How to Convert Binary to Hex in Python
- Use a User-Defined Function to Convert Binary to Hex in Python
-
Use the
int()
andhex()
Functions to Convert Binary to Hex in Python -
Use the
binascii
Module to Convert Binary to Hex in Python -
Use the
format()
Function to Convert Binary to Hex in Python - Use F-Strings to Convert Binary to Hex in Python
- Conclusion
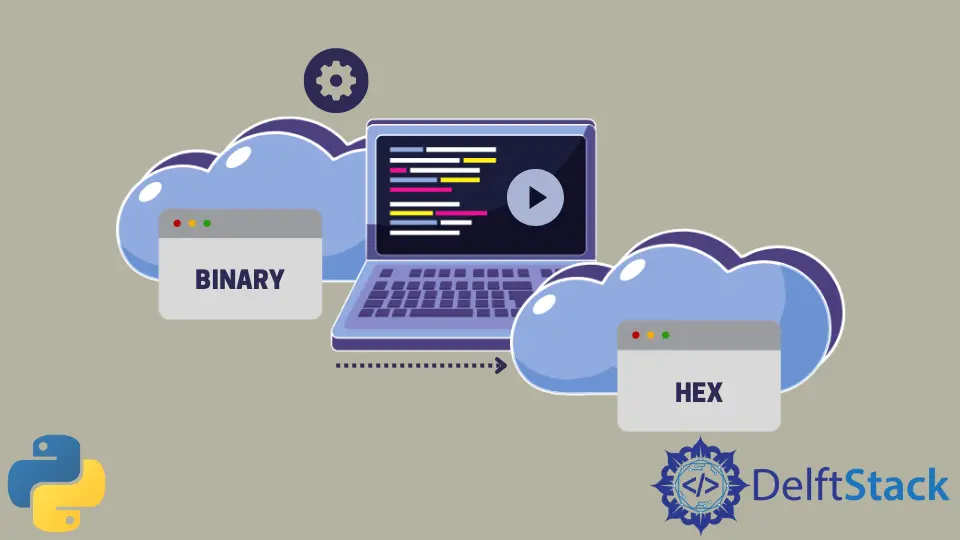
Binary and hexadecimal numeral systems are fundamental in the field of computer science, and Python offers a range of tools and functions to facilitate conversions between these systems. Understanding how to convert binary data to hexadecimal is crucial, as it plays a significant role in various applications, from data representation to low-level programming.
In this article, we’ll explore five distinct methods for converting binary data to hexadecimal in Python.
Use a User-Defined Function to Convert Binary to Hex in Python
We can convert binary to hexadecimal using a user-defined function and a while
loop. This approach allows for greater control and customization while understanding the conversion process step by step.
Before we dive into the Python code, let’s briefly review binary and hexadecimal numeral systems.
Binary is a base-2 numeral system, meaning it uses only two digits, 0
and 1
. Each digit in a binary number represents a power of 2.
For example, 1010
in binary is equivalent to 10
in decimal.
Hexadecimal is a base-16 numeral system, using digits 0-9 and the letters A-F to represent values from 0 to 15. Each digit in a hexadecimal number corresponds to a power of 16.
For example, 1A
in hexadecimal is equivalent to 26
in decimal.
To formulate the code for converting binary to hexadecimal using a user-defined function and a while
loop in Python, you can follow these main steps:
-
Create a user-defined function with a meaningful name, such as
binary_to_hex
, to encapsulate the conversion process. -
Depending on your requirements, you may want to add input validation to ensure that the binary string is valid (contains only
0
and1
). -
To facilitate the conversion, ensure that the length of the binary string is a multiple of 4 by padding it with leading zeros if necessary. You can use the
zfill
method for this purpose. -
Create variables to store the hexadecimal characters and the resulting hexadecimal string. Define a string containing the hexadecimal digits (0-9 and A-F) for mapping.
-
Use a
while
loop to iterate through the binary string until it’s empty. In each iteration:- Extract the rightmost 4 bits (a nibble) from the binary string.
- Convert the nibble to a decimal value using the
int()
function. - Map the decimal value to the corresponding hexadecimal digit using the predefined hexadecimal characters.
- Prepend the hexadecimal digit to the result string.
- Remove the processed nibble from the binary string by slicing it.
-
Once the
while
loop has processed all the binary digits, return the resulting hexadecimal string. -
Test your function by providing binary input and printing the converted hexadecimal output. This will help you ensure that the function works as expected.
Here’s a summarized code structure with these steps:
def binary_to_hex(binary_str):
binary_str = binary_str.zfill((len(binary_str) + 3) // 4 * 4)
hex_chars = "0123456789ABCDEF" # Hexadecimal digits
hex_str = "" # Initialize the hexadecimal result string
while binary_str:
nibble = binary_str[-4:]
decimal_value = int(nibble, 2)
hex_digit = hex_chars[decimal_value]
hex_str = hex_digit + hex_str
binary_str = binary_str[:-4]
return hex_str
binary_str = "1101101101101101"
hex_str = binary_to_hex(binary_str)
print(f"Binary: {binary_str}\nHexadecimal: {hex_str}")
When you run this code, it will take the input binary string 1101101101101101
and convert it to the corresponding hexadecimal representation.
The output will be:
Binary: 1101101101101101
Hexadecimal: DB6D
You can replace the binary_str
variable with your binary input to convert it to hexadecimal using the binary_to_hex
function.
Use the int()
and hex()
Functions to Convert Binary to Hex in Python
To convert binary to hexadecimal in Python, we can also use the int()
function to first convert the binary string to an integer and then use the hex()
function to obtain the hexadecimal representation.
The hex()
function is specifically used to convert an integer to its hexadecimal representation. Here is the basic syntax of the hex()
function:
hex(x)
x
: An integer value that you want to convert to hexadecimal. It can be of typeint
,str
, or any other object that can be converted to an integer.
The hex()
function returns a string representing the hexadecimal value of the input integer. The resulting string will have a 0x
prefix to indicate that it’s in hexadecimal format.
For example:
binary_str = "1101101101101101"
decimal_value = int(binary_str, 2)
hex_str = hex(decimal_value)
print(f"Binary: {binary_str}")
print(f"Hexadecimal: {hex_str}")
When you run this code, it will output:
Binary: 1101101101101101
Hexadecimal: 0xdb6d
The int()
function is used to convert the binary string 1101101101101101
to its decimal equivalent. The second argument, 2
, specifies that the input is in base-2 (binary).
After converting to decimal, we use the hex()
function to get the hexadecimal representation, which is prefixed by 0x
.
If you want to remove the 0x
prefix and obtain the hexadecimal value as a plain string, you can use string slicing like this:
hex_str = hex(decimal_value)[2:] # Remove the "0x" prefix
print(f"Hexadecimal: {hex_str}")
The output will then be:
Binary: 1101101101101101
Hexadecimal: db6d
This removes the 0x
prefix, leaving you with a clean hexadecimal representation.
Use the binascii
Module to Convert Binary to Hex in Python
The binascii
module provides a straightforward and efficient way to perform binary to hexadecimal conversions. This Python module provides various functions for working with binary data.
To convert binary data to hexadecimal, we can use the binascii.hexlify()
function. This function takes a bytes-like object as input and returns its hexadecimal representation as a bytes object.
-
To use the
binascii
module and its functions, you need to import it first. You can do this with the following code:import binascii
-
Create or obtain your binary data as a bytes-like object. This could be in the form of binary data read from a file, received over a network, or any other source.
-
Use the
binascii.hexlify()
function to convert the binary data into its hexadecimal representation. This function takes the binary data as input and returns the hexadecimal data as a bytes object. -
The result of the
binascii.hexlify()
function is a bytes object containing the hexadecimal data. To obtain the hexadecimal representation as a string, you can decode the bytes object using thedecode()
method.
Here’s how you can use it:
import binascii
binary_data = b"\x10\x20\x30"
hexadecimal_data = binascii.hexlify(binary_data)
print(f"Binary Data: {binary_data}")
print(f"Hexadecimal Data: {hexadecimal_data.decode('utf-8')}")
When you run this code, it will output:
Binary Data: b'\x10 0'
Hexadecimal Data: 102030
In this code, we import the binascii
module and create a binary data object, binary_data
. We then use the binascii.hexlify()
function to convert it to its hexadecimal representation.
Finally, we decode the hexadecimal data to a string using the decode()
method.
Handling Larger Binary Data
If you have a binary string, you can convert it to bytes and then use the binascii.hexlify()
function. Here’s another example:
import binascii
binary_str = "1101101101101101"
binary_data = bytes(int(binary_str[i : i + 8], 2) for i in range(0, len(binary_str), 8))
hexadecimal_data = binascii.hexlify(binary_data)
print(f"Binary String: {binary_str}")
print(f"Hexadecimal Data: {hexadecimal_data.decode('utf-8')}")
The output will then be:
Binary String: 1101101101101101
Hexadecimal Data: db6d
In this code, we first convert the binary string 1101101101101101
to a bytes object by grouping it into 8-bit chunks. Then, we use binascii.hexlify()
to obtain the hexadecimal representation.
Use the format()
Function to Convert Binary to Hex in Python
The format()
function in Python provides a flexible way to format and convert values into different representations, including hexadecimal. Here’s how it is used:
Before using the format()
function, you need to convert the binary data to its decimal equivalent using the int()
function. For example, if you have a binary string binary_str
, you can convert it to a decimal integer as follows:
decimal_value = int(binary_str, 2) # Convert binary to decimal
This is crucial because format()
expects a decimal integer as input.
Then, the format()
function allows you to convert the decimal integer to its hexadecimal representation by specifying the format specifier 'x'
. Here’s how you use format()
for the conversion:
# Convert decimal to hexadecimal
hexadecimal_string = format(decimal_value, "x")
In this line of code:
decimal_value
: The decimal integer you obtained from the binary data.'x'
: The format specifier, indicating that you want the output in lowercase hexadecimal format.
The result is the hexadecimal_string
, which contains the hexadecimal representation of the original binary data.
Here’s a complete working code:
binary_str = "1101101101101101" # Binary input
decimal_value = int(binary_str, 2) # Convert binary to decimal
# Convert decimal to hexadecimal
hexadecimal_string = format(decimal_value, "x")
print(f"Binary: {binary_str}")
print(f"Hexadecimal: {hexadecimal_string}")
When you run this code, it will output:
Binary: 1101101101101101
Hexadecimal: db6d
In this code, we first convert the binary string "1101101101101101"
to its decimal equivalent using int()
. Then, we use the format()
function to convert the decimal value to its hexadecimal representation.
The 'x'
format specifier tells format()
to use lowercase hexadecimal characters.
Changing Hexadecimal Format
If you want to change the formatting of the hexadecimal output, you can specify additional options in the format specifier. For example, to get uppercase hexadecimal characters with a 0x
prefix, you can use '#X'
as the format specifier:
# Uppercase hexadecimal with "0x" prefix
hexadecimal_string = format(decimal_value, "#X")
print(f"Hexadecimal: {hexadecimal_string}")
This will produce the following output:
Binary: 1101101101101101
Hexadecimal: 0XDB6D
The format()
function, in this context, provides control over the conversion process, allowing you to specify the output format, including the case of hexadecimal characters and the presence of a 0x
prefix. It’s a flexible and versatile tool for formatting and representing data in different numeral systems, making it valuable in various applications, from data visualization to encoding and network protocols.
Use F-Strings to Convert Binary to Hex in Python
F-strings, a feature introduced in Python 3.6, provides a concise and convenient way to format and display data with minimal code. To convert binary data to hexadecimal using f-strings, we first convert the binary string to an integer and then format it as hexadecimal using an f-string.
Here’s how you can do it:
binary_str = "1101101101101101" # Binary input
decimal_value = int(binary_str, 2) # Convert binary to decimal
hexadecimal_string = f"{decimal_value:x}" # Convert decimal to hexadecimal
print(f"Binary: {binary_str}")
print(f"Hexadecimal: {hexadecimal_string}")
When you run this code, it will output:
Binary: 1101101101101101
Hexadecimal: db6d
In this code, we first convert the binary string 1101101101101101
to its decimal equivalent using int()
. Then, we use an f-string with {decimal_value:x}
to convert the decimal value to its hexadecimal representation.
The :x
format specifier indicates that we want the output in lowercase hexadecimal.
F-strings provide a concise and readable way to format and display data, making them a great choice for converting binary data to hexadecimal. You can easily customize the output format by modifying the f-string, allowing you to control the case of hexadecimal characters or add prefixes if needed.
Customizing the Output Format
If you want to customize the output format, you can modify the f-string accordingly. For example, to get uppercase hexadecimal characters with a 0x
prefix, you can use {decimal_value:X}
:
# Uppercase hexadecimal with "0x" prefix
hexadecimal_string = f"0x{decimal_value:X}"
print(f"Hexadecimal: {hexadecimal_string}")
This will produce the following output:
Binary: 1101101101101101
Hexadecimal: 0xDB6D
F-strings provide flexibility and ease of use when formatting data, making them a valuable tool for converting and displaying values in different numeral systems, including hexadecimal. Whether you’re working with binary data in a file or need to represent data in various formats, f-strings simplify the process and enhance code readability.
Conclusion
Converting binary data to hexadecimal is a fundamental skill for any Python programmer, as it opens up possibilities for data representation and manipulation in a more human-friendly format. The methods discussed in this article each have their merits, and the choice of method depends on the specific needs of your project and your preferences as a developer.
Whether you opt for the binascii
module for its simplicity, the format()
function for its versatility, the hex()
function for converting integers, or f-strings for their concise and readable code, you now have a range of techniques at your disposal.
By understanding and mastering these methods, you’ll be well-equipped to work with binary data effectively, whether you’re dealing with data encoding, cryptography, network protocols, or any other area where binary-to-hexadecimal conversion is necessary. With these tools in your Python arsenal, you can confidently handle binary data and perform conversions with ease.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python Binary
- Binary Numbers Representation in Python
- How to Convert a String to Binary in Python
- How to Convert Binary to Int in Python