How to Convert Hex to Base64 in Python
-
Convert HEX to BASE64 With the
codecs
Module in Python -
Convert HEX to BASE64 With the
base64
Module in Python -
Convert HEX to BASE64 With the
binascii
Module in Python - Convert HEX to BASE64 in Python2
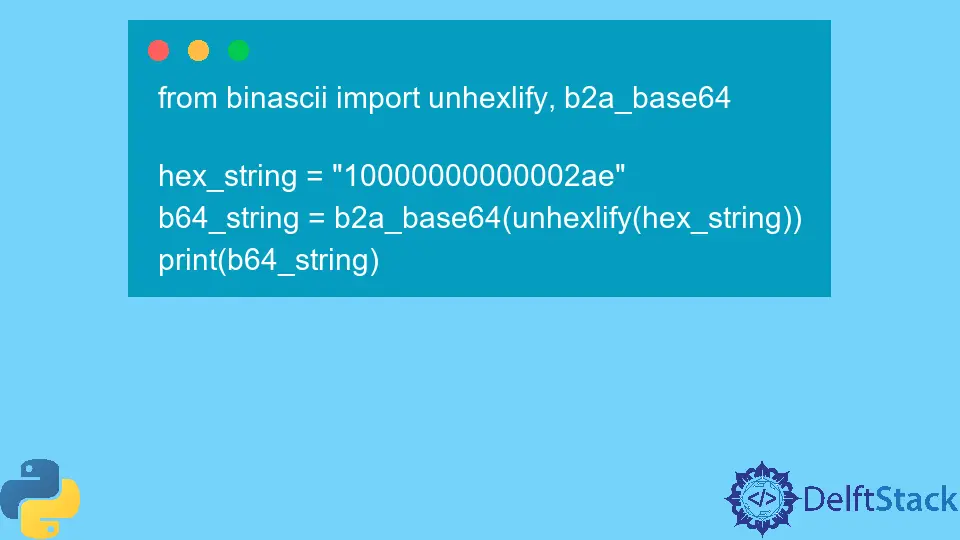
This tutorial will discuss the methods to convert hex to base64 in Python.
Convert HEX to BASE64 With the codecs
Module in Python
The codecs
module in Python provides the encode()
and decode()
methods to convert between different text encoding formats.
The encode()
function takes two parameters; an input string and the format in which it needs to encode that string. The return type of this encode()
function is a string encoded in the format specified in the second parameter.
The decode()
function also takes only two parameters; an encoded input string and the format in which the input string is encoded. The decode()
function returns a decoded string according to the format specified in the second parameter.
To encode a hex string into a base64 string, we’ll first decode that hex string into a regular string with the decode()
function by specifying hex
as the second parameter. We’ll then encode the output of the previous operation with the encode()
function by specifying base64
as the second parameter.
The following code snippet elaborates on the steps required to encode a hex string into a base64 string with the codecs
module in Python.
import codecs
hex_string = "10000000000002ae"
b64_string = codecs.encode(codecs.decode(hex_string, "hex"), "base64").decode()
print(b64_string)
Output:
EAAAAAAAAq4=
In the above code, we encode the hex string 10000000000002ae
into the base64 string EAAAAAAAAq4=
with the codecs
module.
Convert HEX to BASE64 With the base64
Module in Python
Another useful module in Python to convert between different text encoding formats is base64
. The base64
module provides b64encode()
and b64decode()
functions for this task.
The b64encode()
function takes the byte string as an input parameter, converts it into the base64 format, and returns the base64 encoded string. Similarly, the b64decode()
function takes the base64 encoded string, converts it into a byte string, and returns the decoded byte string.
For this particular problem, we’ll have to first convert our input string into a byte string with the bytes.fromhex()
function. We’ll then encode that byte string into a base64 string with the b64encode()
function.
The following code snippet demonstrates how we can encode a hex string into a base64 string with the base64
module in Python.
from base64 import b64encode, b64decode
hex_string = "10000000000002ae"
b64_string = b64encode(bytes.fromhex(hex_string)).decode()
print(b64_string)
Output:
EAAAAAAAAq4=
In the above code, we encode the hex string 10000000000002ae
into the base64 string EAAAAAAAAq4=
with the base64
module.
Convert HEX to BASE64 With the binascii
Module in Python
We can also use Python’s binascii
module to convert between different text encoding formats. The binascii
module provides functions like unhexlify()
and b2a_base64()
which can be used in this scenario.
The unhexlify()
function takes the hex string as an input parameter, converts it into a byte string, and returns the results. The b2a_base64()
function takes a byte string as an input parameter, converts it into a base64 string, and returns the results.
In our particular problem, we need to first convert the hex string into a byte string with the unhexlify()
function and then use the b2a_base64()
function to convert the result of the previous operation into a base64 string.
The following code example demonstrates how we can use the binascii
module to convert a hex string to base64 in Python.
from binascii import unhexlify, b2a_base64
hex_string = "10000000000002ae"
b64_string = b2a_base64(unhexlify(hex_string))
print(b64_string)
Output:
EAAAAAAAAq4=
In the above code, we encode the hex string 10000000000002ae
into the base64 string EAAAAAAAAq4=
with the binascii
module. Out of all the methods discussed above, the binascii
method is the most straightforward, and we need to write the least amount of code to implement it.
Convert HEX to BASE64 in Python2
On the other hand, if we are working with Python2, which natively supports both hex and base64 strings, we can drastically reduce the amount of code required. The following code snippet demonstrates how we can convert a hex string into a base64 string in Python2.
hex_string = "10000000000002ae"
b64_string = hex_string.decode("hex").encode("base64")
print(b64_string)
Output:
EAAAAAAAAq4=
We didn’t need to import any modules in the above code because Python2 natively supports hex and base64 strings. We first converted the hex string into byte string with the decode("hex")
function and then encoded its output to base64 with the encode("base64")
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn