在 Python 中將字串轉換為二進位制
Azaz Farooq
2023年1月30日
Python
Python String
Python Binary
-
在 Python 中使用
format()
函式將字串轉換為二進位制表示 -
在 Python 中使用
bytearray
方法將字串轉換為二進位制表示 -
在 Python 中使用
map()
函式將一個字串轉換為二進位制表示 -
在 Python 中使用
ASCII
方法將字串轉換為二進位制表示
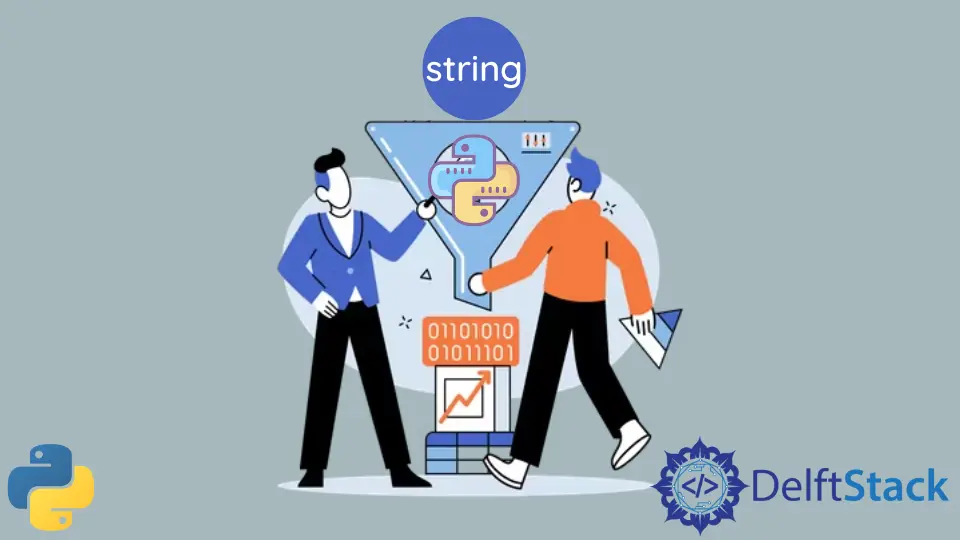
當字串轉換為二進位制時,它將生成代表原始字元的二進位制值列表。每個字元都必須迭代並轉換為二進位制。
本文將討論一些在 Python 中將字串轉換為其二進位制表示的方法。
在 Python 中使用 format()
函式將字串轉換為二進位制表示
我們使用 ord()
函式將字串的 Unicode 點轉換為相應的整數。format()
函式使用 b
二進位制格式將整數轉換為以 2 為底的數字。
完整的示例程式碼如下。
string = "Python"
binary_converted = " ".join(format(ord(c), "b") for c in string)
print("The Binary Representation is:", binary_converted)
輸出:
The Binary Represntation is: 1010000 1111001 1110100 1101000 1101111 1101110
在 Python 中使用 bytearray
方法將字串轉換為二進位制表示
位元組陣列是一組位元組,可以儲存二進位制資料列表。除了可以顯式遍歷字串之外,我們還可以遍歷位元組序列。可以不使用 ord()
函式,而是使用 bytearray()
函式來實現。
完整的示例程式碼如下。
string = "Python"
binary_converted = " ".join(format(c, "b") for c in bytearray(string, "utf-8"))
print("The Binary Represntation is:", binary_converted)
輸出:
The Binary Representation is: 1010000 1111001 1110100 1101000 1101111 1101110
在 Python 中使用 map()
函式將一個字串轉換為二進位制表示
我們也可以使用 map()
函式來代替 format()
函式。map()
使用 bytearray()
函式將字串轉換為位元組陣列,然後使用 bin
將位元組陣列轉換為二進位制表示。
完整的示例程式碼如下。
string = "Python"
binary_converted = " ".join(map(bin, bytearray(string, "utf-8")))
print("The Binary Represntation is:", binary_converted)
在 Python 3 中,我們必須定義一個類似於 utf-8
的編碼方案。否則,將引發錯誤。
輸出:
The Binary Represntation is: 0b1010000 0b1111001 0b1110100 0b1101000 0b1101111 0b1101110
在 Python 中使用 ASCII
方法將字串轉換為二進位制表示
在 Python 3 中,utf-8
是預設的編碼方案。但是這種方法將使用 ASCII
編碼方案,而不是 utf-8
。對於基本的字母數字字串,UTF-8
和 ASCII
編碼之間的差異並不明顯。但是,如果我們處理的文字包含的字元不屬於 ASCII
字符集,則它們將變得很重要。
完整的示例程式碼如下:
st = "Python"
a_bytes = bytes(st, "ascii")
binary_converted = " ".join(["{0:b}".format(x) for x in a_bytes])
print("The Binary Represntation is:", binary_converted)
輸出:
The Binary Representation is: 1010000 1111001 1110100 1101000 1101111 1101110
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
相關文章 - Python String
- 在 Python 中從字串中刪除逗號
- 如何以 Pythonic 方式檢查字符串是否為空
- 在 Python 中將字串轉換為變數名
- Python 如何去掉字串中的空格/空白符
- 如何在 Python 中從字串中提取數字
- Python 如何將字串轉換為時間日期 datetime 格式