Python で文字列をバイナリに変換する
-
Python で
format()
関数を使用して文字列をバイナリ表現に変換する -
Python で
bytearray
メソッドを使用して文字列をバイナリ表現に変換する -
Python で
map()
関数を使用して文字列をバイナリ表現に変換する -
Python で
ASCII
メソッドを使用して文字列をバイナリ表現に変換する
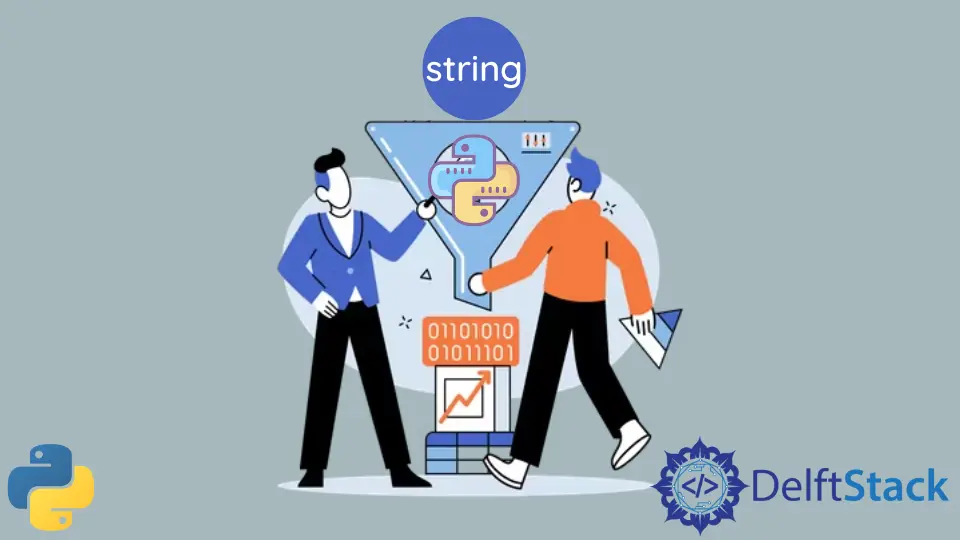
文字列がバイナリに変換されると、元の文字を表すバイナリ値のリストが生成されます。各文字を繰り返し処理して、バイナリに変換する必要があります。
この記事では、Python で文字列をバイナリ表現に変換するいくつかの方法について説明します。
Python で format()
関数を使用して文字列をバイナリ表現に変換する
文字列の Unicode ポイントを対応する整数に変換する ord()
関数を使用します。format()
関数は、b
バイナリ形式を使用して整数を基数 2 の数値に変換します。
完全なサンプルコードを以下に示します。
string = "Python"
binary_converted = " ".join(format(ord(c), "b") for c in string)
print("The Binary Representation is:", binary_converted)
出力:
The Binary Represntation is: 1010000 1111001 1110100 1101000 1101111 1101110
Python で bytearray
メソッドを使用して文字列をバイナリ表現に変換する
バイト配列は、バイナリデータのリストを格納できるバイトのセットです。文字列を明示的に反復する代わりに、バイトシーケンスを反復することができます。これは、ord()
関数を使用せずに、bytearray()
関数を使用して実現できます。
完全なサンプルコードを以下に示します。
string = "Python"
binary_converted = " ".join(format(c, "b") for c in bytearray(string, "utf-8"))
print("The Binary Represntation is:", binary_converted)
出力:
The Binary Representation is: 1010000 1111001 1110100 1101000 1101111 1101110
Python で map()
関数を使用して文字列をバイナリ表現に変換する
format()
関数の代わりに map()
関数を使用することもできます。map()
で文字列を bytearray()
関数でバイト配列に変換し、bin
でバイト配列をバイナリ表現に変換する。
完全なサンプルコードを以下に示します。
string = "Python"
binary_converted = " ".join(map(bin, bytearray(string, "utf-8")))
print("The Binary Represntation is:", binary_converted)
Python 3 では、utf-8
のようなエンコードスキームを定義する必要があります。そうしないと、エラーが発生します。
出力:
The Binary Represntation is: 0b1010000 0b1111001 0b1110100 0b1101000 0b1101111 0b1101110
Python で ASCII
メソッドを使用して文字列をバイナリ表現に変換する
Python 3 では、utf-8
がデフォルトのエンコード方式です。ただし、このメソッドは、utf-8
の代わりに ASCII
エンコーディングスキームを使用します。基本的な英数字の文字列の場合、UTF-8
と ASCII
エンコーディングの違いは目立ちません。ただし、ASCII
文字コレクションの一部ではない文字を組み込んだテキストを処理している場合、これらは重要になります。
完全なサンプルコードを以下に示します。
st = "Python"
a_bytes = bytes(st, "ascii")
binary_converted = " ".join(["{0:b}".format(x) for x in a_bytes])
print("The Binary Represntation is:", binary_converted)
出力:
The Binary Representation is: 1010000 1111001 1110100 1101000 1101111 1101110
関連記事 - Python String
- Python で文字列からコンマを削除する
- Pythonic な方法で文字列が空かどうかを確認する方法
- Python で文字列を変数名に変換する
- Python 文字列の空白を削除する方法
- Python で文字列から数字を抽出する
- Python が文字列を日時 datetime に変換する方法