How to Get ASCII Value of a Character in Python
-
Method 1: Using the
ord()
Function - Method 2: Using a Loop for Multiple Characters
-
Method 3: Using the
map()
Function - Method 4: Creating a Custom Function
- Conclusion
- FAQ
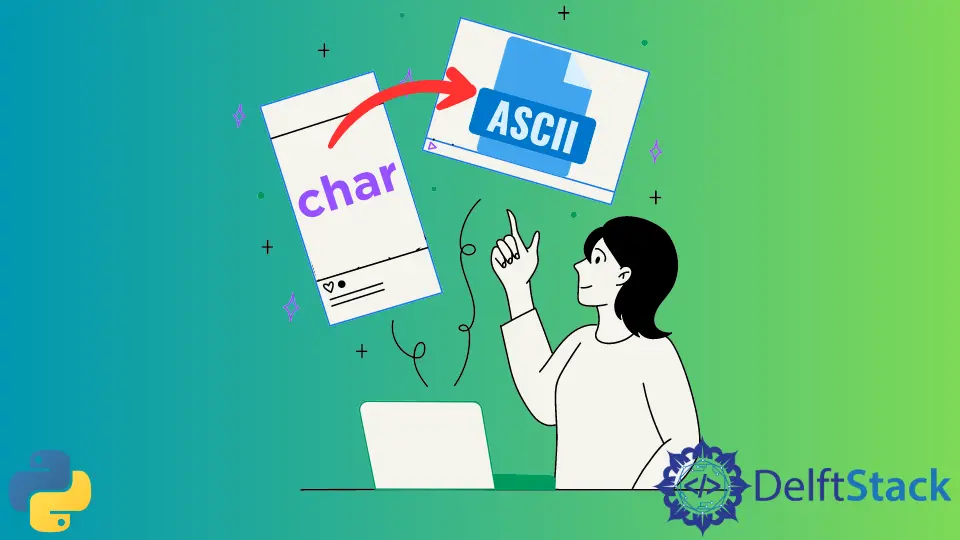
Understanding how to obtain the ASCII value of a character in Python is essential for various programming tasks, especially when dealing with text processing, encoding, and data manipulation.
In this tutorial, we will walk you through different methods to achieve this using Python’s built-in functions. Whether you are a beginner or an experienced programmer, this guide will equip you with the knowledge you need to efficiently work with ASCII values in your Python projects. Let’s dive into the world of ASCII and see how you can effortlessly convert characters into their corresponding ASCII values.
Method 1: Using the ord()
Function
One of the simplest and most effective ways to get the ASCII value of a character in Python is by using the built-in ord()
function. This function takes a single character as an argument and returns its corresponding ASCII (or Unicode) integer value. Here’s how it works:
char = 'A'
ascii_value = ord(char)
print(ascii_value)
Output:
65
In this example, we define a character char
with the value ‘A’. We then pass this character to the ord()
function, which returns the ASCII value of ‘A’, which is 65. This method is straightforward and efficient for anyone looking to convert characters to their ASCII values. You can use this function for any single character, including uppercase and lowercase letters, numbers, and special symbols.
Method 2: Using a Loop for Multiple Characters
If you need to find the ASCII values for multiple characters at once, you can use a loop. This method is particularly useful when you want to convert an entire string into its ASCII values. Here’s how you can do it:
string = "Hello"
ascii_values = [ord(char) for char in string]
print(ascii_values)
Output:
[72, 101, 108, 108, 111]
In this code, we define a string string
with the value “Hello”. We then use a list comprehension to iterate over each character in the string, applying the ord()
function to get the ASCII value of each character. The result is a list of ASCII values corresponding to each character in the string. This method is efficient and very readable, making it easy to convert longer strings into their ASCII representations. You can easily modify the string to include any characters you wish to convert.
Method 3: Using the map()
Function
Another elegant way to convert a string of characters to their ASCII values is by using the map()
function in Python. This functional programming approach allows you to apply a function to all items in an iterable, such as a string. Here’s an example of how to use map()
:
string = "World"
ascii_values = list(map(ord, string))
print(ascii_values)
Output:
[87, 111, 114, 108, 100]
In this example, we define a string string
with the value “World”. We then use the map()
function, passing ord
as the function to apply and string
as the iterable. The map()
function returns an iterator, so we convert it to a list to display the ASCII values. This method is concise and leverages Python’s functional programming capabilities, making it a great choice for those familiar with this programming paradigm. It’s also very efficient for larger strings.
Method 4: Creating a Custom Function
For those who prefer a more tailored approach, you can create your own function to get the ASCII values of characters. This method allows you to add additional functionality, such as error handling or formatting options. Here’s a simple example:
def get_ascii_values(input_string):
return [ord(char) for char in input_string]
result = get_ascii_values("Python")
print(result)
Output:
[80, 121, 116, 104, 111, 110]
In this code, we define a function get_ascii_values
that takes an input string and returns a list of ASCII values for each character. We then call this function with the string “Python” and print the result. This method provides flexibility and allows you to customize the function to meet specific needs, such as filtering out non-ASCII characters or formatting the output in a particular way.
Conclusion
Getting the ASCII value of a character in Python is a straightforward process, thanks to the built-in ord()
function and various programming techniques like loops and functional programming. Whether you need to convert a single character or an entire string, Python provides you with multiple methods to achieve your goals efficiently. By mastering these techniques, you can enhance your programming skills and tackle a variety of text processing tasks with ease.
FAQ
-
What is ASCII?
ASCII stands for American Standard Code for Information Interchange, which is a character encoding standard for electronic communication. -
Can I get ASCII values for non-ASCII characters?
Theord()
function can return Unicode values for characters beyond the standard ASCII range, but for true ASCII, only values from 0 to 127 are applicable. -
Is there a way to convert ASCII values back to characters?
Yes, you can use thechr()
function in Python to convert an ASCII value back to its corresponding character. -
Can I use
ord()
with multiple characters?
No,ord()
only accepts a single character. For multiple characters, you would need to use a loop or a comprehension. -
What is the difference between
ord()
andchr()
?
ord()
converts a character to its ASCII value, whilechr()
converts an ASCII value back to its corresponding character.