How to Convert Int to ASCII in Python
-
Use the
chr()
Function to Convertint
to ASCII in Python -
Use the
unichr()
Function to Convertint
to ASCII in Python 2 -
Use String Formatting to Convert
int
to ASCII in Python - Conclusion
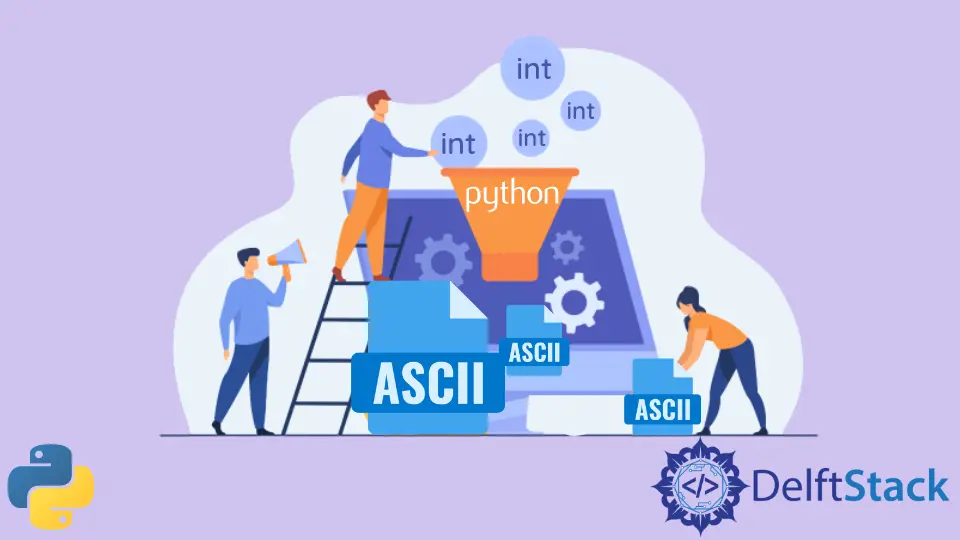
In Python programming, the need to convert integers into their corresponding ASCII characters is a common requirement. This operation is fundamental for tasks such as data encoding, text manipulation, and communication protocol handling.
Python provides multiple methods for achieving this conversion, including the chr()
function, string formatting, and the legacy unichr()
function in Python 2. In this article, we will explore these techniques, providing step-by-step guidance on how to employ them effectively.
Use the chr()
Function to Convert int
to ASCII in Python
One of the simplest and most straightforward ways to convert integers to their corresponding ASCII characters is by using the chr()
function.
The chr()
function is a built-in Python function that takes an integer representing a Unicode code point and returns the corresponding character as a string. This character can be an ASCII character or any other character in the Unicode standard.
When you provide an integer as an argument to chr()
, it will return the character associated with that Unicode code point.
To convert an integer to its ASCII representation using the chr()
function, follow these simple steps:
-
Identify the integer you want to convert to ASCII. This integer should represent the ASCII code of the desired character.
-
Use the
chr()
function and pass the integer as an argument to it. -
The
chr()
function will return a single-character string representing the ASCII character. -
Store or use the result as needed in your code.
Let’s convert a few ASCII codes to their respective characters using the chr()
function.
ascii_code_65 = 65
ascii_code_98 = 98
char_A = chr(ascii_code_65)
char_b = chr(ascii_code_98)
print(char_A)
print(char_b)
Here, two ASCII codes, 65
and 98
, are converted into their respective ASCII characters using the chr()
function. The ascii_code_65
variable is assigned the value 65
, which corresponds to the ASCII code for the character A
, while ascii_code_98
is assigned the value 98
, representing the ASCII code for the character b
.
The chr()
function is then used to convert these ASCII codes into their respective characters, storing them in char_A
and char_b
.
The output of the provided code will be as follows:
A
b
You can also use the chr()
function within a loop to convert a list of ASCII codes into a string.
ascii_codes = [72, 101, 108, 108, 111]
ascii_characters = [chr(code) for code in ascii_codes]
result = "".join(ascii_characters)
print(result)
Here, ascii_codes
is a list containing five integers. A list comprehension is then used to convert each ASCII code in ascii_codes
into its corresponding character using the chr()
function.
This results in a list named ascii_characters
that contains the characters H
, e
, l
, l
, and o
. The join()
method is then applied to concatenate the characters in the ascii_characters
list into a single string, which is stored in the result
variable.
So, the expected output of the code is:
Hello
Use the unichr()
Function to Convert int
to ASCII in Python 2
In Python 2, alongside the chr()
function, you can use the unichr()
function to convert integers to their respective Unicode characters. The unichr()
function is particularly useful when dealing with Unicode characters, and it provides the Unicode string for a given number.
Here’s an example of using unichr()
to convert an integer to an ASCII character in Python 2:
# python 2
a = unichr(101)
print a
In this code, the unichr(101)
call converts the integer 101
into its corresponding Unicode character, which happens to be e
.
Therefore, the output of this code will be:
e
It’s important to note that in Python 3 and later versions, there is no distinction between regular strings and Unicode strings, as all strings are Unicode by default.
To convert the ASCII value (character) back to an integer, you can use the ord()
function. The ord()
function takes a string of unit length (i.e., a single character) as an argument and returns the Unicode equivalent as an integer.
It serves as the reverse counterpart to the chr()
and unichr()
functions and is a built-in function in Python.
Here’s an example using the ord()
function to convert the character e
back to its ASCII code:
print(ord("e"))
The output of this code will be:
101
In this case, the ord("e")
call returns the integer 101
, which is the ASCII code for the character e
. This demonstrates how you can use the ord()
function to convert characters back to their corresponding ASCII values.
Use String Formatting to Convert int
to ASCII in Python
String formatting in Python is a powerful way to create formatted strings. One of the formatting options involves the %
operator or the newer f-strings
.
By using formatting placeholders, it’s possible to insert values into strings, including integers that represent ASCII codes. By specifying the format type %c
for characters or using an f"{number:c}"
syntax, it’s feasible to convert integers into their ASCII characters.
To convert an integer to its ASCII representation using string formatting, follow these steps:
-
Identify the integer value representing the desired ASCII code. Refer to an ASCII table to find the specific ASCII code for a character.
-
Use string formatting, either with the
%c
formatting option or thef"{number:c}"
syntax, specifying the integer as the placeholder value. -
The formatted string will represent the ASCII character corresponding to the provided integer.
Let’s convert an ASCII code to its corresponding character using the %c
formatting option.
ascii_code_65 = 65
char_A = "%c" % ascii_code_65
print(char_A)
Output:
A
In this example, the ASCII code 65
, which corresponds to the character A
, is converted to its character representation using the %c
formatting option. This option is applied within a string where the %
operator acts as a placeholder for the character.
The %c
indicates that the subsequent value (in this case, ascii_code_65
) should be treated as a character. After the formatting, the result is stored in the variable char_A
, and when we print it, the output is A
, representing the character A
.
Alternatively, the f"{number:c}"
syntax can be used to convert ASCII codes to characters.
ascii_code_66 = 66
char_B = f"{ascii_code_66:c}"
print(char_B)
Output:
B
In the second example, the f"{number:c}"
syntax is used to achieve the same conversion. This modern Python formatting method allows you to include the :c
specifier within an f-string.
The specifier :c
indicates that the following value (ascii_code_66
) should be interpreted as a character. Similar to the first example, the result is stored in the variable char_B
, and when printed, it displays B
, which corresponds to the character associated with the ASCII code 66
.
Both methods provide a convenient way to convert ASCII codes to characters, with the choice between them depending on your coding style and preference.
Conclusion
This article explored three key methods: the chr()
function for simplicity, string formatting for flexibility, and the Python 2 unichr()
function for Unicode characters. With these techniques, you can confidently handle a variety of programming tasks involving ASCII characters.
Whether you’re encoding, decoding, or working on other projects, Python equips you with the right tools. By mastering these methods, you’ll be well-prepared to tackle a wide range of programming challenges.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python Integer
- How to Convert Int to Binary in Python
- How to Convert Roman Numerals to Integers in Python
- How to Convert Integer to Roman Numerals in Python
- Integer Programming in Python
- How to Convert Boolean Values to Integer in Python
- How to Convert String to Integer in Python