How to Convert Hex to ASCII in Python
-
Use the
decode()
Method to Convert Hex to ASCII in Python -
Use the
codecs.decode()
Method to Convert Hex to ASCII in Python -
Use the
binascii
Module to Convert Hex to ASCII in Python - Use List Comprehension to Convert Hex to ASCII in Python
-
Use the
int.to_bytes()
Method to Convert Hex to ASCII in Python -
Use the
struct
Module to Convert Hex to ASCII in Python - Conclusion
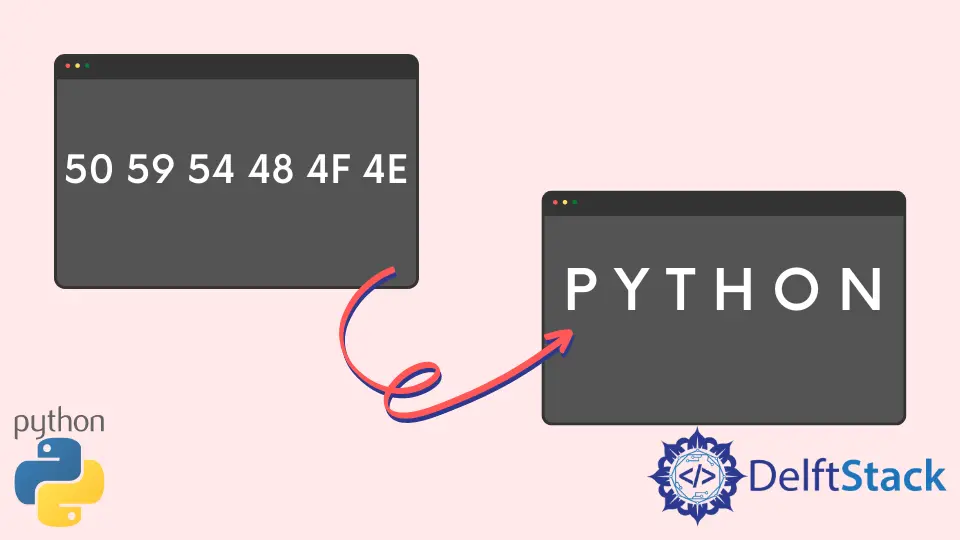
Converting a hexadecimal string to an ASCII string is important in Python, especially when dealing with binary data or communicating with hardware.
This tutorial will look into various methods to convert a hexadecimal string to an ASCII string in Python. Suppose we have a string written in hexadecimal form, 68656c6c6f
, and we want to convert it into an ASCII string, which will be hello
(in ASCII code h
= 68
, e
= 65
, l
= 6c
and o
= 6f
).
Use the decode()
Method to Convert Hex to ASCII in Python
The string.decode(encoding, error)
method in Python 2 takes an encoded string as input and decodes it using the character encoding specified in the encoding
argument. The error
parameter specifies the error handling schemes to use in case of an error that can be strict
, ignore
, and replace
.
Therefore, to convert a hex string to an ASCII string, we must set the encoding
parameter of the string.decode()
method as hex
. The below example code demonstrates how to use the string.decode()
method to convert a hex to ASCII in Python 2.
string = "68656c6c6f"
print(string.decode("hex"))
Output:
hello
In Python 3, the bytearray.decode(encoding, error)
method takes a byte array as input and decodes it using the character encoding specified in the encoding
argument.
To decode a string in Python 3, we first need to convert it to a byte array and then use the bytearray.decode()
method. The bytearray.fromhex(string)
method can first convert the string into a byte array.
The below example code demonstrates how to use the bytearray.decode()
and bytearray.fromhex(string)
methods to convert a hex string to an ASCII string in Python 3.
string = "68656c6c6f"
byte_array = bytearray.fromhex(string)
print(byte_array.decode())
Output:
hello
Use the codecs.decode()
Method to Convert Hex to ASCII in Python
The codecs.decode(obj, encoding, error)
method is similar to decode()
. It takes an object as input and decodes it using the character encoding specified in the encoding
argument. The error
argument specifies the error handling scheme to be used in case of an error.
In Python 2, the codecs.decode()
returns a string as output; in Python 3, it returns a byte array. The below example code demonstrates how to convert a hex string to ASCII using the codecs.decode()
method and convert the returned byte array to string using the str()
method.
import codecs
string = "68656c6c6f"
binary_str = codecs.decode(string, "hex")
print(str(binary_str, "utf-8"))
Output:
hello
Use the binascii
Module to Convert Hex to ASCII in Python
The binascii
module in Python provides methods for converting between binary data and ASCII text. The unhexlify()
function converts hexadecimal strings to bytes.
import binascii
# Hexadecimal representation of "Hello World"
hex_string = "48656c6c6f20576f726c64"
ascii_string = binascii.unhexlify(hex_string).decode("utf-8")
print(ascii_string)
import binascii
: This line imports thebinascii
module, which provides various binary-to-text encoding and decoding functions.hex_string = "48656c6c6f20576f726c64"
: This line initializes a variablehex_string
with a hexadecimal representation of the ASCII string"Hello World"
.ascii_string = binascii.unhexlify(hex_string).decode('utf-8')
: Here, we usebinascii.unhexlify()
to convert the hexadecimal string to bytes, thendecode()
into a UTF-8 string.print(ascii_string)
: This line prints the resulting ASCII string.
Output:
Hello World
Another function of the binascii
module in Python to convert hexadecimal string to an ASCII string is binascii.a2b_hex()
. This function is used to convert ASCII-encoded hexadecimal data to binary data.
Here’s an example code:
import binascii
# Hexadecimal representation of "Hello World"
hex_string = "48656c6c6f20576f726c64"
binary_data = binascii.a2b_hex(hex_string)
ascii_string = binary_data.decode("utf-8")
print(ascii_string)
import binascii
: This line imports thebinascii
module.hex_string = "48656c6c6f20576f726c64"
: This line initializes a variablehex_string
with a hexadecimal representation of the ASCII string"Hello World"
.binary_data = binascii.a2b_hex(hex_string)
: This line usesbinascii.a2b_hex()
to convert the hexadecimal string to binary data.ascii_string = binary_data.decode('utf-8')
: The resulting binary data is then decoded into a UTF-8 string.print(ascii_string)
: This line prints the resulting ASCII string,"Hello World"
.
Use List Comprehension to Convert Hex to ASCII in Python
This method involves iterating over the hexadecimal string and converting pairs of characters to their corresponding ASCII characters.
# Hexadecimal representation of "Hello World"
hex_string = "48656c6c6f20576f726c64"
ascii_string = "".join(
[chr(int(hex_string[i : i + 2], 16)) for i in range(0, len(hex_string), 2)]
)
print(ascii_string)
hex_string = "48656c6c6f20576f726c64"
: This line initializes a variablehex_string
with a hexadecimal representation of the ASCII string"Hello World"
.[chr(int(hex_string[i:i+2], 16)) for i in range(0, len(hex_string), 2)]
: This list comprehension iterates over the hexadecimal string in pairs, converts them to integers, and then to corresponding ASCII characters usingchr()
.''.join(...)
: The resulting list of characters is joined into a single string.print(ascii_string)
: This line prints the resulting ASCII string.
Output:
Hello World
Use the int.to_bytes()
Method to Convert Hex to ASCII in Python
Python’s to_bytes()
method can convert an integer to a bytes object. When combined with the int()
function for hexadecimal conversion, this can convert a hexadecimal string to an ASCII string.
Here’s an example code:
# Hexadecimal representation of "Hello World"
hex_string = "48656c6c6f20576f726c64"
num = int(hex_string, 16) # Convert hex string to integer
# Convert integer to bytes with big-endian encoding
binary_data = num.to_bytes((num.bit_length() + 7) // 8, byteorder="big")
# Convert binary data to ASCII string
ascii_string = binary_data.decode("utf-8")
print(ascii_string)
hex_string = "48656c6c6f20576f726c64"
: This line initializes a variablehex_string
with a hexadecimal representation of the ASCII string"Hello World"
.num = int(hex_string, 16)
: This line usesint()
to convert the hexadecimal string to an integer.num.to_bytes((num.bit_length() + 7) // 8, byteorder='big')
: Here, we useto_bytes()
to convert the integer to a bytes object. The length of the bytes is determined by(num.bit_length() + 7) // 8
, which ensures enough bytes to represent the integer.binary_data.decode('utf-8')
: The resulting binary data is decoded into a UTF-8 string.print(ascii_string)
: This line prints the resulting ASCII string,"Hello World"
.
Use the struct
Module to Convert Hex to ASCII in Python
Python’s struct
module provides functions to interpret packed binary data. In this case, we can convert a hexadecimal string to a binary representation and decode it into an ASCII string.
Here’s an example code:
import struct
# Hexadecimal representation of "Hello World"
hex_string = "48656c6c6f20576f726c64"
# Unpack hexadecimal string to binary data
binary_data = struct.pack(
"!{}s".format(len(hex_string) // 2), bytes.fromhex(hex_string)
)
# Convert binary data to ASCII string
ascii_string = binary_data.decode("utf-8")
print(ascii_string)
import struct
: This line imports thestruct
module, which provides functions to interpret packed binary data.hex_string = "48656c6c6f20576f726c64"
: This line initializes a variablehex_string
with a hexadecimal representation of the ASCII string"Hello World"
.struct.pack('!{}s'.format(len(hex_string)//2), bytes.fromhex(hex_string))
: This line usesstruct.pack()
to convert the hexadecimal string to a binary representation. The format'!{}s'.format(len(hex_string)//2)
specifies that we are packing a binary string of lengthlen(hex_string)//2
in big-endian byte order('!')
. Thebytes.fromhex(hex_string)
convert the hex string to binary data.binary_data.decode('utf-8')
: The resulting binary data is decoded into a UTF-8 string.print(ascii_string)
: This line prints the resulting ASCII string,"Hello World"
.
Conclusion
In this article, we’ve explored six methods to convert a hexadecimal string to an ASCII string in Python. Each method has its strengths and use cases, so choose the one that best suits your application.
Understanding these methods will give you greater flexibility in handling binary data in your Python projects.
Related Article - Python ASCII
- How to Convert String to ASCII Value in Python
- How to Convert Int to ASCII in Python
- How to Get ASCII Value of a Character in Python