How to Convert Binary to Int in Python
-
Convert Binary to Integer in Python Using the
0b
Prefix -
Convert Binary to Integer in Python Using
int()
Function With Base 2 - Convert Binary to Integer in Python Using f-strings
- Convert Binary to Integer in Python Using a Custom Function
-
Convert Binary to Integer in Python Using the
bin()
Function - Convert Binary to Integer in Python Using List Comprehensions and Enumerate
- Convert Binary to Integer in Python Using Bitwise Left Shift Operations
- Conclusion
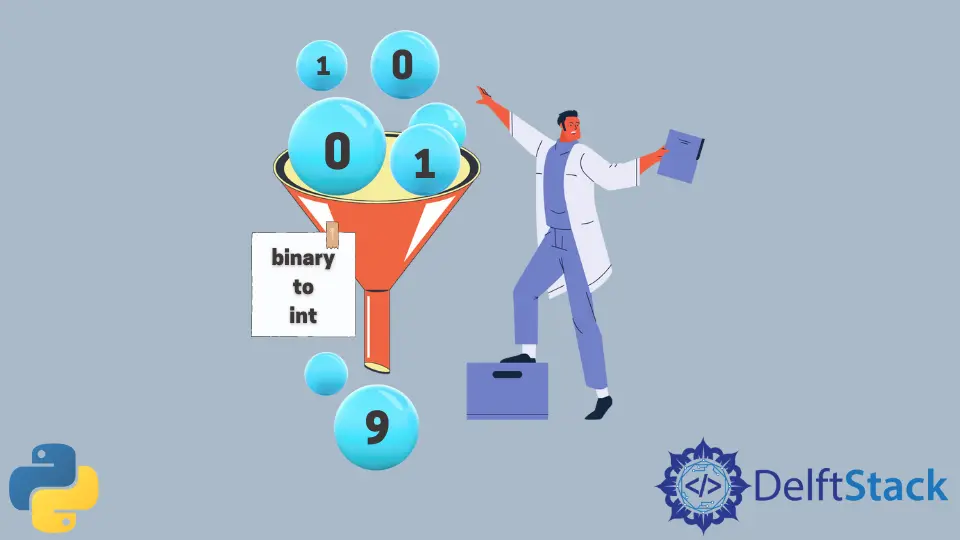
Binary to integer conversion is a fundamental operation in computer programming, especially when dealing with low-level data or working with binary data. In Python, there are multiple methods to perform this conversion, and one of the most straightforward techniques is using the 0b
prefix to indicate binary numbers.
In this article, we’ll explore the utilization of the 0b
prefix, along with other techniques like the int()
function, f-strings, custom functions, the bin()
function, list comprehensions, and bitwise left shift operations to convert binary representations to integers.
Convert Binary to Integer in Python Using the 0b
Prefix
In Python, the 0b
prefix is used to indicate that a literal number is in binary format. It serves as a marker to tell Python that the following sequence of digits represents a binary number.
For example, if you see a number written as 0b1010
, Python interprets it as the binary representation of the integer 10
.
Here’s how to convert a binary number with the 0b
prefix to an integer:
To convert a binary number to an integer, you must begin with a binary number. Make sure it’s prefixed with 0b
to indicate its binary nature.
binary_number = 0b1010
decimal_number = int(binary_number)
In this example, binary_number
is the binary representation of 10
.
Once you’ve converted the binary number to an integer, you can use it in your Python code, perform arithmetic operations, or display it as needed.
Let’s go through a practical example to demonstrate the conversion of a binary number to an integer using the 0b
prefix:
binary_number = 0b110101
print("Integer:", binary_number)
When you run this code, you’ll see the following output:
Integer: 53
In this example, we start with the binary number 0b110101
, which is equivalent to the decimal number 53
. The int()
function is used to convert it to an integer, and the result is printed to the console.
It’s important to note that the 0b
prefix is crucial when using the int()
function for binary-to-integer conversion. If the binary number is missing the 0b
prefix, that number will simply be a decimal number.
To avoid this, always make sure your binary number starts with the 0b
prefix.
Convert Binary to Integer in Python Using int()
Function With Base 2
Converting binary to integer is as simple as using the int()
function, specifying the binary string as the first argument, and setting the base to 2.
First, you need a binary string that you want to convert to an integer. For example, let’s use the binary string 1101
.
Apply the int()
function to the binary string, setting the base to 2:
binary_string = "1101"
decimal_integer = int(binary_string, 2)
print(decimal_integer)
In the code above, binary_string
is the binary input, and decimal_integer
will store the integer value of the binary string.
After executing the code, the decimal_integer
variable will contain the decimal (base-10) representation of the binary input. In this case, decimal_integer
will be 13
, as 1101
in binary is equivalent to 13
in decimal.
Using the int()
function with base 2 is advantageous for several reasons:
- This method is incredibly simple and easy to understand. It provides a clean and direct way to convert binary strings into integers.
- The
int()
function is a built-in Python function, which means you don’t need to write custom code to perform the conversion. It’s readily available and optimized for performance. - The
int()
function can also be used for converting strings from other bases, making it versatile for various numeral systems. - The use of
int()
with base 2 makes your code more readable and maintainable, as it clearly indicates the intent of the conversion.
Convert Binary to Integer in Python Using f-strings
In Python, f-strings provide a concise and readable way to format strings, including numeric values. To convert a binary string to an integer, you can use the int()
function within an f-string to perform the conversion on the fly. Here’s how it’s done:
You need a binary string as your input. This string should only consist of 0
s and 1
s, representing the binary number you want to convert to an integer.
An f-string is a string literal that starts with an f
or F
character. Within an f-string, you can embed expressions, including calls to functions like int()
.
Here’s how to use an f-string to convert a binary string to an integer:
binary_string = "1101"
decimal_number = f"{int(binary_string, 2)}"
In this example, the int()
function is used to convert the binary string "1101"
to an integer with base 2 (binary). The result is then formatted using an f-string.
Once the conversion is performed within the f-string, you can display the result as needed.
Let’s go through an example to demonstrate how to convert a binary string to an integer using f-strings:
# Binary string
binary_string = "1101"
# Convert the binary string to an integer using an f-string
decimal_number = f"{int(binary_string, 2)}"
# Display the result
print(f"Binary String: {binary_string}")
print(f"Decimal Number: {decimal_number}")
When you run this code, you’ll see the following output:
Binary String: 1101
Decimal Number: 13
In this example, we start with the binary string "1101"
, which represents the decimal number 13
. Using an f-string, we convert the binary string to an integer and display the results.
It’s essential to ensure that the binary string contains only 0s and 1s. Any other characters would result in a ValueError
when converting to an integer using base 2.
Convert Binary to Integer in Python Using a Custom Function
Another way we can convert binary to an integer is by using a custom function in Python. This method is particularly useful when you want to have more control or understand the underlying process of the conversion.
To do this, we will create a Python function that iterates through the binary string and accumulates the decimal value based on the position and value of each binary digit.
Here’s how to create and use a custom function for binary to integer conversion:
First, define a custom function.
def binary_to_integer(binary_string):
decimal_integer = 0
for digit in binary_string:
decimal_integer = decimal_integer * 2 + int(digit)
return decimal_integer
In this function, binary_to_integer
, we initialize decimal_integer
to 0
. Then, we iterate through each digit in the binary string.
For each digit, we double the existing decimal_integer
and add the integer value of the binary digit (0
or 1
) to it. This process effectively simulates the conversion from binary to decimal.
Now that we have our custom function defined, we can use it to convert binary strings to integers.
binary_string = "1101"
decimal_integer = binary_to_integer(binary_string)
print(decimal_integer)
In this example, binary_string
is set to 1101
. After calling binary_to_integer(binary_string)
, the decimal_integer
variable will contain the integer equivalent of the binary input, which is 13
in this case.
Using a custom function for binary-to-integer conversion offers several advantages:
- With a custom function, you have full control over the conversion process. You can modify the function to handle different input formats or perform additional operations if needed.
- Creating a custom function allows you to gain a deeper understanding of how binary-to-integer conversion works. It’s a valuable learning experience.
- Once you’ve defined the custom function, you can reuse it in your code whenever you need to perform binary to integer conversions.
- You can extend the function to support variations of binary representations or customize it to suit your specific requirements.
Convert Binary to Integer in Python Using the bin()
Function
Python also provides another unique method for converting binary strings to integers, the built-in bin()
function.
Python’s bin()
function is primarily designed to convert an integer into a binary string. However, by reversing the process and applying it creatively, we can use it to convert binary strings to integers.
Let’s take a closer look at how to do this:
Begin by creating a binary string that you want to convert to an integer. For this example, we’ll use the binary string 1101
.
To convert the binary string to an integer, you can apply the int()
function to the result of bin()
:
binary_string = "1101"
decimal_integer = int(bin(int(binary_string, 2)), 2)
print(decimal_integer)
In this code, int(binary_string, 2)
first converts the binary string to an integer. The bin()
function is then applied to this integer, which converts it back to a binary string but with a 0b
prefix.
Finally, int(..., 2)
is used again to convert this binary string (with the 0b
prefix) back to an integer. The result is stored in the decimal_integer
variable.
After executing the code, the decimal_integer
variable will contain the decimal (base-10) representation of the original binary string. In this case, decimal_integer
will be 13
, as 1101
in binary is equivalent to 13
in decimal.
Using the bin()
function to convert binary strings to integers offers several advantages:
- This method showcases a unique approach to binary-to-integer conversion, which can be useful in specific scenarios where the
bin()
function is already part of your code. - The use of
bin()
makes your code more readable by explicitly indicating the conversion process from binary to integer. - You don’t need to write custom functions or complex logic; the
bin()
function provides a built-in and efficient way to achieve the conversion. - If you’re already familiar with using the
bin()
function for integer-to-binary conversion, this method leverages the same syntax in reverse.
Convert Binary to Integer in Python Using List Comprehensions and Enumerate
Another creative approach to convert binary to integer in Python is to use list comprehensions and the enumerate
function.
List comprehensions allows you to create lists using a concise and readable syntax. By combining list comprehensions with the enumerate
function, we can efficiently convert binary strings to integers.
Start by defining a binary string that you want to convert to an integer. For this example, we’ll still use the binary string 1101
.
To convert the binary string to an integer, you can use list comprehensions along with the enumerate
function. Here’s the code:
binary_string = "1101"
decimal_integer = sum(
int(bit) * 2**i for i, bit in enumerate(reversed(binary_string))
)
print(decimal_integer)
In this code, enumerate(reversed(binary_string))
is used to iterate through the binary string in reverse order while keeping track of the position of each bit. The bit
variable represents each binary digit, and i
represents its position (from right to left).
We multiply each bit by 2
to the power of its position and sum them up, which effectively converts the binary string to an integer.
After executing the code, the decimal_integer
variable will store the decimal (base-10) representation of the binary input. In this case, decimal_integer
will be 13
, as 1101
in binary is equivalent to 13
in decimal.
Utilizing list comprehensions and the enumerate
function for binary-to-integer conversion provides several advantages:
- The code is highly readable and concise, making it easy to understand and maintain.
- This method can be easily adapted to handle various binary string representations, making it versatile for different use cases.
- It follows Python’s idiomatic style, making your code more in line with the principles of the language.
- You don’t need to create custom functions for the conversion, as list comprehensions and
enumerate
provide a built-in solution.
Convert Binary to Integer in Python Using Bitwise Left Shift Operations
One of the less conventional but highly efficient approaches for binary-to-integer conversion involves using bitwise left-shift operations.
Bitwise left shift operations are fundamental operations in computer programming. The left shift operator (<<
) moves the bits of a binary number to the left by a specified number of positions.
This effectively multiplies the number by 2
raised to the power of the shift value. Using this concept, we can convert binary strings to integers by iterating through the binary digits and applying left shifts.
Here’s how to convert a binary string to an integer using bitwise left-shift operations:
Begin by defining the binary string that you want to convert to an integer. For our example, we’ll use the same binary string 1101
.
You’ll need to initialize a variable to store the decimal integer. Let’s call it decimal_integer
and set it to 0
initially.
Iterate through the binary string and apply left shifts as needed to accumulate the decimal integer value. Here’s the code to do this:
binary_string = "1101"
decimal_integer = 0
for bit in binary_string:
# Left shift the current value of decimal_integer by one bit and add the new bit
decimal_integer = (decimal_integer << 1) | int(bit)
print(decimal_integer)
Output:
13
In the code above, for each bit in the binary string, we left shift the current value of decimal_integer
by one bit using the <<
operator. We then use the bitwise OR (|
) operation to add the new bit to the integer.
After running the code, the decimal_integer
variable will contain the decimal (base-10) representation of the original binary string. In this case, decimal_integer
will be 13
, as 1101
in binary is equivalent to 13
in decimal.
Converting binary to an integer using bitwise left shift operations offers several advantages:
- Bitwise operations are highly efficient and can be faster than some other conversion methods, making this approach suitable for performance-critical applications.
- The code is concise and elegant, with the ability to convert binary strings to integers in a few lines of code.
- This method works with various binary string representations, and you can easily adapt it to different use cases.
- There’s no need to create custom functions; bitwise left shifts are part of the core Python language.
Conclusion
In this article, we’ve delved into several methods for converting binary representations to integers in Python. We began by exploring the 0b
prefix, a straightforward way to indicate binary numbers in Python.
We then examined alternative techniques, such as using the int()
function, f-strings, custom functions, the bin()
function, list comprehensions, and bitwise left shift operations. Each of these methods presents its unique advantages, from simplicity and readability to efficiency and control.
As a Python programmer, having a repertoire of these conversion methods at your disposal ensures flexibility and proficiency when working with binary data. Whether you choose to use the 0b
prefix for clarity or opt for custom functions to gain a deeper understanding, the choice ultimately depends on your specific needs and preferences.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Binary
- How to Convert Binary to Hex in Python
- Binary Numbers Representation in Python
- How to Convert a String to Binary in Python