在 Python 中将字符串转换为二进制
Azaz Farooq
2023年1月30日
-
在 Python 中使用
format()
函数将字符串转换为二进制表示 -
在 Python 中使用
bytearray
方法将字符串转换为二进制表示 -
在 Python 中使用
map()
函数将一个字符串转换为二进制表示 -
在 Python 中使用
ASCII
方法将字符串转换为二进制表示
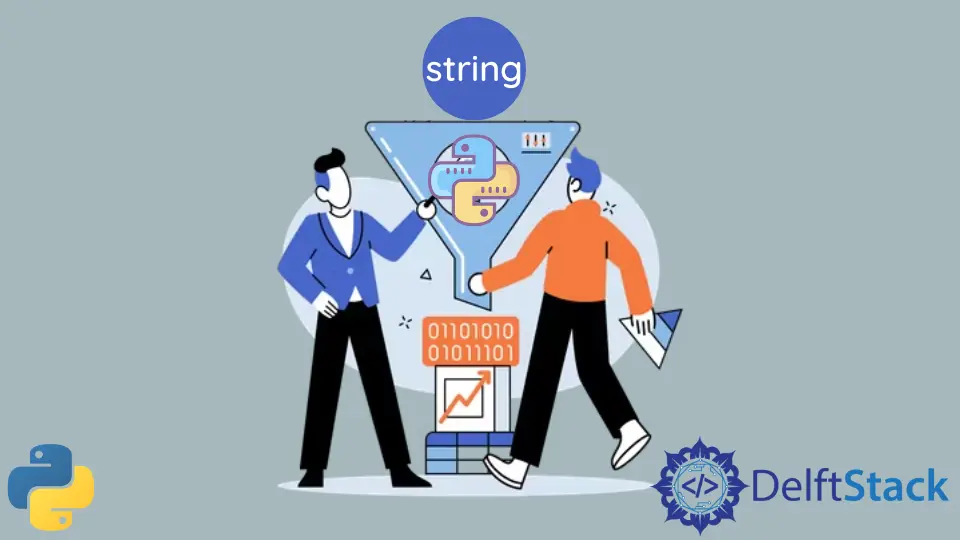
当字符串转换为二进制时,它将生成代表原始字符的二进制值列表。每个字符都必须迭代并转换为二进制。
本文将讨论一些在 Python 中将字符串转换为其二进制表示的方法。
在 Python 中使用 format()
函数将字符串转换为二进制表示
我们使用 ord()
函数将字符串的 Unicode 点转换为相应的整数。format()
函数使用 b
二进制格式将整数转换为以 2 为底的数字。
完整的示例代码如下。
string = "Python"
binary_converted = " ".join(format(ord(c), "b") for c in string)
print("The Binary Representation is:", binary_converted)
输出:
The Binary Represntation is: 1010000 1111001 1110100 1101000 1101111 1101110
在 Python 中使用 bytearray
方法将字符串转换为二进制表示
字节数组是一组字节,可以存储二进制数据列表。除了可以显式遍历字符串之外,我们还可以遍历字节序列。可以不使用 ord()
函数,而是使用 bytearray()
函数来实现。
完整的示例代码如下。
string = "Python"
binary_converted = " ".join(format(c, "b") for c in bytearray(string, "utf-8"))
print("The Binary Represntation is:", binary_converted)
输出:
The Binary Representation is: 1010000 1111001 1110100 1101000 1101111 1101110
在 Python 中使用 map()
函数将一个字符串转换为二进制表示
我们也可以使用 map()
函数来代替 format()
函数。map()
使用 bytearray()
函数将字符串转换为字节数组,然后使用 bin
将字节数组转换为二进制表示。
完整的示例代码如下。
string = "Python"
binary_converted = " ".join(map(bin, bytearray(string, "utf-8")))
print("The Binary Represntation is:", binary_converted)
在 Python 3 中,我们必须定义一个类似于 utf-8
的编码方案。否则,将引发错误。
输出:
The Binary Represntation is: 0b1010000 0b1111001 0b1110100 0b1101000 0b1101111 0b1101110
在 Python 中使用 ASCII
方法将字符串转换为二进制表示
在 Python 3 中,utf-8
是默认的编码方案。但是这种方法将使用 ASCII
编码方案,而不是 utf-8
。对于基本的字母数字字符串,UTF-8
和 ASCII
编码之间的差异并不明显。但是,如果我们处理的文本包含的字符不属于 ASCII
字符集,则它们将变得很重要。
完整的示例代码如下:
st = "Python"
a_bytes = bytes(st, "ascii")
binary_converted = " ".join(["{0:b}".format(x) for x in a_bytes])
print("The Binary Represntation is:", binary_converted)
输出:
The Binary Representation is: 1010000 1111001 1110100 1101000 1101111 1101110
相关文章 - Python String
- 在 Python 中从字符串中删除逗号
- 在 Python 中将字符串转换为变量名
- Python 如何去掉字符串中的空格/空白符
- 如何在 Python 中从字符串中提取数字
- Python 如何将字符串转换为时间日期 datetime 格式
- Python2 和 3 中如何将(Unicode)字符串转换为小写