在 Python 中將二進位制轉換為十六進位制
- 在 Python 中建立並使用使用者定義的函式將二進位制轉換為十六進位制
-
在 Python 中使用
int()
和hex()
函式將Binary
轉換為Hex
-
在 Python 中使用
binascii
模組將Binary
轉換為Hex
-
在 Python 中使用
format()
函式將Binary
轉換為Hex
-
在 Python 中使用
f-strings
將Binary
轉換為Hex
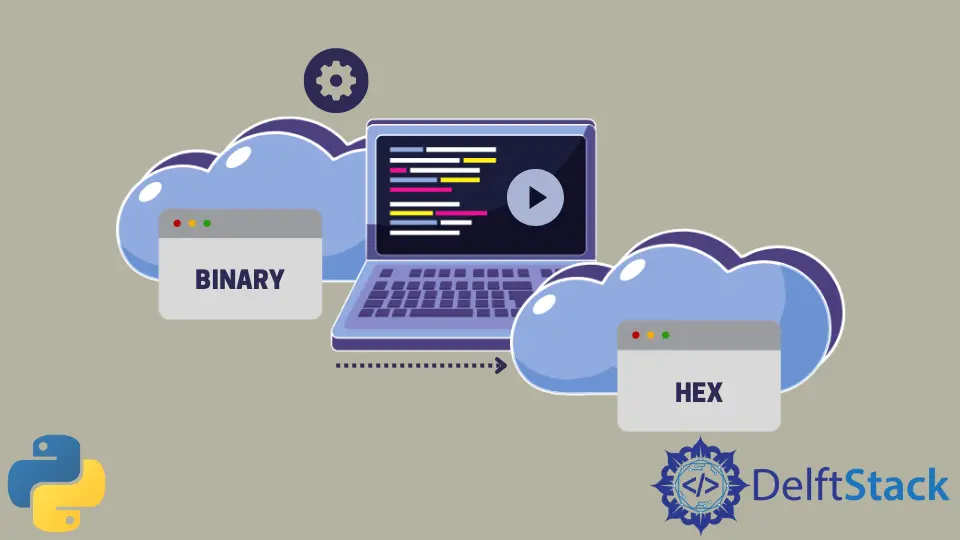
二進位制和十六進位制是可以在 Python 中表示數值的眾多數字系統中的兩個。本教程重點介紹在 Python 中將 Binary
轉換為 Hex
的不同方法。
在 Python 中建立並使用使用者定義的函式將二進位制轉換為十六進位制
我們可以在 while
迴圈的幫助下建立我們的使用者定義函式,並將其放置到位以將 Binary
中的值轉換為 Python 中的 Hex
。
以下程式碼使用使用者定義的函式在 Python 中將 Binary
轉換為 Hex
。
print("Enter the Binary Number: ", end="")
bnum = int(input())
h = 0
m = 1
chk = 1
i = 0
hnum = []
while bnum != 0:
rem = bnum % 10
h = h + (rem * m)
if chk % 4 == 0:
if h < 10:
hnum.insert(i, chr(h + 48))
else:
hnum.insert(i, chr(h + 55))
m = 1
h = 0
chk = 1
i = i + 1
else:
m = m * 2
chk = chk + 1
bnum = int(bnum / 10)
if chk != 1:
hnum.insert(i, chr(h + 48))
if chk == 1:
i = i - 1
print("\nEquivalent Hexadecimal Value = ", end="")
while i >= 0:
print(end=hnum[i])
i = i - 1
print()
上面的程式碼提供了以下輸出。
Enter the Binary Number: 0101101
Equivalent Hexadecimal Value = 2D
在 Python 中使用 int()
和 hex()
函式將 Binary
轉換為 Hex
我們同時使用 int()
和 hex()
函式來實現此方法。
首先,使用 int()
方法將給定的二進位制數轉換為整數值。在此過程之後,hex()
函式將新找到的整數值轉換為十六進位制值。
以下程式碼使用 int()
和 hex()
函式在 Python 中將 Binary
轉換為 Hex
。
print(hex(int("0101101", 2)))
上面的程式碼提供了以下輸出。
0x2d
在 Python 中使用 binascii
模組將 Binary
轉換為 Hex
Python 提供了一個從 Python 3 開始的 binascii
模組,可用於在 Python 中將 Binary
轉換為 Hex
。binascii
模組需要手動匯入 Python 程式碼才能使此方法工作。
此方法開啟一個文字檔案,接收檔案的內容,並可以使用 hexlify()
函式返回檔案中給定資料的 hex
值。
以下程式碼使用 binascii
模組在 Python 中將 Binary
轉換為 Hex
。
import binascii
bFile = open("ANYBINFILE.exe", "rb")
bData = bFile.read(8)
print(binascii.hexlify(bData))
在 Python 中使用 format()
函式將 Binary
轉換為 Hex
format()
函式是在 Python 中實現字串格式化的方法之一。format()
函式用於在 {}
大括號內提供格式化字串。
以下程式碼使用 format()
函式在 Python 中將 Binary
轉換為 Hex
。
print("{0:0>4X}".format(int("0101101", 2)))
上面的程式碼提供了以下輸出。
002D
在 Python 中使用 f-strings
將 Binary
轉換為 Hex
在 Python 3.6 中引入,它是 Python 中實現字串格式化的最新方法。它可以在較新和最新版本的 Python 中使用。
它比其他兩個同行,%
符號和 str.format()
更有效,因為它更快更容易理解。它還有助於以比其他兩種方法更快的速度在 Python 中實現字串格式。
以下程式碼使用 f-strings
在 Python 中將 Binary
轉換為 Hex
。
bstr = "0101101"
hexstr = f"{int(bstr, 2):X}"
print(hexstr)
上面的程式碼提供了以下輸出。
2D
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn