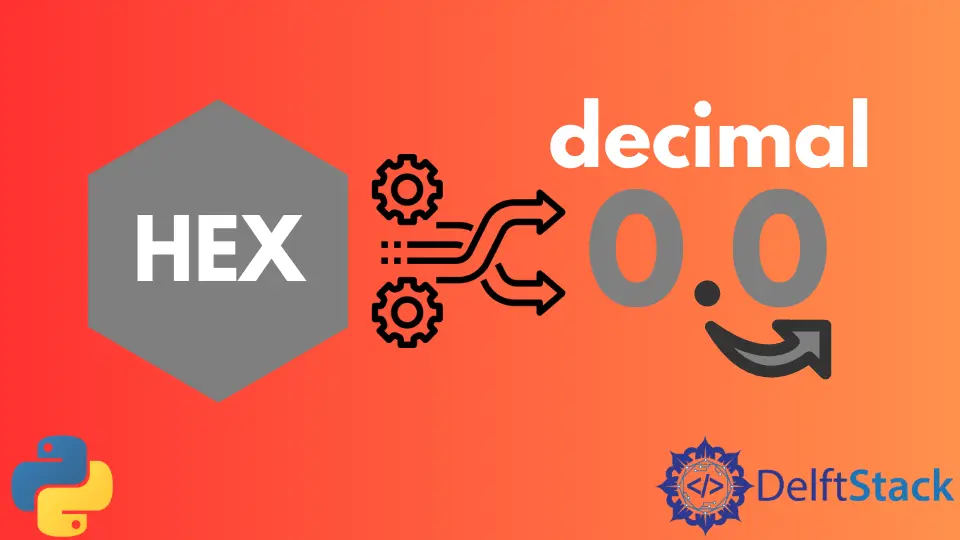
Converting hexadecimal values to decimal is a common task in programming, especially when dealing with color codes, memory addresses, or low-level data manipulation. Python makes this process straightforward and efficient.
In this tutorial, we’ll explore several methods to convert hexadecimal values to decimal in Python, ensuring you have the tools and knowledge to handle any situation that arises. Whether you’re a beginner or an experienced programmer, understanding how to perform this conversion will enhance your coding skills. Let’s dive into the world of hexadecimal and decimal conversions in Python!
Method 1: Using the Built-in int()
Function
One of the simplest ways to convert a hexadecimal string to a decimal integer in Python is by using the built-in int()
function. This function can take two arguments: the string representation of the number and the base of the number system. For hexadecimal, the base is 16. Here’s how it works:
hex_value = "1A3F"
decimal_value = int(hex_value, 16)
print(decimal_value)
Output:
6719
In this example, we first define a hexadecimal string hex_value
with the value “1A3F”. We then pass this string and the base 16 to the int()
function, which converts the hexadecimal string into its decimal equivalent. The result is stored in decimal_value
, and when we print it, we see 6719, which is the decimal representation of the hexadecimal number 1A3F. This method is not only efficient but also easy to remember, making it a go-to approach for many developers.
Method 2: Using hex()
and ord()
Functions
Another interesting way to convert hexadecimal to decimal in Python involves using the hex()
and ord()
functions. This method is a bit more manual but can be useful for educational purposes. Here’s how you can implement it:
hex_value = "1A3F"
decimal_value = 0
length = len(hex_value)
for i in range(length):
digit = hex_value[length - i - 1]
decimal_value += int(digit, 16) * (16 ** i)
print(decimal_value)
Output:
6719
In this method, we initialize decimal_value
to zero and calculate the length of the hexadecimal string. We then loop through each character of the hexadecimal string from the end to the beginning. For each character, we convert it to its decimal equivalent using int(digit, 16)
and multiply it by 16 raised to the power of its position. Finally, we sum these values to get the decimal equivalent. This method highlights the underlying mechanics of base conversion and can deepen your understanding of how different number systems work.
Method 3: Using a Custom Function
If you want to encapsulate the conversion logic into a reusable function, you can create your own custom function to convert hexadecimal to decimal. This is particularly useful if you plan to perform this conversion multiple times throughout your code. Here’s how to do it:
def hex_to_decimal(hex_value):
decimal_value = 0
length = len(hex_value)
for i in range(length):
digit = hex_value[length - i - 1]
decimal_value += int(digit, 16) * (16 ** i)
return decimal_value
result = hex_to_decimal("1A3F")
print(result)
Output:
6719
In this example, we define a function called hex_to_decimal()
that takes a hexadecimal string as an argument. The function follows a similar logic to the previous method, calculating the decimal value through a loop. After defining the function, we call it with the hexadecimal string “1A3F” and print the result. This approach not only keeps your code clean and organized but also makes it easier to maintain and reuse.
Conclusion
Converting hexadecimal to decimal in Python is a straightforward task, thanks to the versatility of the language. Whether you choose to use the built-in int()
function, implement a manual conversion method, or create a reusable function, Python provides you with the tools to handle these conversions efficiently. Mastering these techniques will not only enhance your programming skills but also prepare you for various real-world applications. Keep practicing, and soon you’ll be converting numbers like a pro!
FAQ
-
How does the
int()
function work for hexadecimal conversion?
Theint()
function takes a string representation of a number and its base, converting it to a decimal integer. For hexadecimal, the base is 16. -
Can I convert lowercase hexadecimal values using these methods?
Yes, both uppercase and lowercase hexadecimal values can be converted using the methods described, as Python is case-insensitive for hexadecimal digits.
-
What if the hexadecimal string contains invalid characters?
If the string contains invalid characters (like letters beyond A-F or numbers beyond 9), Python will raise a ValueError when using theint()
function. -
Are there performance differences between the methods?
The built-inint()
function is generally faster and more efficient than manual methods, as it is optimized for performance in Python. -
Can I convert decimal back to hexadecimal in Python?
Yes, you can use thehex()
function to convert a decimal integer back to a hexadecimal string.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn