How to Convert HEX to RGB in Python
-
Convert a Hexadecimal Value to an RGB Value With the Python Image Library
PIL
in Python - Convert a Hexadecimal Value to an RGB Value With the Self-Defined Method in Python
- Converting Hexadecimal to RGB With the Regular Expressions in Python
-
Converting Hexadecimal to RGB With the
bytes.fromhex
and Unpacking in Python -
Converting Hexadecimal to RGB With the
Matplotlib
and Unpacking in Python -
Converting Hexadecimal to RGB With the
struct.unpack
in Python - Conclusion
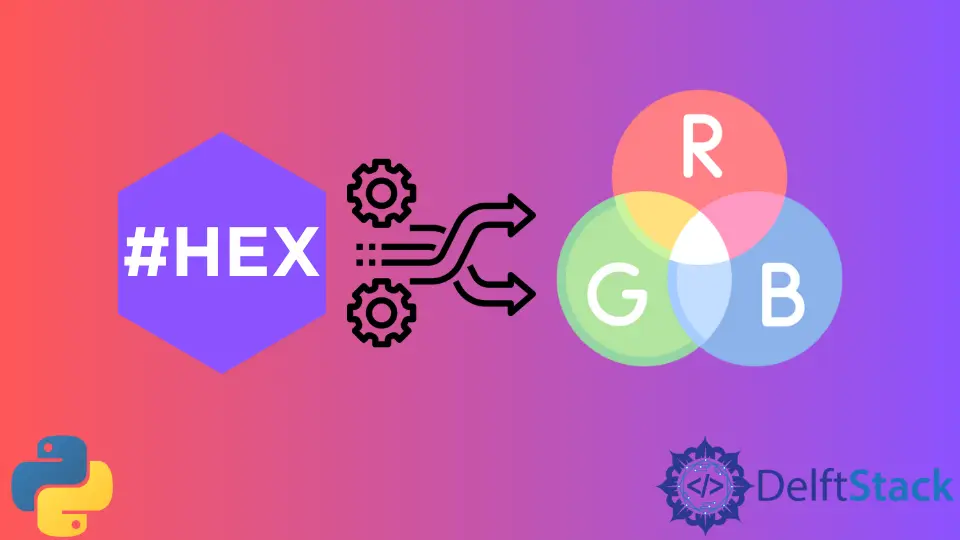
In the realm of digital graphics and web design, the translation between hexadecimal color codes and RGB (Red, Green, Blue) values is a fundamental task. This conversion is crucial because hexadecimal codes are extensively used in web design and graphic tools, while RGB values are essential for various operations in image processing and digital graphics.
Python, known for its simplicity and vast libraries, offers multiple methods to perform this conversion, each suited to different contexts and requirements. This article delves into several Python techniques for converting hexadecimal values to RGB, covering methods that range from using built-in libraries like the Python Image Library (PIL) and Matplotlib to implementing custom solutions.
These methods are not only practical for typical web and graphic tasks but also provide insights into Python’s versatility in handling different data types and formats.
Convert a Hexadecimal Value to an RGB Value With the Python Image Library PIL
in Python
The PIL
library or Python Image Library provides many tools for working with images in Python. If we have a Hexadecimal value and we want to convert it to a corresponding RGB value, we can use the PIL
library for that.
The ImageColor.getcolor()
function in the PIL
library takes a color string and converts it to a corresponding RGB value. The following example program demonstrates how we can convert a Hexadecimal value to an RGB value with the PIL
library.
from PIL import ImageColor
def hex_to_rgb(hex_color):
return ImageColor.getcolor(hex_color, "RGB")
# Example usage
print(hex_to_rgb("#FF5733"))
In this example, we begin by importing the necessary module ImageColor
from the Pillow
package. The hex_to_rgb
function is defined to take a single parameter, hex_color
, a string representing a hex color code.
Within the function, the ImageColor.getcolor()
method is employed, utilizing the provided hex color code and specifying the desired color mode as RGB
. The result is a tuple representing the color in RGB format.
In the example usage, we call the hex_to_rgb
function with the hex color code #FF5733
and print the resulting RGB tuple.
Output:
Convert a Hexadecimal Value to an RGB Value With the Self-Defined Method in Python
We will manually convert the user input from a Hexadecimal format to an RGB value in this method. First, we can remove the #
character from the user input and convert the hexadecimal values to base-10 integer values with the int()
function for each alternating index.
After that, we can group these converted values into an RGB tuple with the tuple()
function. The example program below shows how we can convert a Hexadecimal value to an RGB value with the self-defined approach.
def hex_to_rgb(hex_color):
hex_color = hex_color.lstrip("#") # Remove the '#' prefix if present
return tuple(int(hex_color[i : i + 2], 16) for i in (0, 2, 4))
# Example usage
print(hex_to_rgb("#FF5733"))
In our hex_to_rgb
function, we begin by ensuring compatibility with standard hex color formats. This is achieved by removing the #
prefix from the hex color string using the lstrip()
method.
The core of our function is the line tuple(int(hex_color[i:i+2], 16) for i in (0, 2, 4))
, where we utilize Python’s generator expression within a tuple constructor for a concise and efficient conversion process.
We iterate over the indices 0, 2, and 4, which correspond to the starting positions of the red, green, and blue components in the hex string, respectively. For each of these components, we extract a substring of two characters using hex_color[i:i+2]
.
This substring, representing a color component in hexadecimal, is then converted into an integer. The int()
function is instrumental here, as we specify 16 as the base, allowing the conversion from a hexadecimal to a decimal number.
Output:
Converting Hexadecimal to RGB With the Regular Expressions in Python
Regular expressions (regex) are powerful tools for pattern matching in strings. In Python, the re
module facilitates regex operations, allowing us to validate and dissect strings according to defined patterns.
When converting hex to RGB, regex can parse and extract individual color components accurately.
import re
def hex_to_rgb(hex_color):
hex_color = hex_color.lstrip("#") # Remove the '#' if present
return tuple(int(n, 16) for n in re.findall(r"..", hex_color))
# Example Usage
print(hex_to_rgb("#FF5733")) # Expected Output: (255, 87, 51)
In the hex_to_rgb
function, we first remove any #
from the start of the hex string for standardization. The re.findall(r'..', hex_color)
is crucial, as it finds all occurrences of two consecutive characters in the hex string.
These two-character groups represent the red, green, and blue components of the color in hexadecimal format.
By iterating over these groups with tuple(int(n, 16) for n in ...)
, we convert each hexadecimal value to its decimal equivalent using int(n, 16)
.
This conversion is pivotal as it transforms the hex values into the RGB format. The function then returns these values as a tuple, effectively converting the color from hex to RGB.
Output:
Converting Hexadecimal to RGB With the bytes.fromhex
and Unpacking in Python
Python provides an efficient way to convert hex color codes to RGB tuples using bytes.fromhex
and tuple unpacking. The bytes.fromhex
method is designed to convert hex strings into bytes, which are essentially sequences of integers.
Tuple unpacking then allows us to extract these integers into a format suitable for representing RGB values.
def hex_to_rgb(hex_color):
hex_color = hex_color.lstrip("#") # Remove '#' if present
return tuple(bytes.fromhex(hex_color))
# Example Usage
print(hex_to_rgb("#FF5733")) # Output expected: (255, 87, 51)
In our hex_to_rgb
function, we start by removing the #
symbol from the hex string. This standardizes the input format, making the function versatile for different hex string formats.
The core of the function involves the bytes.fromhex(hex_color)
method. This method takes the hex string (now without the #
) and converts it into a bytes object.
Each pair of hex digits is converted into its corresponding byte, which in turn is a number between 0 and 255 – exactly what we need for RGB values.
After converting the hex string to a bytes object, we directly unpack this object into a tuple. This step is facilitated by Python’s ability to unpack sequences into multiple variables.
In our case, since an RGB color is represented by three components, the bytes object (comprising three bytes) is unpacked into a tuple of three integers.
Output:
Converting Hexadecimal to RGB With the Matplotlib
and Unpacking in Python
Matplotlib, a comprehensive library primarily used for creating static, animated, and interactive visualizations in Python, also offers convenient functions for color manipulation, including the conversion of hex color codes to RGB values.
The Matplotlib library’s utility extends beyond plotting and charting. It provides a suite of color-related functions, among which is the ability to convert hexadecimal color codes to RGB format. This functionality is particularly valuable as it allows users to leverage an established, well-documented, and reliable tool for color conversion tasks.
import matplotlib.colors as mcolors
def hex_to_rgb(hex_color):
# Converts hex to a normalized RGB tuple, then to standard RGB format
return tuple(round(i * 255) for i in mcolors.hex2color(hex_color))
# Example Usage
print(hex_to_rgb("#FF5733")) # Output: (255, 87, 51)
In our approach to converting hexadecimal to RGB using the Matplotlib library, we first import the matplotlib.colors
module as mcolors
, recognizing its capabilities not just in plotting but also in color manipulation.
We define the hex_to_rgb
function, tasked with the conversion process, taking a hex color code as its input. The core of our function is the mcolors.hex2color
call, adept at transforming a hexadecimal string to a normalized RGB tuple (with values between 0 and 1).
To align these values with the standard RGB format, where components are integers from 0 to 255, we multiply each by 255 and round to the nearest integer. This is efficiently done using a generator expression within the tuple
function, which iterates over each component, performs the multiplication and rounding, and returns the results in a tuple format.
Output:
Converting Hexadecimal to RGB With the struct.unpack
in Python
The struct
module in Python offers a way to work with packed binary data. In our case, it’s a powerful tool to convert a hexadecimal color code into an RGB tuple.
This method is particularly useful when dealing with low-level data manipulation or when performance is a key concern.
format
: A string that determines how the data is to be interpreted. For RGB conversion, we use 'BBB'
which stands for three unsigned bytes - the components of the RGB.
buffer
: The binary data to be unpacked. In our case, it’s the hexadecimal color string converted to bytes.
Example.
import struct
def hex_to_rgb(hex_color):
hex_color = hex_color.lstrip("#")
return struct.unpack("BBB", bytes.fromhex(hex_color))
# Example usage
print(hex_to_rgb("#FF5733"))
In this code snippet, we start by importing the struct
module.
We then define the function hex_to_rgb
, which takes a hexadecimal color string as input. Inside the function, we first remove the #
character from the start of the string (if present) to get the pure hexadecimal value.
Next, we convert the hexadecimal string into bytes using bytes.fromhex(hex_color)
. This conversion is essential because struct.unpack
works with binary data.
Finally, we unpack these bytes using the format 'BBB'
, which tells Python to interpret the three bytes as three separate values, each corresponding to a color component in RGB.
Output:
Conclusion
This comprehensive exploration into converting hexadecimal values to RGB in Python has illuminated the versatility and power of Python in handling such conversions. From utilizing high-level libraries like PIL
and Matplotlib
to delving into more intricate methods involving regular expressions
and the struct
module, Python offers a rich set of tools for this common yet crucial task in digital graphics and web design.
Each method discussed has its unique advantages, catering to different needs and scenarios, whether it’s the simplicity and readability of using high-level libraries or the efficiency and control provided by lower-level operations.
Understanding these different approaches is invaluable for Python programmers working in fields related to web development, data visualization, and digital art. It empowers them to choose the most appropriate method based on the context of their project, efficiency requirements, and their comfort with Python programming.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn