Python で文字列から数字を抽出する
Syed Moiz Haider
2021年7月20日
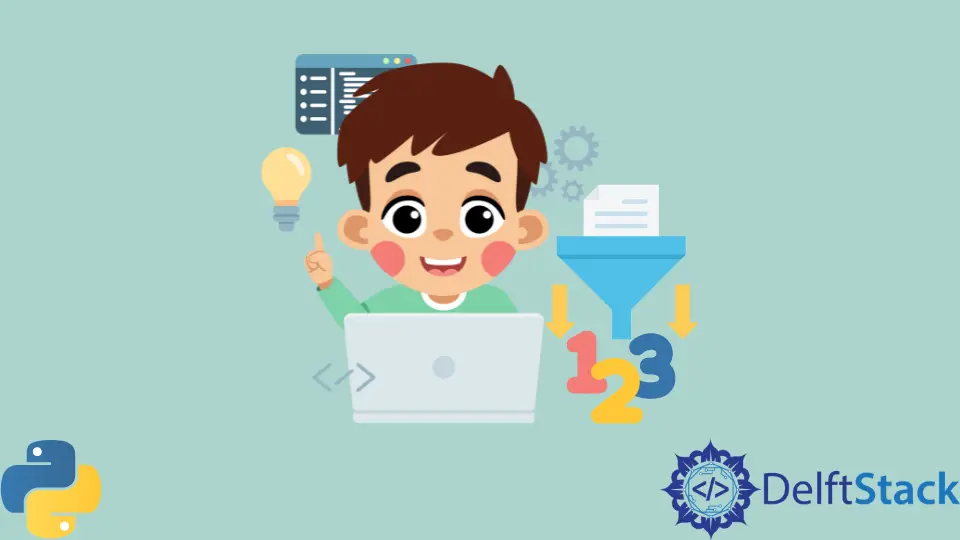
このチュートリアルでは、Python で文字列から数値を取得する方法を説明します。また、異なるアプローチを使用して概念をさらに明確にするために、いくつかのコード例を挙げています。
文字列から数字を抽出するためにリスト内包表記を使用する
文字列から数字を得るには、単純なリスト内包を用います。文字列を文字列のリストに変換するには split()
メソッドを、桁が見つかったかどうかを調べるには isdigit()
メソッドを用います。
基本コードの例は次のとおりです。
temp_string = "Hi my age is 32 years and 250 days12"
print(temp_string)
numbers = [int(temp) for temp in temp_string.split() if temp.isdigit()]
print(numbers)
出力:
Hi my age is 32 years and 250 days12
[32, 250]
しかし、このコードではアルファベットを含む数字は識別できません。
文字列から数値を抽出するには re
モジュールを用いる
Python の re
モジュールには、文字列を検索して結果を抽出する関数も用意されています。re
モジュールは findall()
メソッドを提供しており、これはすべてのマッチしたリストを返します。以下にコード例を示します。
import re
temp_string = "Hi my age is 32 years and 250.5 days12"
print(temp_string)
print([float(s) for s in re.findall(r"-?\d+\.?\d*", temp_string)])
出力:
Hi my age is 32 years and 250.5 days12
[32.0, 250.5, 12.0]
RegEx
の解は負と正の両方の数値に対して動作し、リスト内包表記のアプローチで発生した問題を克服します。
著者: Syed Moiz Haider
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn