Binary Numbers Representation in Python
- Summing Binary Numbers Using Built-in Functions
- Manipulating Binary Numbers with Bitwise Operators
- Conclusion
- FAQ
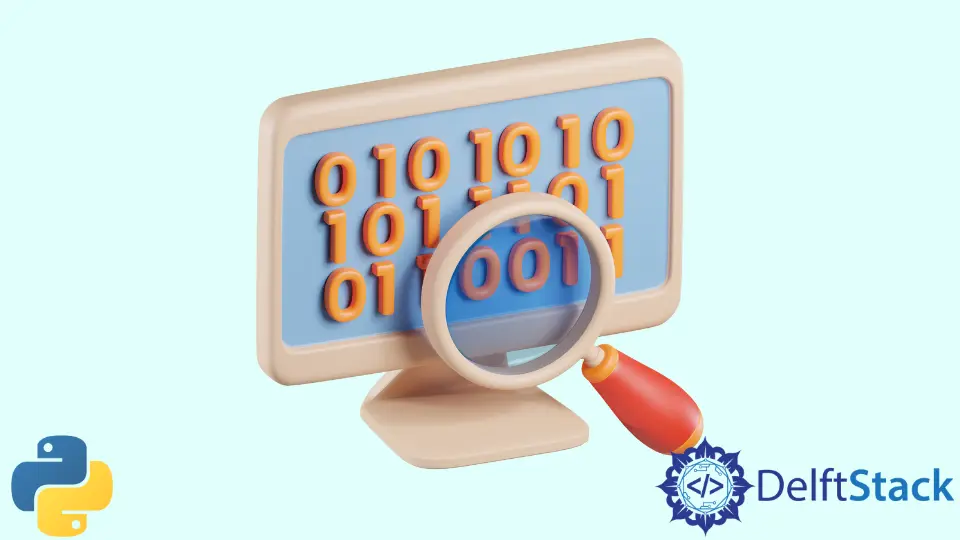
Understanding binary numbers is essential for anyone delving into programming, especially in Python.
This tutorial will guide you through summing binary numbers using Python’s built-in functions and manipulating binary data with bitwise operators. By the end of this article, you will have a solid grasp of how to represent binary numbers in Python and perform various operations on them. Whether you’re a beginner or looking to brush up on your skills, this comprehensive guide will enhance your coding toolkit. Let’s dive into the fascinating world of binary numbers and see how Python can make these operations straightforward and efficient.
Summing Binary Numbers Using Built-in Functions
Python offers a straightforward way to sum binary numbers by leveraging its built-in functions. We can convert binary strings into integers, perform the addition, and then convert the result back into a binary format. The built-in function bin()
is particularly useful for converting integers back to binary strings.
Here’s how you can do it:
binary1 = '1101' # Binary representation of 13
binary2 = '1011' # Binary representation of 11
sum_binary = bin(int(binary1, 2) + int(binary2, 2))[2:] # Sum and convert back to binary
print(sum_binary)
Output:
11000
In this code snippet, we start with two binary strings, binary1
and binary2
. By using int(binary1, 2)
, we convert the binary string to its integer equivalent. This is crucial because Python cannot perform arithmetic operations directly on strings. After summing the two integers, we use the bin()
function to convert the result back to binary. The [2:]
slice is used to remove the 0b
prefix that Python adds to indicate a binary number. The final output, 11000
, represents the sum of the two binary numbers, which is 24 in decimal.
Manipulating Binary Numbers with Bitwise Operators
Bitwise operators are powerful tools in Python that allow you to manipulate binary numbers at the bit level. These operators include AND (&
), OR (|
), XOR (^
), NOT (~
), left shift (<<
), and right shift (>>
). Using these operators, you can perform various operations on binary data efficiently.
Here’s an example of using bitwise operators:
a = 0b1101 # Binary representation of 13
b = 0b1011 # Binary representation of 11
and_result = a & b
or_result = a | b
xor_result = a ^ b
not_result = ~a
print(bin(and_result))
print(bin(or_result))
print(bin(xor_result))
print(bin(not_result))
Output:
0b1001
0b1111
0b0100
-0b1110
In this example, we define two binary numbers, a
and b
, using Python’s binary literal notation. The bitwise AND operation (&
) produces a result where only the bits that are set in both a
and b
remain set. The OR operation (|
) retains all bits that are set in either number. The XOR operation (^
) gives us a result where bits are set only if they are different between the two numbers. Finally, the NOT operation (~
) inverts all the bits of a
. The output shows the results of these operations in binary format, allowing you to see how each operation affects the bits.
Conclusion
In this article, we’ve explored the representation of binary numbers in Python and how to manipulate them using built-in functions and bitwise operators. Summing binary numbers is made easy with Python’s conversion functions, allowing seamless transitions between binary and decimal formats. Furthermore, the use of bitwise operators opens up a new realm of possibilities for data manipulation, making Python a versatile tool for programmers. Whether you’re working on a small project or a larger application, understanding these concepts will enhance your coding skills and enable you to tackle more complex tasks with confidence.
FAQ
-
What is a binary number?
A binary number is a representation of numeric values using two symbols: 0 and 1. It is the base-2 numeral system used in computer science. -
How do I convert a binary number to decimal in Python?
You can convert a binary number to decimal using theint()
function with base 2, like this:int('binary_string', 2)
. -
What are bitwise operators in Python?
Bitwise operators are used to perform operations on binary numbers at the bit level, including AND, OR, XOR, NOT, left shift, and right shift. -
Can I perform arithmetic operations directly on binary strings in Python?
No, you must first convert binary strings to integers before performing arithmetic operations. -
How does the
bin()
function work in Python?
Thebin()
function converts an integer into its binary representation as a string, prefixed with ‘0b’ to indicate that it is a binary number.
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedIn